Java Reference
In-Depth Information
Table 4.5
Useful Methods of the
Character
Class
Method
Description
Example
Converts a character that looks
getNumericValue(ch)
Character.getNumericValue('6')
like a number into that number
returns
6
Whether or not the character is
isDigit(ch)
Character.isDigit('X')
one of the digits
'0'
through
'9'
returns
false
Whether or not the character is in
isLetter(ch)
Character.isLetter('f')
the range
'a'
to
'z'
or
'A'
to
'Z'
returns
true
Whether or not the character is a
isLowerCase(ch)
Character.isLowerCase('Q')
lowercase letter
returns
false
Whether or not the character is an
isUpperCase(ch)
Character.isUpperCase('Q')
uppercase letter
returns
true
The lowercase version of the given
toLowerCase(ch)
Character.toLowerCase('Q')
letter
returns
'q'
The uppercase version of the given
toUpperCase(ch)
Character.toUpperCase('x')
letter
returns
'X'
concatenating individual characters in the loop. This is called a
cumulative concate-
nation
. The following method accepts a string and returns the same characters in the
reverse order:
public static String reverse(String phrase) {
String result = "";
for (int i = 0; i < phrase.length(); i++) {
result = phrase.charAt(i) + result;
}
return result;
}
For example, the call of
reverse("Tin man")
returns
"nam niT"
.
Several useful methods can be called to check information about a character or
convert one character into another. Remember that
char
is a primitive type, which
means that you can't use the dot syntax used with
String
s. Instead, the methods are
static methods in a class called
Character
; the methods accept
char
parameters and
return appropriate values. Some of the most useful
Character
methods are listed in
Table 4.5.
The following method counts the number of letters A-Z in a
String
, ignoring all
nonletter characters such as punctuation, numbers, and spaces:
public static int countLetters(String phrase) {
int count = 0;
for (int i = 0; i < phrase.length(); i++) {
char ch = phrase.charAt(i);
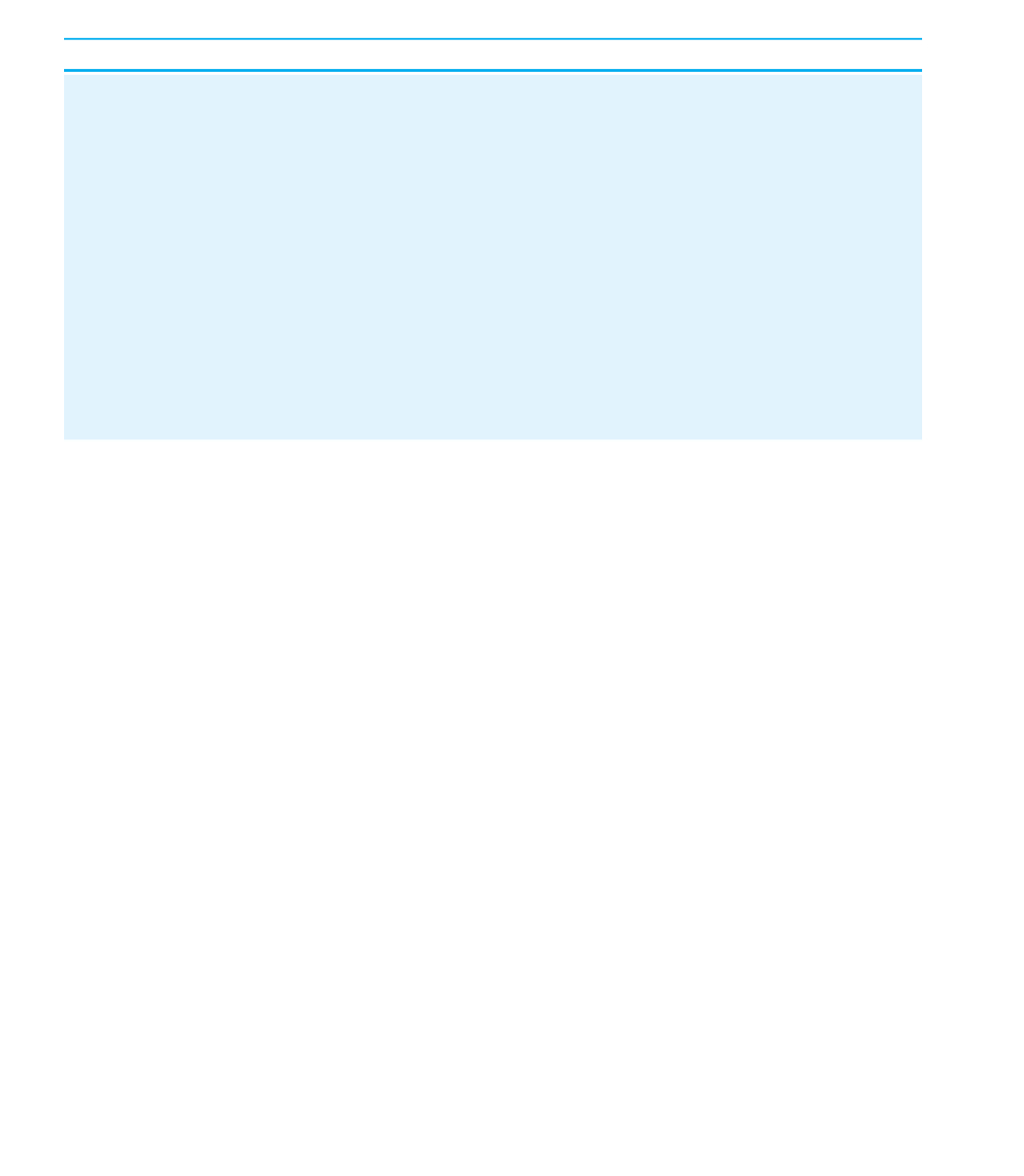
Search WWH ::

Custom Search