Java Reference
In-Depth Information
if (s.equals("yes")) {
processYes();
} else if (s.equals("no")) {
processNo();
} else {
System.out.println("You didn't type yes or no");
}
Remember when you're working with strings that you should always call the
equals
method rather than using
==
.
The
String
class also has a special variation of the
equals
method called
equalsIgnoreCase
that ignores case differences (uppercase versus lowercase letters).
For example, you could rewrite the preceding code as follows to recognize responses
like “Yes,” “YES,” “No,” “NO,” yES”, and so on:
System.out.print("yes or no? ");
String s = console.next();
if (s.equalsIgnoreCase("yes")) {
processYes();
} else if (s.equalsIgnoreCase("no")) {
processNo();
} else {
System.out.println("You didn't type yes or no");
}
Suppose you are writing a program that plays a betting game with a user and you
want to give different warnings about how much cash the user has left. The nested
if/else
construct that follows distinguishes three different cases: funds less than
$500, which is considered low; funds between $500 and $1000, which is considered
okay; and funds over $1000, which is considered good. Notice that the user is given
different advice in each case:
if (money < 500) {
System.out.println("You have $" + money + " left.");
System.out.print("Cash is dangerously low. Bet carefully.");
System.out.print("How much do you want to bet? ");
bet = console.nextInt();
} else if (money < 1000) {
System.out.println("You have $" + money + " left.");
System.out.print("Cash is somewhat low. Bet moderately.");
System.out.print("How much do you want to bet? ");
bet = console.nextInt();
} else {






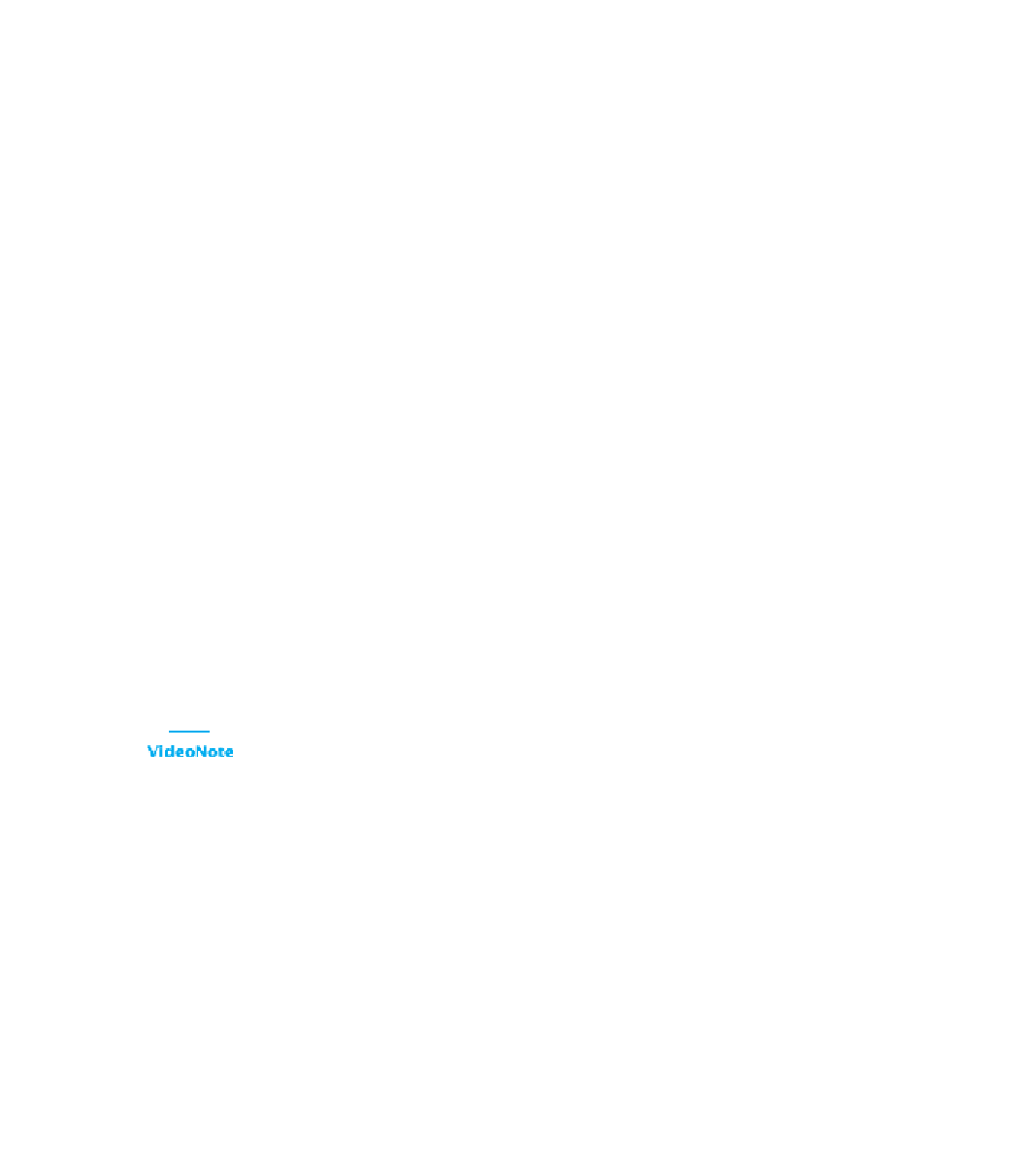
Search WWH ::

Custom Search