Java Reference
In-Depth Information
If you use a calculator, you will find that the four numbers add up to 11.1. If you
divide this number by 4, you get 2.775. Yet Java reports the result as
2.7750000000000004
. Where do all of those zeros come from, and why does the
number end in 4? The answer is that floating-point numbers can lead to
roundoff errors.
Roundoff Error
A numerical error that occurs because floating-point numbers are stored as
approximations rather than as exact values.
Roundoff errors are generally small and can occur in either direction (slightly high
or slightly low). In the previous case, we got a roundoff error that was slightly high.
Floating-point numbers are stored in a format similar to scientific notation, with a
set of digits and an exponent. Consider how you would store the value one-third in
scientific notation using base-10. You would state that the number is 3.33333 (repeat-
ing) times 10 to the -1 power. We can't store an infinite number of digits on a com-
puter, though, so we'll have to stop repeating the 3s at some point. Suppose we can
store 10 digits. Then the value for one-third would be stored as 3.333333333 times 10
to the -1. If we multiply that number by 3, we don't get back 1. Instead, we get
9.999999999 times 10 to the -1 (which is equal to 0.9999999999).
You might wonder why the numbers we used in the previous example caused a
problem when they didn't have any repeating digits. You have to remember that the
computer stores numbers in base-2. Numbers like 2.1 and 5.4 might look like simple
numbers in base-10, but they have repeating digits when they are stored in base-2.
Roundoff errors can lead to rather surprising outcomes. For example, consider the
following short program:
1
public class
Roundoff {
2
public static void
main(String[] args) {
3
double
n = 1.0;
4
for
(
int
i = 1; i <= 10; i++) {
5 n += 0.1;
6 System.out.println(n);
7 }
8 }
9 }
This program presents a classic cumulative sum with a loop that adds 0.1 to the
number
n
each time the loop executes. We start with
n
equal to
1.0
and the loop iter-
ates 10 times, which we might expect to print the numbers 1.1, 1.2, 1.3, and so on
through 2.0. Instead, it produces the following output:
1.1
1.2000000000000002
1.3000000000000003

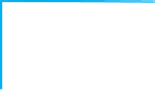
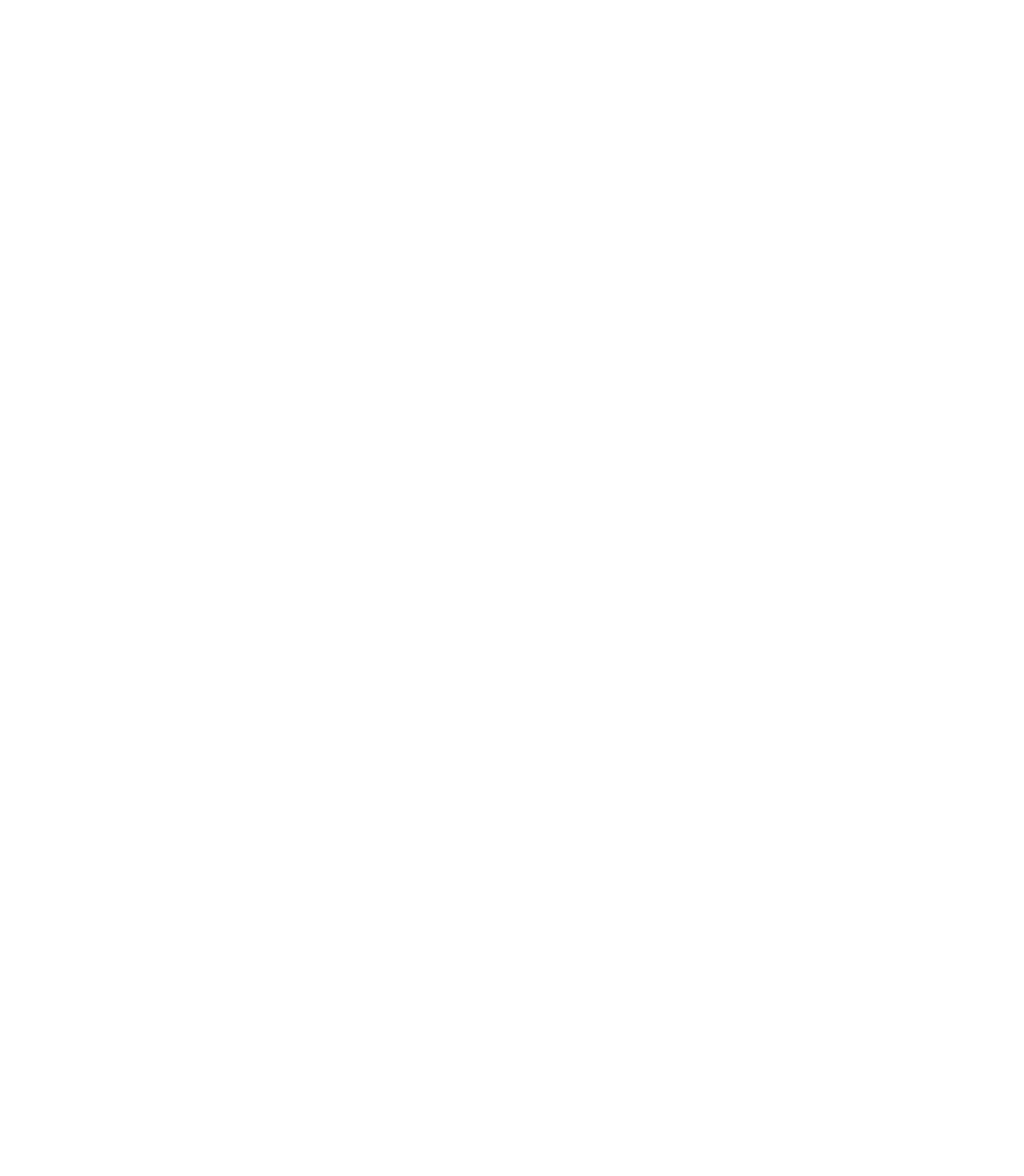
Search WWH ::

Custom Search