Java Reference
In-Depth Information
9 System.out.println("This program will add a series");
10 System.out.println("of numbers from a file.");
11 System.out.println();
12
13 Scanner console =
new
Scanner(System.in);
14 System.out.print("What is the file name? ");
15 String name = console.nextLine();
16 Scanner input =
new
Scanner(
new
File(name));
17 System.out.println();
18
19
double
sum = 0.0;
20
int
count = 0;
21
while
(input.hasNextDouble()) {
22
double
next = input.nextDouble();
23 count++;
24 System.out.println("number " + count + " = " + next);
25 sum += next;
26 }
27 System.out.println("Sum = " + sum);
28 }
29 }
Notice that the program has two different
Scanner
objects: one for reading from
the console and one for reading from the file. We read the file name using a call on
nextLine
to read an entire line of input from the user, which allows the user to type
in file names that have spaces in them. Notice that we still need the
throws
FileNotFoundException
in the header for
main
because even though we are
prompting the user to enter a file name, there won't necessarily be a file of that name.
If we have this program read from the file
numbers.dat
used earlier, it will pro-
duce the following output:
This program will add a series
of numbers from a file.
What is the file name?
numbers.dat
number 1 = 308.2
number 2 = 14.9
number 3 = 7.4
number 4 = 2.8
number 5 = 3.9
number 6 = 4.7
number 7 = -15.4
number 8 = 2.8
Sum = 329.29999999999995
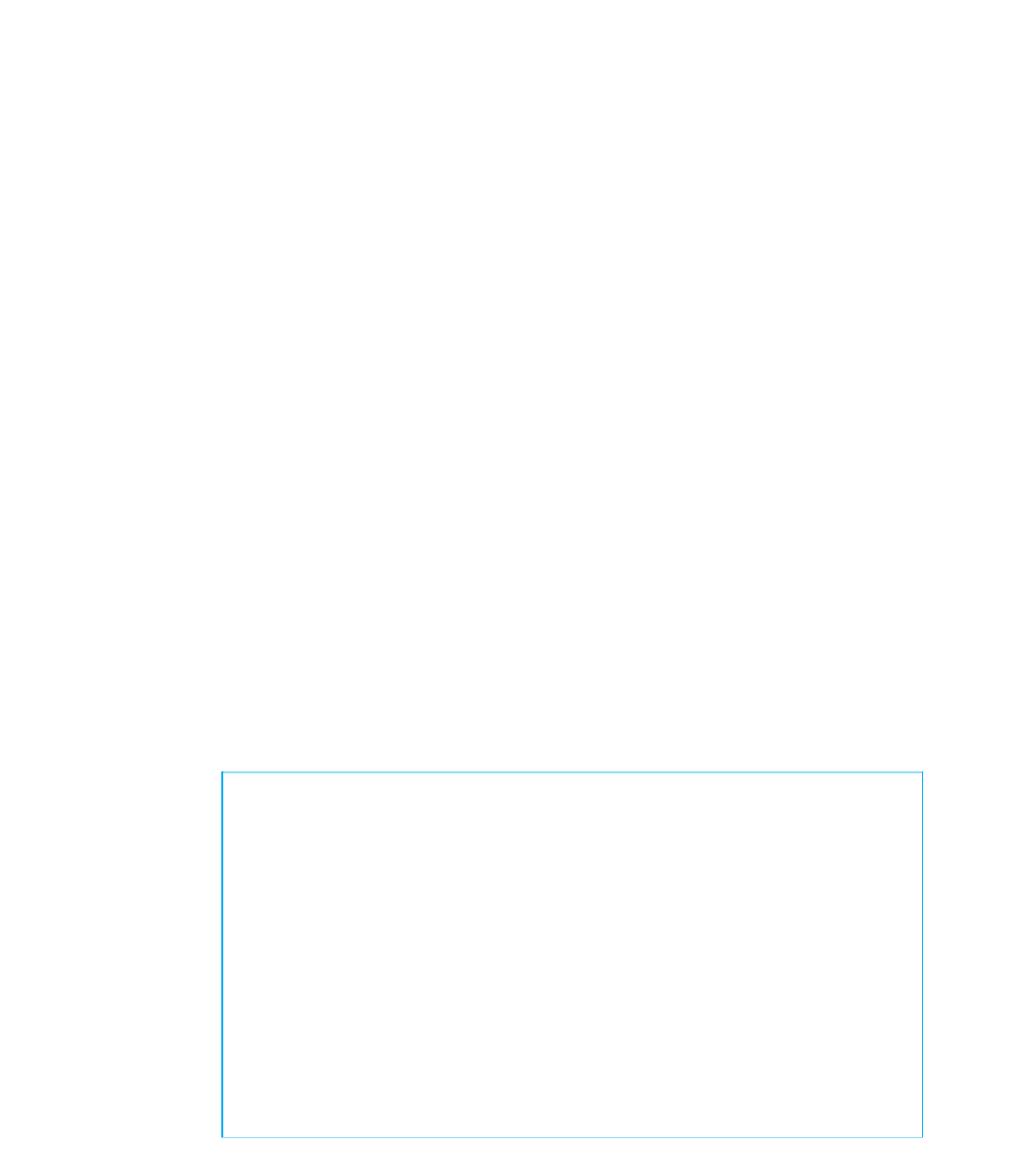
Search WWH ::

Custom Search