Java Reference
In-Depth Information
we haven't seen a space
and
that we haven't reached the end of the string. We can
express that idea using the logical
AND
operator:
int stop = 0;
while (s.charAt(stop) != ' ' && stop < s.length()) {
stop++;
}
Unfortunately, even this test does not work. It expresses the two conditions prop-
erly, because we want to make sure that we haven't reached a space and we want to
make sure that we haven't reached the end of the string. But think about what hap-
pens just as we reach the end of a string. Suppose that
s
is
"four"
and
stop
is equal
to
3
. We see that the character at index 3 is not a space and we see that
stop
is less
than the length of the string, so we increment one more time and
stop
becomes
4
. As
we come around the loop, we test whether
s.charAt(4)
is a space. This test throws
an exception. We also test whether
stop
is less than
4
, which it isn't, but that test
comes too late to avoid the exception.
Java offers a solution for this situation. The logical operators
&&
and
||
use
short-
circuited evaluation.
Short-Circuited Evaluation
The property of the logical operators
&&
and
||
that prevents the second
operand from being evaluated if the overall result is obvious from the
value of the first operand.
In our case, we are performing two different tests and asking for the logical
AND
of
the two tests. If either test fails, the overall result is
false
, so if the first test fails, it's
not necessary to perform the second test. Because of short-circuited evaluation—that
is, because the overall result is obvious from the first test—we don't perform the second
test at all. In other words, the performance and evaluation of the second test are pre-
vented (short-circuited) by the fact that the first test fails.
This means we need to reverse the order of our two tests:
int stop = 0;
while (stop < s.length() && s.charAt(stop) != ' ') {
stop++;
}
If we run through the same scenario again with
stop
equal to
3
, we pass both of
these tests and increment
stop
to
4
. Then, as we come around the loop again, we first
test to see if
stop
is less than
s.length()
. It is not, which means the test evaluates
to
false
. As a result, Java knows that the overall expression will evaluate to
false
and never evaluates the second test. This order of events prevents the exception from
occurring, because we never test whether
s.charAt(4)
is a space.

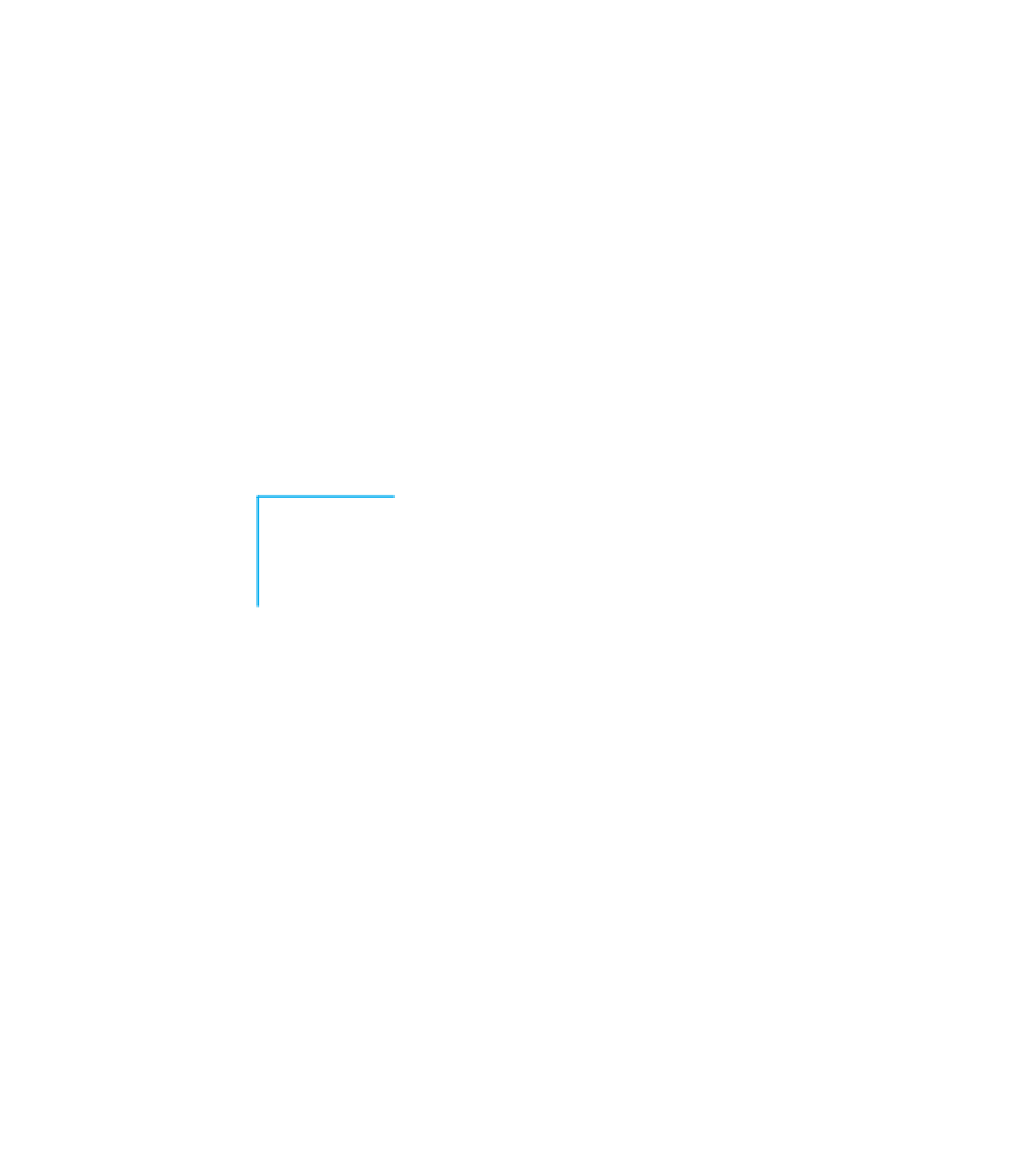
Search WWH ::

Custom Search