Java Reference
In-Depth Information
value. Remember that the value passed to
nextInt
should be one more than the
desired maximum, so we'll pass
100
:
// pick a random number between 00 and 99 inclusive
Random rand = new Random();
int number = rand.nextInt(100);
The next important feature our game should have is to give a hint when the player
makes an incorrect guess. The tricky part is figuring out how many digits of the
player's guess match the correct number. Since this code is nontrivial to write, let's
make a method called
matches
that does the work for us. To figure out how many
digits match, the
matches
method needs to use the guess and the correct number as
parameters. It will return the number of matching digits. Therefore, its header should
look like this:
public static int matches(int number, int guess) {
...
}
Our algorithm must count the number of matching digits. Either digit from the
guess can match either digit from the correct number. Since the digits are somewhat
independent—that is, whether the ones digit of the guess matches is independent of
whether the tens digit matches—we should use sequential
if
statements rather than
an
if/else
statement to represent these conditions.
The digit-matching algorithm has one special case. If the player guesses a number
such as
33
that contains two of the same digit, and if that digit is contained in the cor-
rect answer (say the correct answer is
37
), it would be misleading to report that two
digits match. It makes more sense for the program to report one matching digit. To
handle this case, our algorithm must check whether the guess contains two of the same
digit and consider the second digit of the guess to be a match only if it is different
from the first.
Here is the pseudocode for the algorithm:
matches = 0.
if (the first digit of the guess matches
either digit of the correct number) {
we have found one match.
}
if (the second digit of the guess is different from the first digit,
AND it matches either digit of the correct number) {
we have found another match.
}
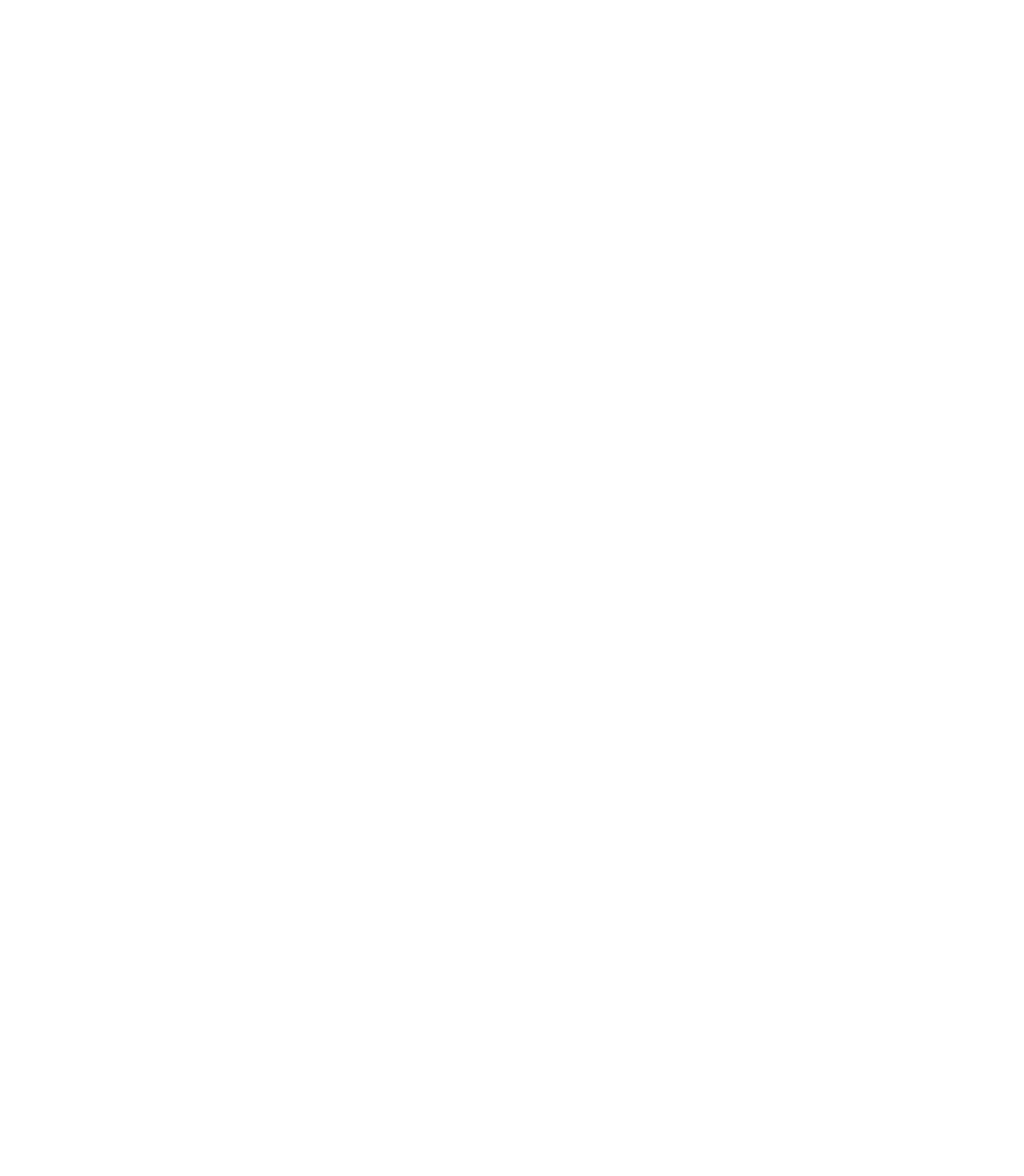
Search WWH ::

Custom Search