Java Reference
In-Depth Information
To complete this code, we have to refine what to do when “we've found it.” If we
find the character, we have our answer: the current value of the variable
i
. And if that
is the answer we want to return, we can put a
return
statement there:
for (int i = 0; i < s.length(); i++) {
if (s.charAt(i) == ch) {
return i;
}
}
To understand this code, you have to understand how the
return
statement works.
For example, if the
Strings
is the one from our example (“four score...”) and we
are searching for the character
'r'
, we know that when
i
is equal to
3
we will find
that
s.charAt(3)
is equal to
'r'
. That case causes our code to execute the
return
statement, effectively saying:
return 3;
When a
return
statement is executed, Java immediately exits the method, which
means that we break out of the loop and return
3
as our answer. Even though the loop
would normally increment
i
to
4
and keep going, our code doesn't do that because
we hit the
return
statement.
There is only one thing missing from our code. If we try to compile it as it is, we
get this error message from the Java compiler:
missing return statement
This error message occurs because we haven't told Java what to do if we never
find the character we are searching for. In that case, we will execute the
for
loop in
its entirety and reach the end of the method without having returned a value. This is
not acceptable. If we say that the method returns an
int
, we have to guarantee that
every path through the method will return an
int
.
If we don't find the character, we want to return some kind of special value to
indicate that the character was not found. We can't use the value
0
, because
0
is a
legal index for a
String
(the index of the first character). So, the convention in Java
is to return
-1
if the character is not found. It is easy to add the code for this
return
statement after the
for
loop:
public static int indexOf(char ch, String s) {
for (int i = 0; i < s.length(); i++) {
if (s.charAt(i) == ch) {
return i;
}
}
return -1;
}

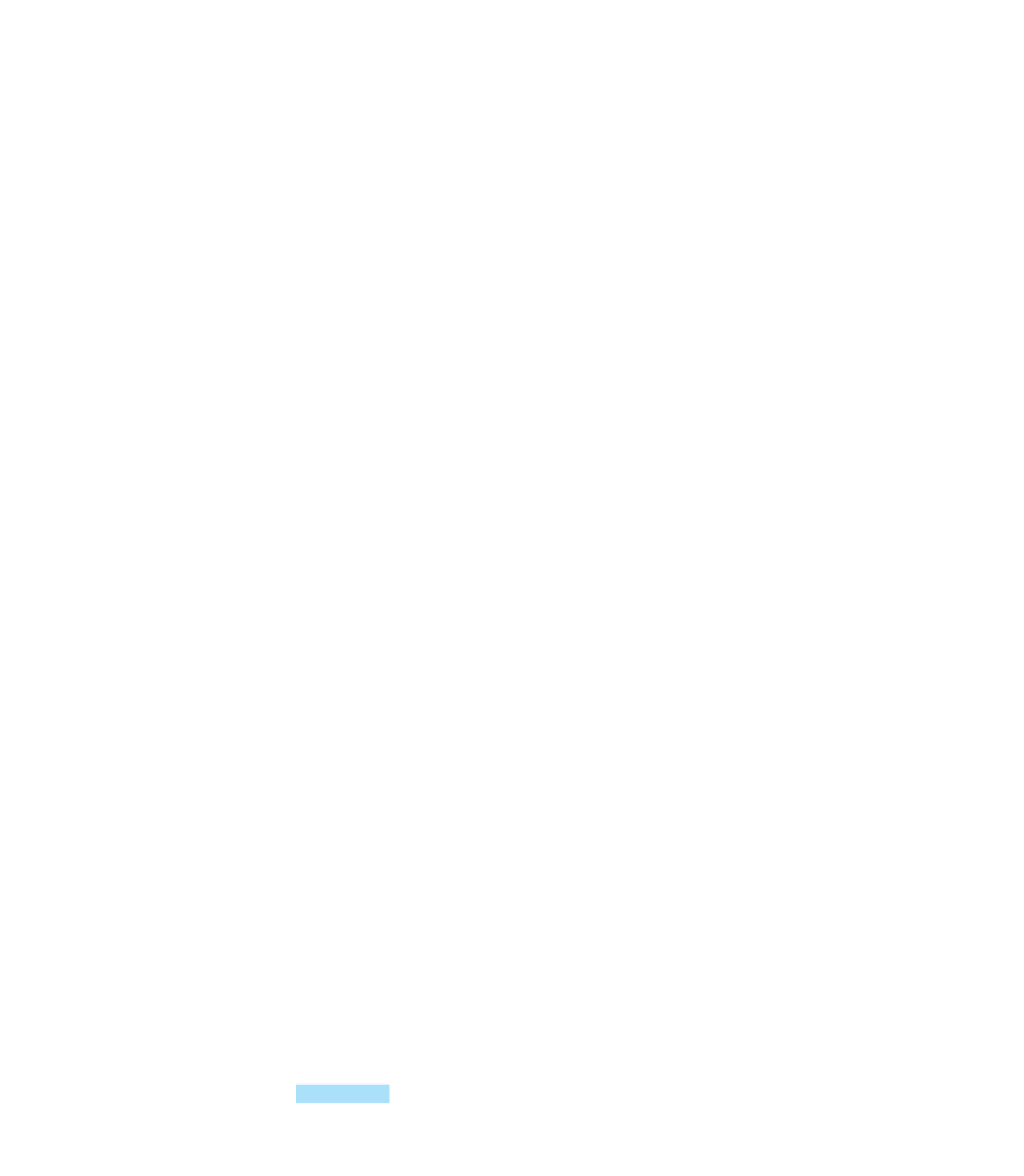
Search WWH ::

Custom Search