Java Reference
In-Depth Information
Continued from previous page
}
if (score >= 80) {
grade = "B";
}
if (score >= 70) {
grade = "C";
}
if (score >= 60) {
grade = "D";
}
if (score < 60) {
grade = "F";
}
However, if you then try to use the variable
grade
after this code, you'll get
this error from the compiler:
variable grade might not have been initialized
This is a clue that there is a problem. The Java compiler is saying that it
believes there are paths through this code that will leave the variable
grade
uninitialized. In fact, the variable will always be initialized, but the compiler can-
not figure this out. We can fix this problem by giving an initial value to
grade
:
String grade = "no grade";
This change allows the code to compile. But if you compile and run the pro-
gram, you will find that it gives out only two grades: D and F. Anyone who has a
score of at least 60 ends up with a D and anyone with a grade below 60 ends up
with an F. And even though the compiler complained that there was a path that
would allow
grade
not to be initialized, no one ever gets a grade of “no grade.”
The problem here is that you want to execute exactly one of the assignment
statements, but when you use sequential
if
statements, it's possible for the pro-
gram to execute several of them sequentially. For example, if a student has a score
of 95, that student's
grade
is set to
"A"
, then reset to
"B"
, then reset to
"C"
, and
finally reset to
"D"
. You can fix this problem by using a nested
if/else
construct:
String grade;
if (score >= 90) {
grade = "A";
} else if (score >= 80) {
Continued on next page
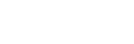
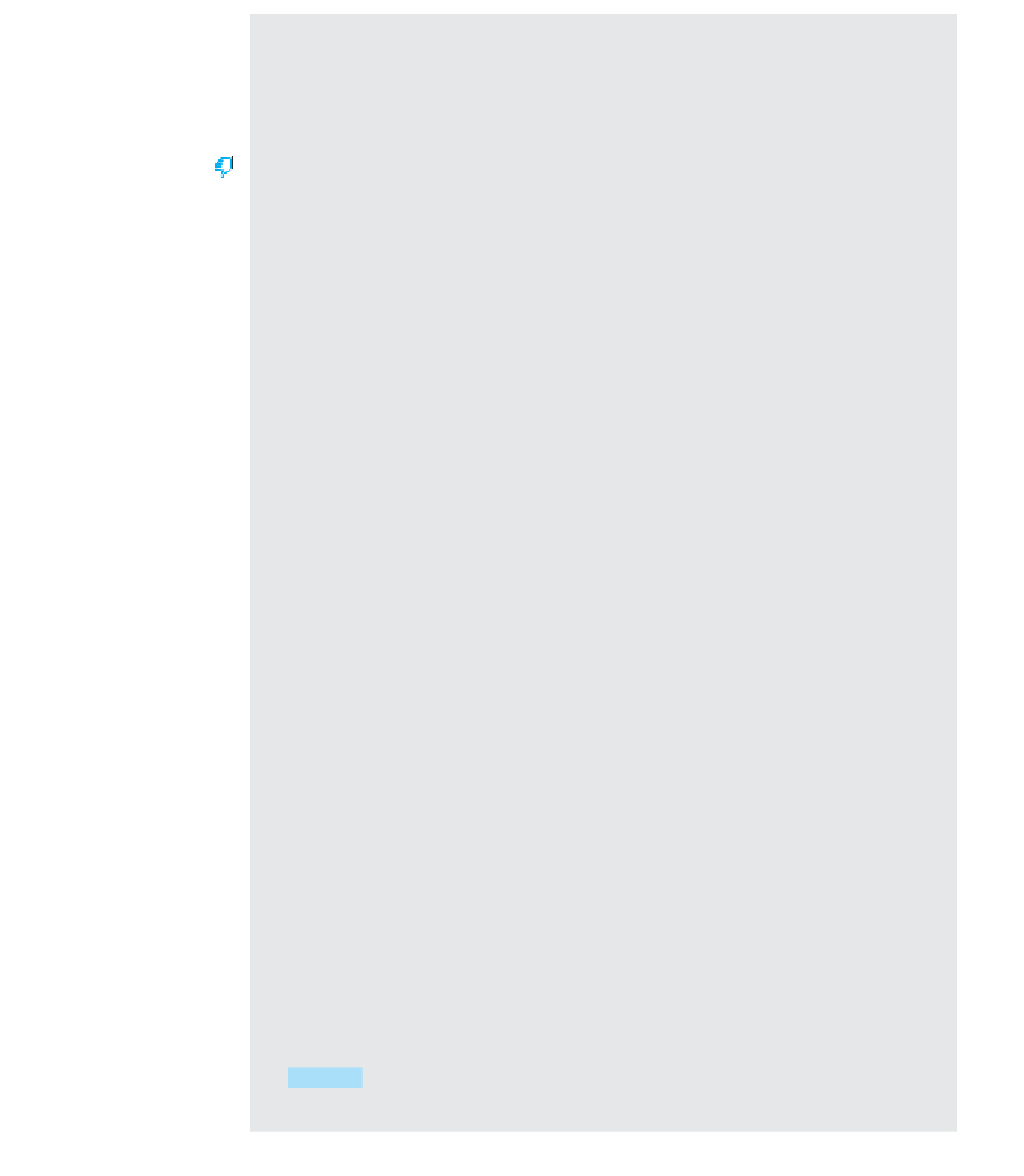
Search WWH ::

Custom Search