Java Reference
In-Depth Information
include the parentheses after the word “length.” This is another one of those unfortu-
nate inconsistencies that Java programmers just have to memorize.
The previous code provides a pattern that you will see often with array-processing
code: a
for
loop that starts at 0 and that continues while the loop variable is less than
the length of the array, doing something with element
[i]
in the body of the loop.
The program goes through each array element sequentially, which we refer to as
traversing
the array.
Array Traversal
Processing each array element sequentially from the first to the last.
This pattern is so useful that it is worth including it in a more general form:
for (int i = 0; i < <array>.length; i++) {
<do something with array[i]>;
}
We will see this traversal pattern repeatedly as we explore common array
algorithms.
As we discussed in the last section, we refer to array elements by combining the name
of the variable that refers to the array with an integer index inside square brackets:
<array variable>[<integer expression>]
Notice in this syntax description that the index can be an arbitrary integer expres-
sion. To explore this feature, let's examine how we would access particular values in
an array of integers. Suppose that we construct an array of length 5 and fill it up with
the first five odd integers:
int[] list = new int[5];
for (int i = 0; i < list.length; i++) {
list[i] = 2 * i + 1;
}
The first line of code declares a variable
list
of type
int[]
that refers to an array
of length 5. The array elements are auto-initialized to
0
:
[0]
[1]
[2]
[3]
[4]
list
30
30
30
30
0
Then the code uses the standard traversing loop to fill in the array with successive
odd numbers:
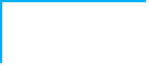
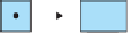

Search WWH ::

Custom Search