Java Reference
In-Depth Information
If we wanted the code to return the first of the two values in the middle instead,
we could subtract 1 from the length before dividing it in half. Here is a complete set
of
println
statements that follows this approach:
System.out.println("first = " + list[0]);
System.out.println("middle = " + list[(list.length - 1) / 2]);
System.out.println("last = " + list[list.length - 1]);
As you learn how to use arrays, you will find yourself wondering what types of
operations you can perform on an array element that you are accessing. For example,
for the array of integers called
list
, what exactly can you do with
list[i]
? The
answer is that you can do anything with
list[i]
that you would normally do with
any variable of type
int
. For example, if you have a variable called
x
of type
int
,any
of the following expressions are valid:
x = 3;
x++;
x *= 2;
x--;
That means that the same expressions are valid for
list[i]
if
list
is an array of
integers:
list[i] = 3;
list[i]++;
list[i] *= 2;
list[i]--;
From Java's point of view, because
list
is declared to be of type
int[]
, an array
element like
list[i]
is of type
int
and can be manipulated as such. For example, to
increment every value in the array, you could use the standard traversing loop:
for (int i = 0; i < list.length; i++) {
list[i]++;
}
This code would increment each value in the array, turning the array of odd num-
bers into an array of even numbers.
It is possible to refer to an illegal index of an array, in which case Java throws an
exception. For example, for an array of length 5, the legal indexes are from 0 to 4.
Any number less than 0 or greater than 4 is outside the bounds of the array:
[0]
[1]
[2]
[3]
[4]
1
3
5
7
9
index less than 0
out of bounds
legal indexes 0-
4
index 5 or more
out of bounds




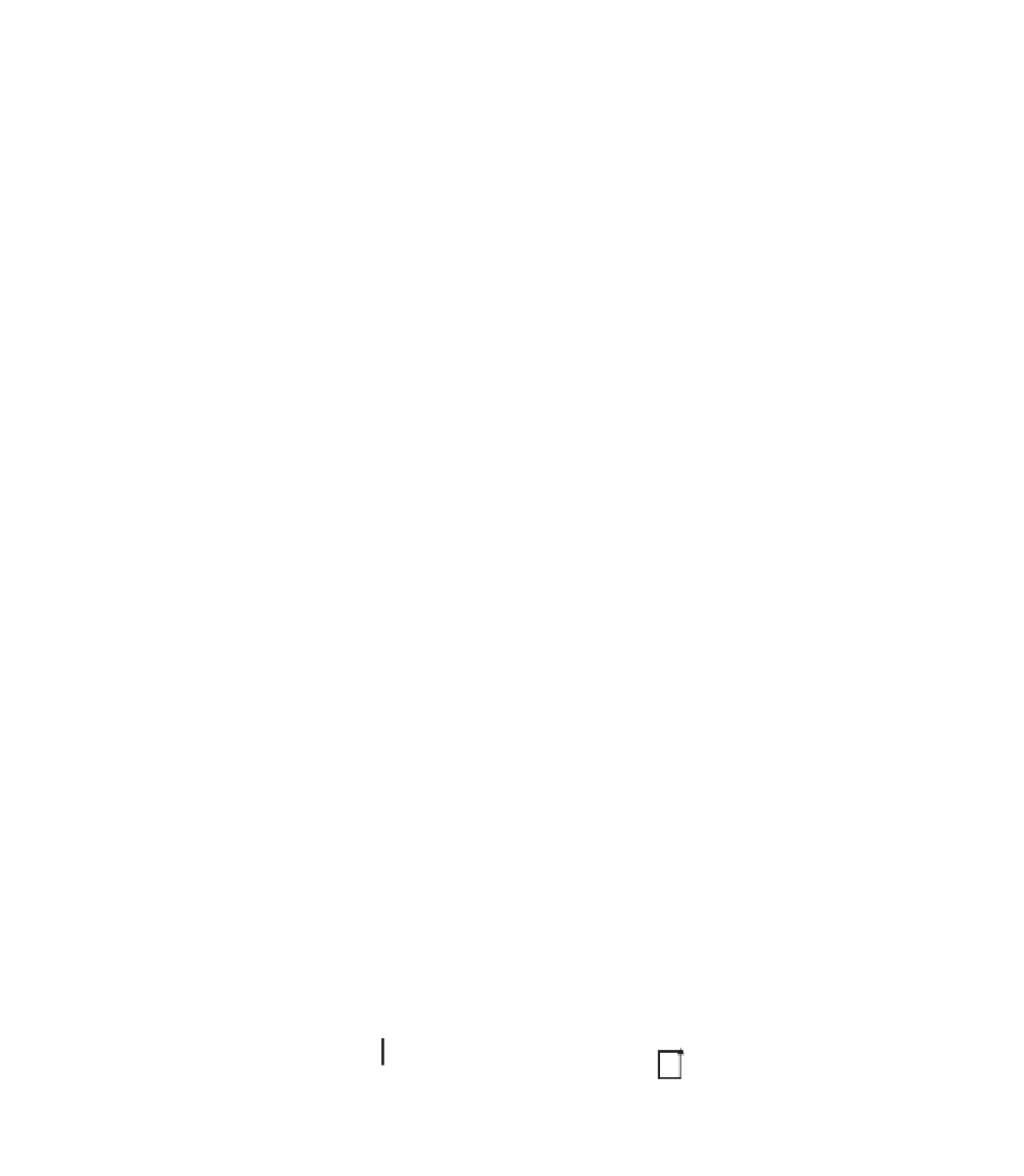
Search WWH ::

Custom Search