Java Reference
In-Depth Information
int times = console.nextInt();
if (times == 1) {
System.out.print("And how much are you contributing? ");
int donation = console.nextInt();
sum = sum + donation;
count1++;
total = total + donation;
}
if (times == 2) {
System.out.print("And how much are you contributing? ");
int donation = console.nextInt();
sum = sum + 2 * donation;
count2++;
total = total + donation;
}
Rewrite it so that it has a better structure and avoids redundancy. To simplify things, you may assume that the
user always types
1
or
2
. (How would the code need to be modified to handle any number that the user might
type?)
9.
The following code is poorly structured:
Scanner console = new Scanner(System.in);
System.out.print("How much will John be spending? ");
double amount = console.nextDouble();
System.out.println();
int numBills1 = (int) (amount / 20.0);
if (numBills1 * 20.0 < amount) {
numBills1++;
}
System.out.print("How much will Jane be spending? ");
amount = console.nextDouble();
System.out.println();
int numBills2 = (int) (amount / 20.0);
if (numBills2 * 20.0 < amount) {
numBills2++;
}
System.out.println("John needs " + numBills1 + " bills");
System.out.println("Jane needs " + numBills2 + " bills");
Rewrite it so that it has a better structure and avoids redundancy. You may wish to introduce a method to help
capture redundant code.
10.
Write a piece of code that reads a shorthand text description of a color and prints the longer equivalent. Acceptable color
names are
B
for
Blue
,
G
for
Green
, and
R
for
Red
. If the user types something other than
B
,
G
, or
R
, the program should
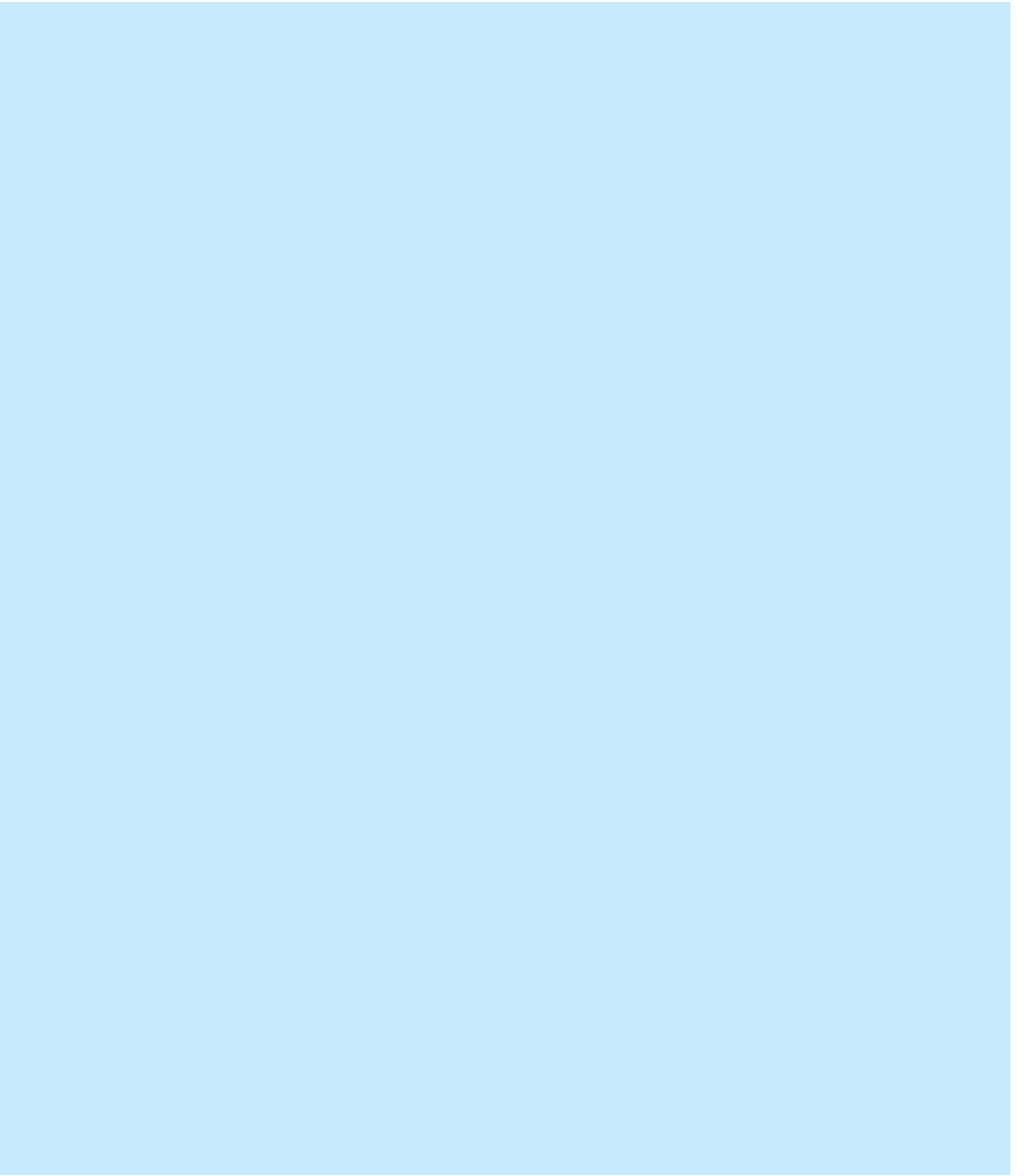
Search WWH ::

Custom Search