Java Reference
In-Depth Information
Table 5.5
De Morgan's Laws
Original expression
Negated expression
Simplified negation
p || q
!(p || q)
!p && !q
p && q
!(p && q)
!p || !q
We can use the first De Morgan's law for our two-digit number program because
we are trying to find the negation of an expression that involves the logical OR opera-
tor. Instead of writing:
while (!(number >= 10 && number <= 99))
we can say:
while (number < 10 || number > 99)
Each individual test has been negated and the AND operator has been replaced
with an OR operator.
Let's look at a second example that involves the other De Morgan's law. Suppose
that you want to ask the user a question and you want to force the user to answer
either “yes” or “no”. If you have a
String
variable called
response
, you can use the
following test to describe what you want to be true:
response.equals("yes") || response.equals("no")
If we're writing a loop to keep reading a response until this expression evaluates to
true
, then we want to write the loop so that it uses the negation of this test. So, once
again, we could use the NOT operator for the entire expression:
while (!(response.equals("yes") || response.equals("no")))
Once again it is best to simplify the expression using De Morgan's law:
while (!response.equals("yes") && !response.equals("no"))
In the previous chapter, you learned that it is good programming practice to think
about the preconditions of a method and to mention them in the comments for the
method. You also learned that in some cases your code can throw exceptions if pre-
conditions are violated.

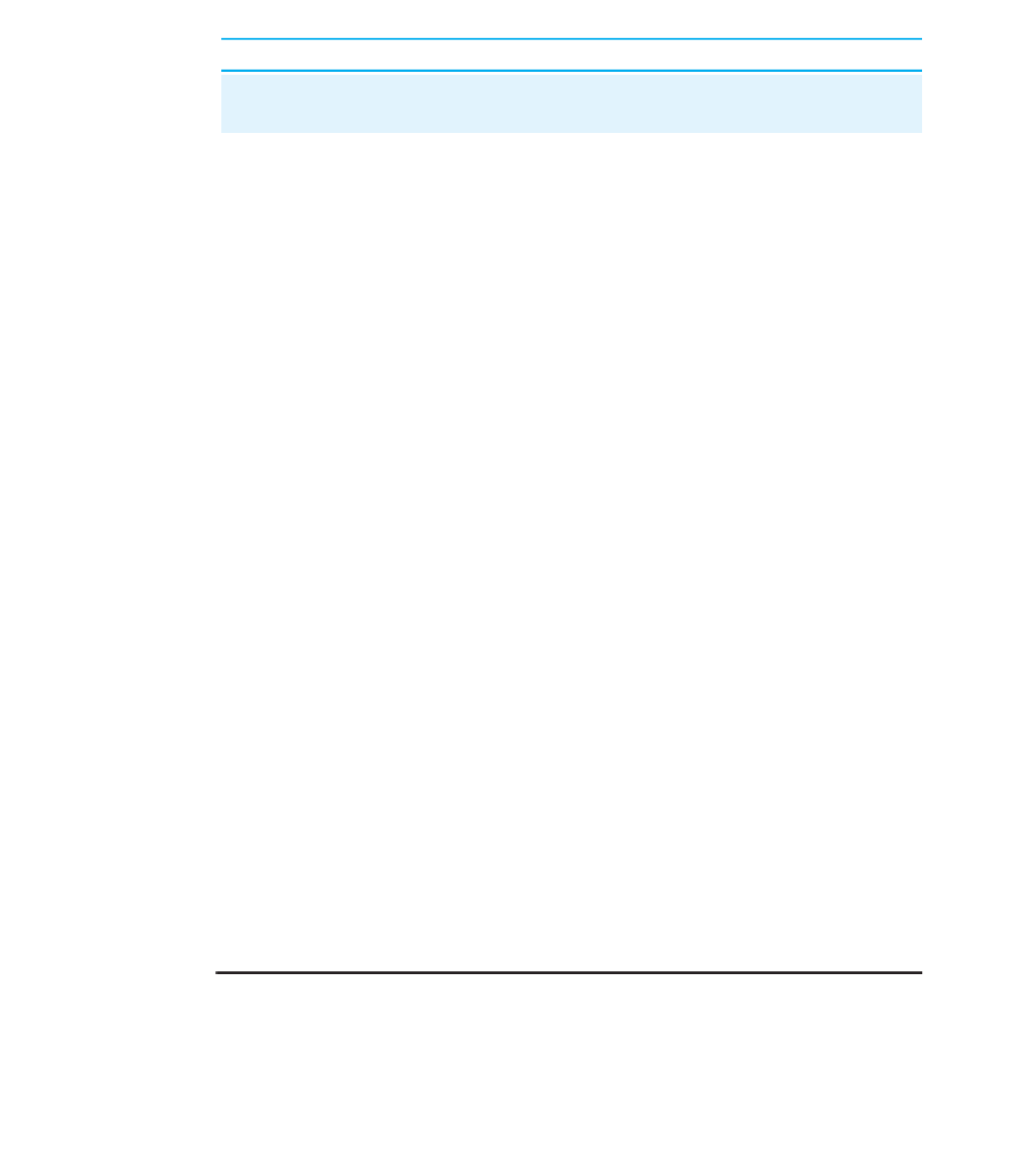
Search WWH ::

Custom Search