Java Reference
In-Depth Information
indexed starting at 0, this task is easier to write with
for
loops that start with
0
rather
than
1
. Consider, for example, the following code that prints out the individual char-
acters of
s1
:
String s1 = "hello";
for (int i = 0; i < s1.length(); i++) {
System.out.println(i + ": " + s1.charAt(i));
}
This code produces the following output:
0: h
1: e
2: l
3: l
4: o
Remember that when we start loops at 0, we usually test with less than (
<
) rather
than less than or equal to (
<=
). The string
s1
has five characters in it, so the call on
s1.length()
will return
5
. But because the first index is 0, the last index will be one
less than 5, or 4. This convention takes a while to get used to, but zero-based index-
ing is used throughout Java, so you'll eventually get the hang of it.
Another useful
String
method is the
substring
method. It takes two integer
arguments representing a starting and ending index. When you call the
substring
method, you provide two of these indexes: the index of the first character you want
and the index just past the last index that you want.
Recall that the
String s2
has the following positions:
0
1
2
3
4
5
6
7
8
9
10
11
h
o
w
3
a
r
e
3
y
o
u
?
If you want to pull out the individual word “how” from this string, you'd ask for
s2.substring(0, 3)
Remember that the second value that you pass to the
substring
method is sup-
posed to be one beyond the end of the substring you are forming. So, even though there
is a space at position 3 in the original string, it will not be part of what you get from the
call on
substring
. Instead, you'll get all the characters just before position 3.
Following this rule means that sometimes you will give a position to a substring at
which there is no character. For instance, the last character in the string to which
s2
refers is at index 11 (the question mark). If you want to get the substring “you?”
including the question mark, you'd ask for
s2.substring(8, 12)
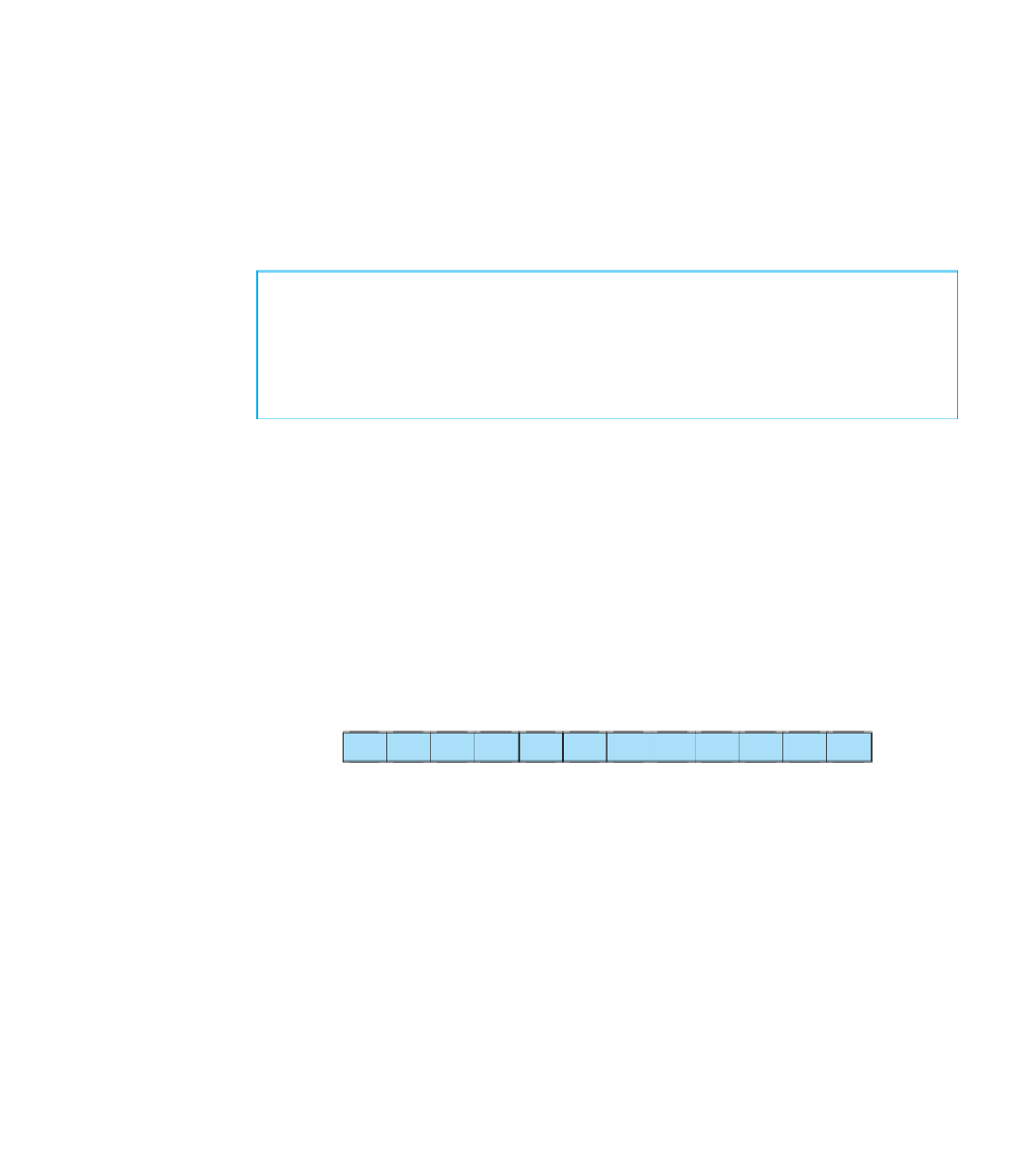
Search WWH ::

Custom Search