Java Reference
In-Depth Information
Table 3.3
Useful Methods of
String
Objects
Method
Description
Example (assuming
s
is
"hello
"
)
character at a specific index
s.charAt(1)
returns
'e'
charAt(index)
whether or not the string ends
with some text
s.endsWith("llo")
returns
true
endsWith(text)
index of a particular character
or
String
(
-1
if not present)
s.indexOf("o")
returns
4
indexOf(text)
number of characters in
the string
s.length()
returns
5
length()
whether or not the string
startsWith(text)
s.startsWith("hi")
starts with some text
returns
false
characters from start index
s.substring(1, 3)
returns
"el"
substring(start,
to just before stop index
stop)
a new string with all
lowercase letters
s.toLowerCase()
returns
"hello"
toLowerCase()
a new string with all
uppercase letters
s.toUpperCase()
returns
"HELLO"
toUpperCase()
You might think that this will turn the string
s
into its uppercase equivalent, but it
doesn't. The second line of code constructs a new string that has the uppercase equiv-
alent of the value of
s
, but we don't do anything with this new value. In order to turn
the string into uppercase, the key is to either store this new string in a different vari-
able or reassign the variable
s
to point to the new string:
String s = "Hello Maria";
s = s.toUpperCase();
System.out.println(s);
This version of the code produces the following output:
HELLO MARIA
The
toUpperCase
and
toLowerCase
methods are particularly helpful when you
want to perform string comparisons in which you ignore the case of the letters involved.
As you've seen, you can easily produce output in the console window by calling
System.out.println
and
System.out.print
. You can also write programs that
pause and wait for the user to type a response. Such programs are known as
interactive
programs, and the responses typed by the user are known as
console input.
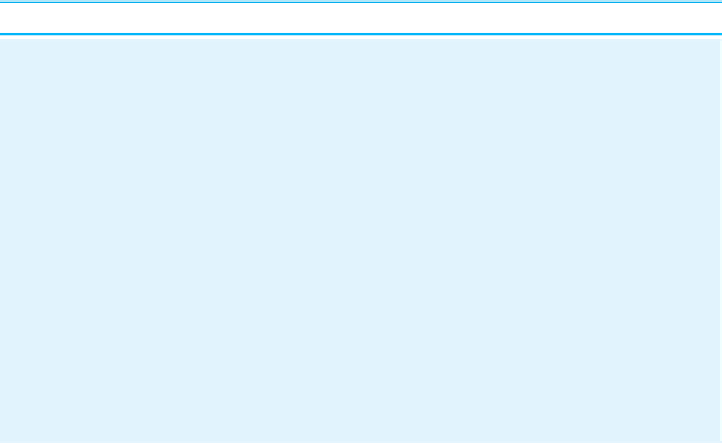







Search WWH ::

Custom Search