Java Reference
In-Depth Information
10
Scanner console =
new
Scanner(System.in);
11
double
bmi1 = getBMI(console);
12
double
bmi2 = getBMI(console);
13
reportResults(bmi1, bmi2);
14
}
15
16
// introduces the program to the user
17
public static void
giveIntro() {
18
System.out.println("This program reads data for two");
19
System.out.println("people and computes their body");
20
System.out.println("mass index and weight status.");
21
System.out.println();
22
}
23
24
// prompts for one person's statistics, returning the BMI
25
public static double
getBMI(Scanner console) {
26
System.out.println("Enter next person's information:");
27
System.out.print("height (in inches)? ");
28
double
height = console.nextDouble();
29
System.out.print("weight (in pounds)? ");
30
double
weight = console.nextDouble();
31
double
bmi = BMIFor(height, weight);
32
System.out.println();
33
return
bmi;
34
}
35
36
// this method contains the body mass index formula for
37
// converting the given height (in inches) and weight
38
// (in pounds) into a BMI
39
public static double
BMIFor(
double
height,
double
weight) {
40
return
weight / (height * height) * 703;
41
}
42
43
// reports the overall bmi values and weight status
44
public static void
reportResults(
double
bmi1,
double
bmi2) {
45
System.out.printf("Person #1 body mass index = %5.2f\n", bmi1);
46
reportStatus(bmi1);
47
System.out.printf("Person #2 body mass index = %5.2f\n",bmi2);
48
reportStatus(bmi2);
49
}
50
51
// reports the weight status for the given BMI value
52
public static void
reportStatus(
double
bmi) {
53
if
(bmi < 18.5) {
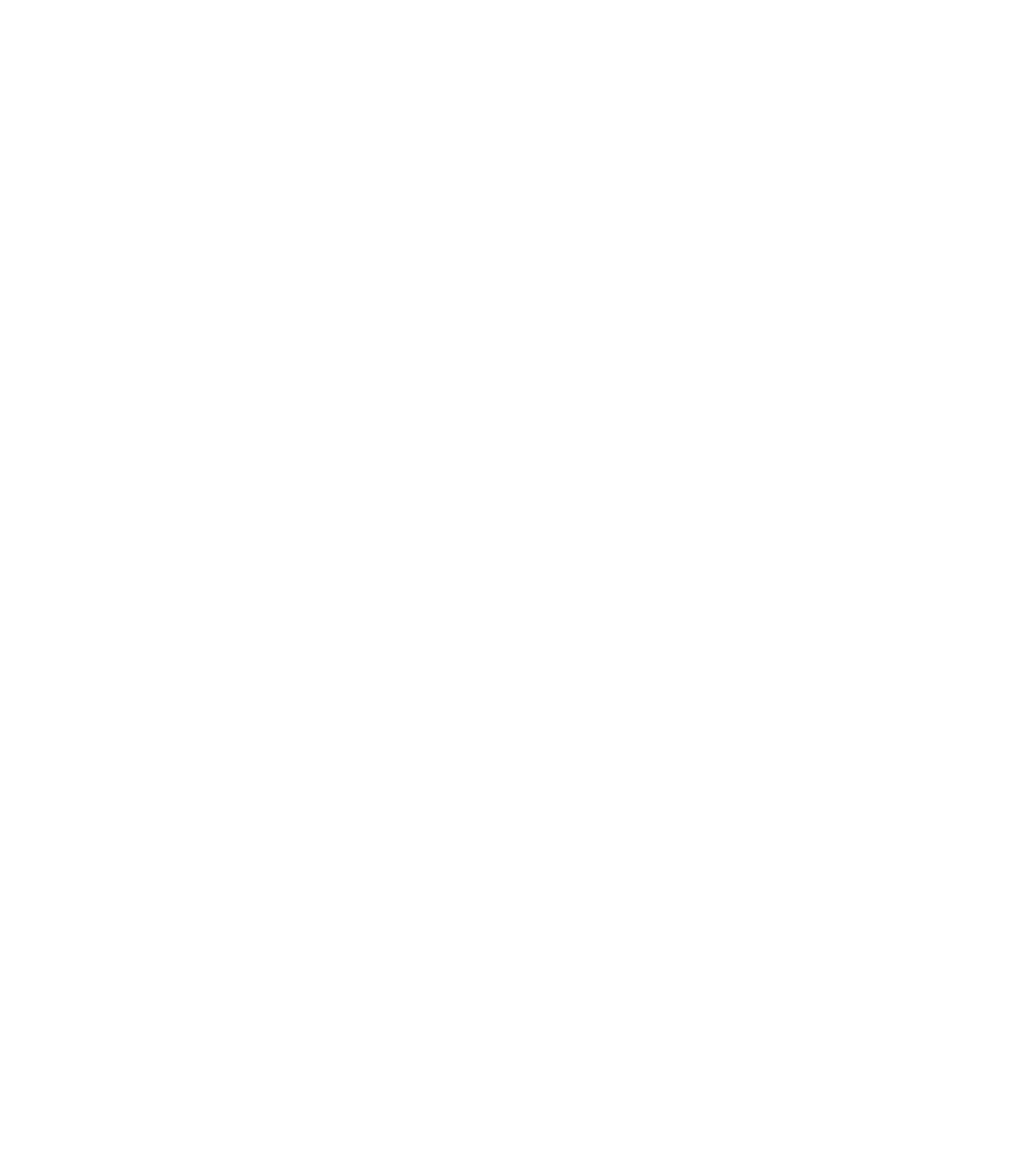
Search WWH ::

Custom Search