Java Reference
In-Depth Information
In Java, exceptions are objects. Before you can throw an exception, you have
to construct an exception object using
new
. You'll normally construct the object as
you are throwing the exception, because the exception object includes information
about what was going on when the error occurred. Java has a class called
IllegalArgumentException
that is meant to cover a case like this where someone
has passed an inappropriate value as an argument. You can construct the exception
object and include it in a
throw
statement as follows:
throw new IllegalArgumentException();
Of course, you'll want to do this only when the precondition fails, so you need to
include the code inside an
if
statement:
if (n < 0) {
throw new IllegalArgumentException();
}
You can also include some text when you construct the exception that will be dis-
played when the exception is thrown:
if (n < 0) {
throw new IllegalArgumentException("negative n: " + n);
}
Incorporating the
pre/post
comments and the exception code into the method
definition, you get the following code:
// pre : n >= 0
// post: returns n factorial (n!)
public static int factorial(int n) {
if (n < 0) {
throw new IllegalArgumentException("negative n: " + n);
}
int product = 1;
for (int i = 2; i <= n; i++) {
product *= i;
}
return product;
}
You don't need an
else
after the
if
that throws the exception, because when an
exception is thrown, it halts the execution of the method. So, if someone calls the
factorial
method with a negative value of
n
, Java will never execute the code that
follows the
throw
statement.





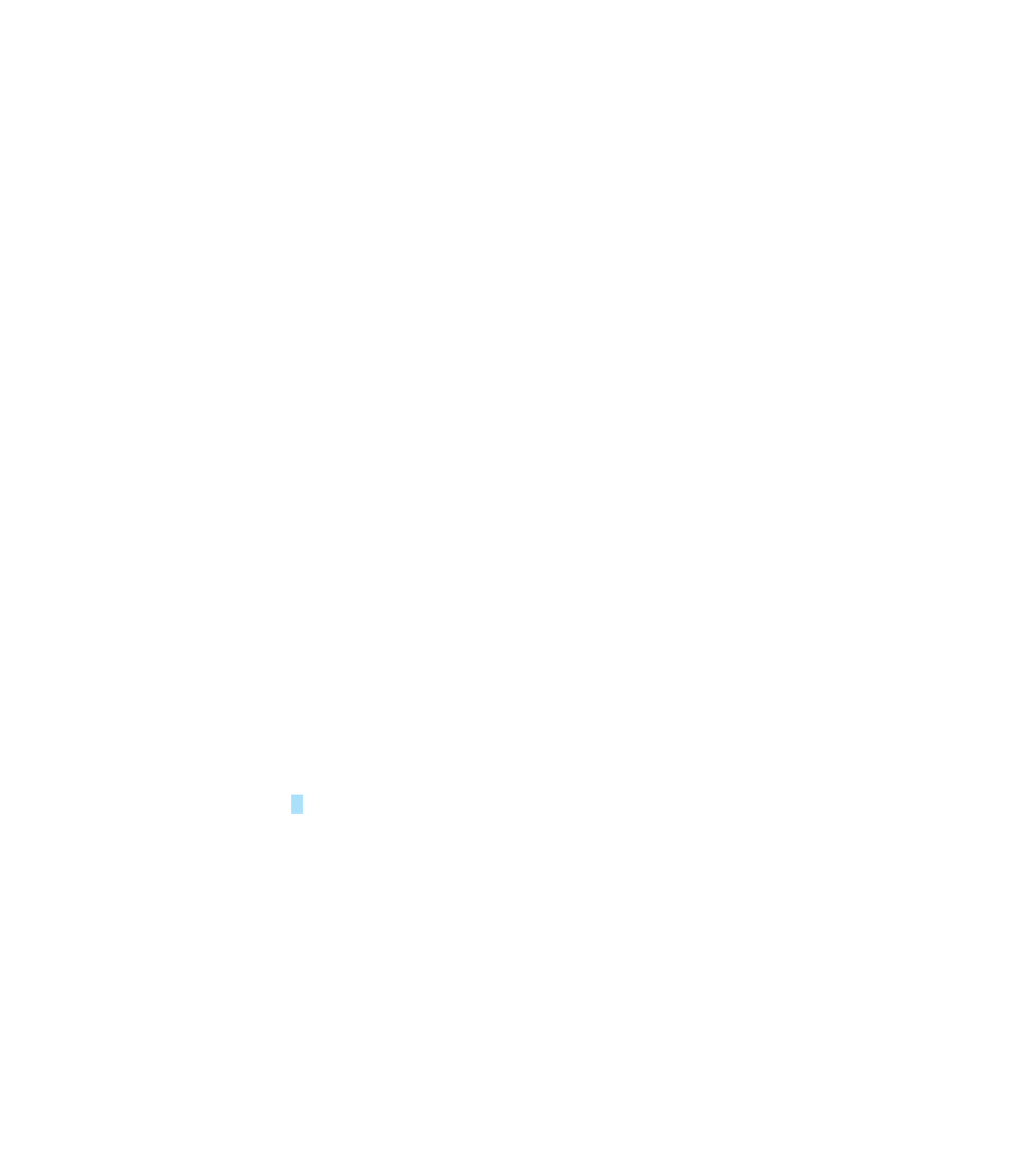
Search WWH ::

Custom Search