Java Reference
In-Depth Information
Suppose that you want to print this text with exactly one space between each pair
of words:
a new nation, conceived in liberty
How do you do that? Assume that you are writing a method that is passed a
String
to echo and a
PrintStream
object to which the output should be sent:
public static void echoFixed(String text, PrintStream output) {
...
}
You can construct a
Scanner
from the
String
and then use the
next
method to
read one word at a time. Recall that the
Scanner
class ignores whitespace, so you'll
get just the individual words without all of the spaces between them. As you read
words, you'll need to echo them to the
PrintStream
object. Here's a first attempt:
Scanner data = new Scanner(text);
while (data.hasNext()) {
output.print(data.next());
}
This code does a great job of deleting the long sequences of spaces from the
String
, but it goes too far: It eliminates all of the spaces. To get one space between
each pair of words, you'll have to include some spaces:
Scanner data = new Scanner(text);
while (data.hasNext()) {
output.print(data.next() + " ");
}
This method produces results that look pretty good, but it prints an extra space at
the end of the line. To get rid of that space so that you truly have spaces appearing
only between pairs of words, you'll have to change the method slightly. This is a
classic fencepost problem; you want to print one more word than you have spaces.
You can use the typical solution of processing the first word before the loop begins
and swapping the order of the other two operations inside the loop (printing a space
and then the word):
Scanner data = new Scanner(text);
output.print(data.next());
while (data.hasNext()) {
output.print(" " + data.next());
}


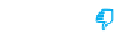

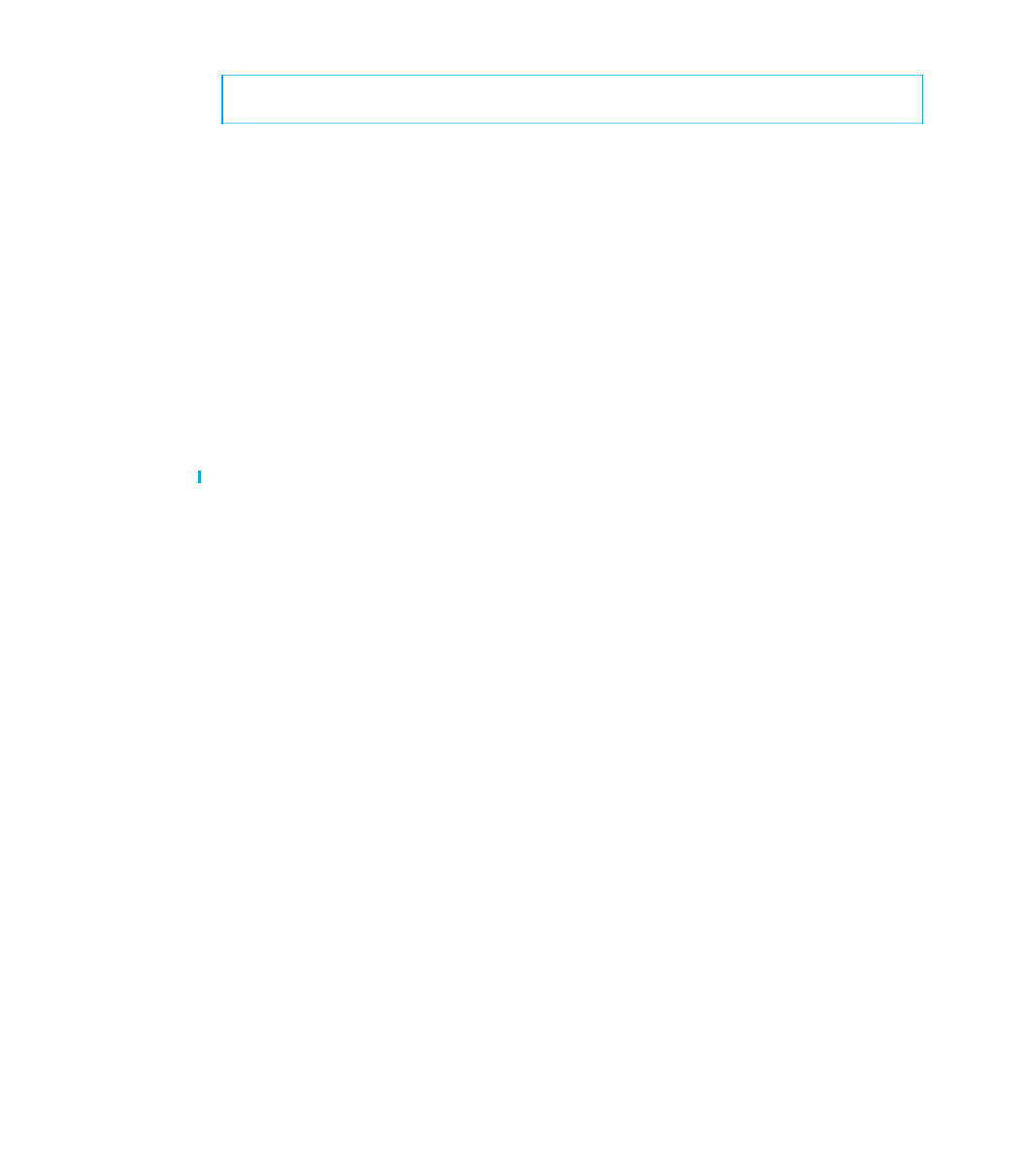

Search WWH ::

Custom Search