Java Reference
In-Depth Information
This goal is easy to accomplish with an
if
statement and an integer variable called a
counter.
You start by initializing the counter to
0
:
int negatives = 0;
You can use any name you want for the variable. Here we used
negatives
because that is what you're counting. The other essential step is to increment the
counter inside the loop if it passes the test of interest:
if (next < 0) {
negatives++;
}
When you put this all together and modify the comments and introduction, you
end up with the following variation of the cumulative sum program:
1 // Finds the average of a sequence of numbers as well as
2 // reporting how many of the user-specified numbers were negative.
3
4
import
java.util.*;
5
6
public class
ExamineNumbers2 {
7
public static void
main(String[] args) {
8 System.out.println("This program examines a sequence");
9 System.out.println("of numbers to find the average");
10 System.out.println("and count how many are negative.");
11 System.out.println();
12
13 Scanner console =
new
Scanner(System.in);
14
15 System.out.print("How many numbers do you have? ");
16
int
totalNumber = console.nextInt();
17
18
int
negatives = 0;
19
double
sum = 0.0;
20
for
(
int
i = 1; i <= totalNumber; i++) {
21 System.out.print(" #" + i + "? ");
22
double
next = console.nextDouble();
23 sum += next;
24
if
(next < 0) {
25 negatives++;
26 }
27 }
28 System.out.println();
29
30
if
(totalNumber <= 0) {
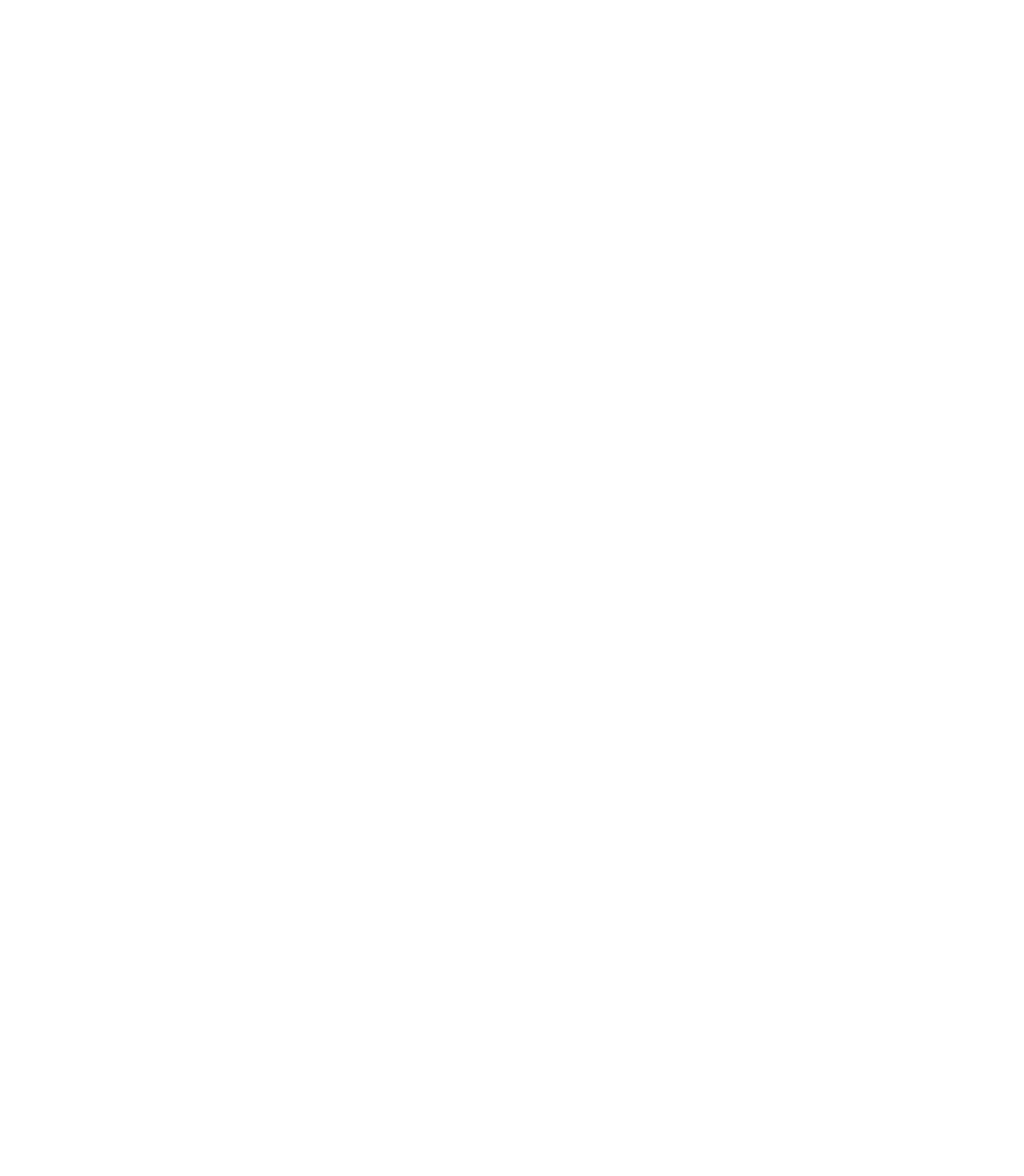
Search WWH ::

Custom Search