Java Reference
In-Depth Information
double height1 = console.nextDouble();
System.out.print("weight (in pounds)? ");
double weight1 = console.nextDouble();
Once we have the person's height and weight, we can compute the person's BMI.
The CDC website gives the following BMI formula for adults:
weight
(lb)
[height
(in)]
2
*
703
This formula is fairly easy to translate into a Java expression:
double bmi1 = weight1 / (height1 * height1) * 703;
If you look closely at the sample execution, you will see that we want to print
blank lines to separate different parts of the user interaction. The introduction ends
with a blank line, then there is a blank line after the “prompt and read” portion of the
interaction. So, after we add an empty
println
and put all of these pieces together,
our
main
method looks like this:
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
System.out.println("Enter next person's information:");
System.out.print("height (in inches)? ");
double height1 = console.nextDouble();
System.out.print("weight (in pounds)? ");
double weight1 = console.nextDouble();
double bmi1 = weight1 / (height1 * height1) * 703;
System.out.println();
...
}
This program prompts for values and computes the BMI. Now we need to include
code to report the results. We could use a
println
for the BMI:
System.out.println("Person #1 body mass index = " + bmi1);
This would work, but it produces output like the following:
Person #1 body mass index = 29.930121708547368
The long sequence of digits after the decimal point is distracting and implies a
level of precision that we simply don't have. It is more appropriate and more appeal-
ing to the user to list just a few digits after the decimal point. This is a good place to
use a
printf
:
System.out.printf("Person #1 body mass index = %5.2f\n", bmi1);
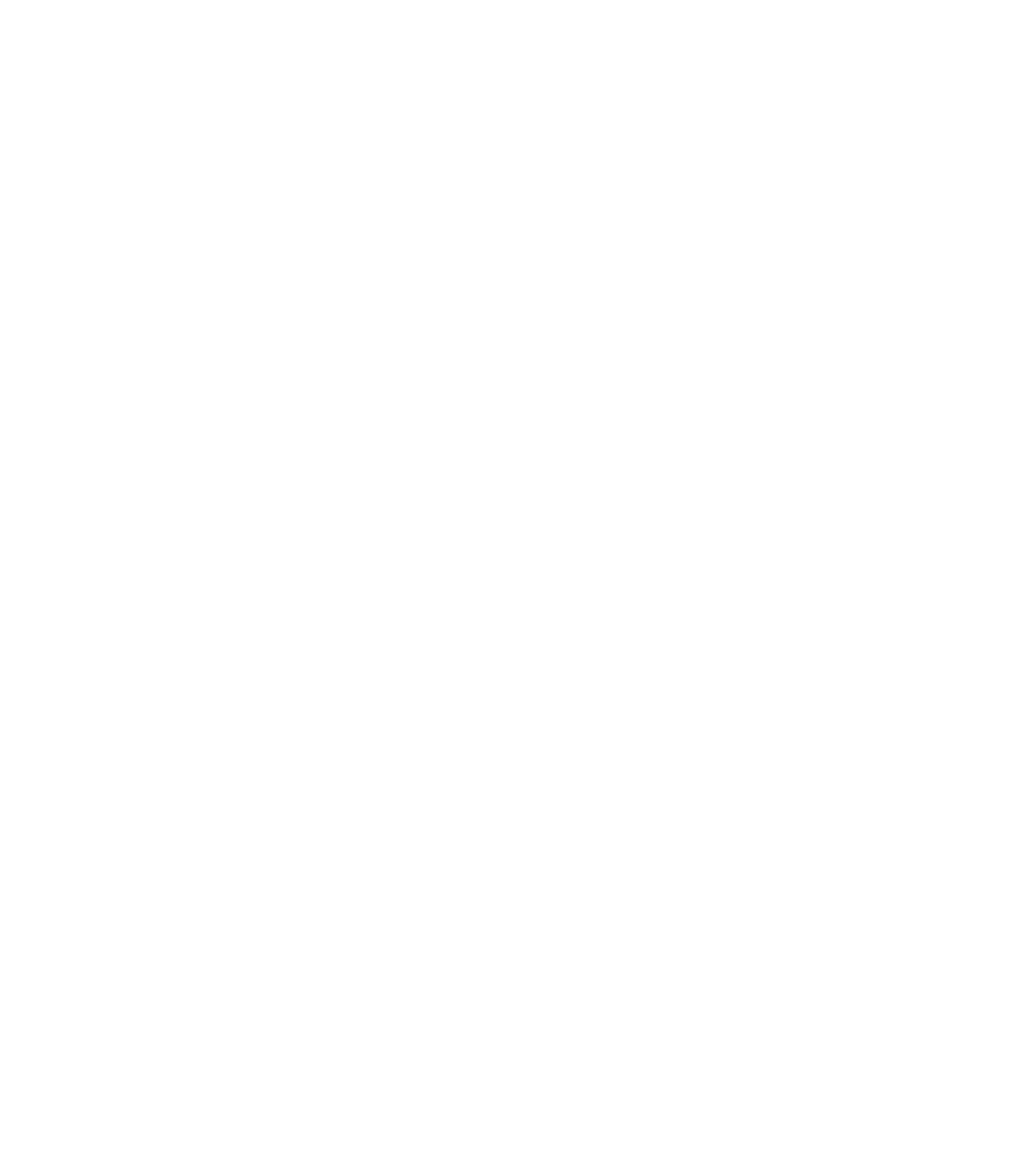
Search WWH ::

Custom Search