Java Reference
In-Depth Information
Continued from previous page
This confusion is due to the fact that parameters' types are written in the dec-
laration of the method, like this:
public static void writeSpaces(int number)
Types must be written when variables or parameters are declared, but when
variables are used, such as when the code calls a method and passes the variables
as actual parameters, their types are not written. Actual parameters are not decla-
rations; therefore, types should not be written before them:
writeSpaces(spaces1); // much better!
We've seen that a parameter can be used to provide input to a method. But while you
can use a parameter to send a value into a method, you can't use a parameter to get a
value out of a method.
When a parameter is set up, a local variable is created and is initialized to
the value being passed as the actual parameter. The net effect is that the local variable
is a copy of the value coming from the outside. Since it is a local variable, it can't
influence any variables outside the method. Consider the following sample program:
1
public class
ParameterExample2 {
2
public static void
main(String[] args) {
3
int
x = 17;
4 doubleNumber(x);
5 System.out.println("x = " + x);
6 System.out.println();
7
8
int
number = 42;
9 doubleNumber(number);
10 System.out.println("number = " + number);
11 }
12
13
public static void
doubleNumber(
int
number) {
14 System.out.println("Initial value = " + number);
15 number *= 2;
16 System.out.println("Final value = " + number);
17 }
18 }
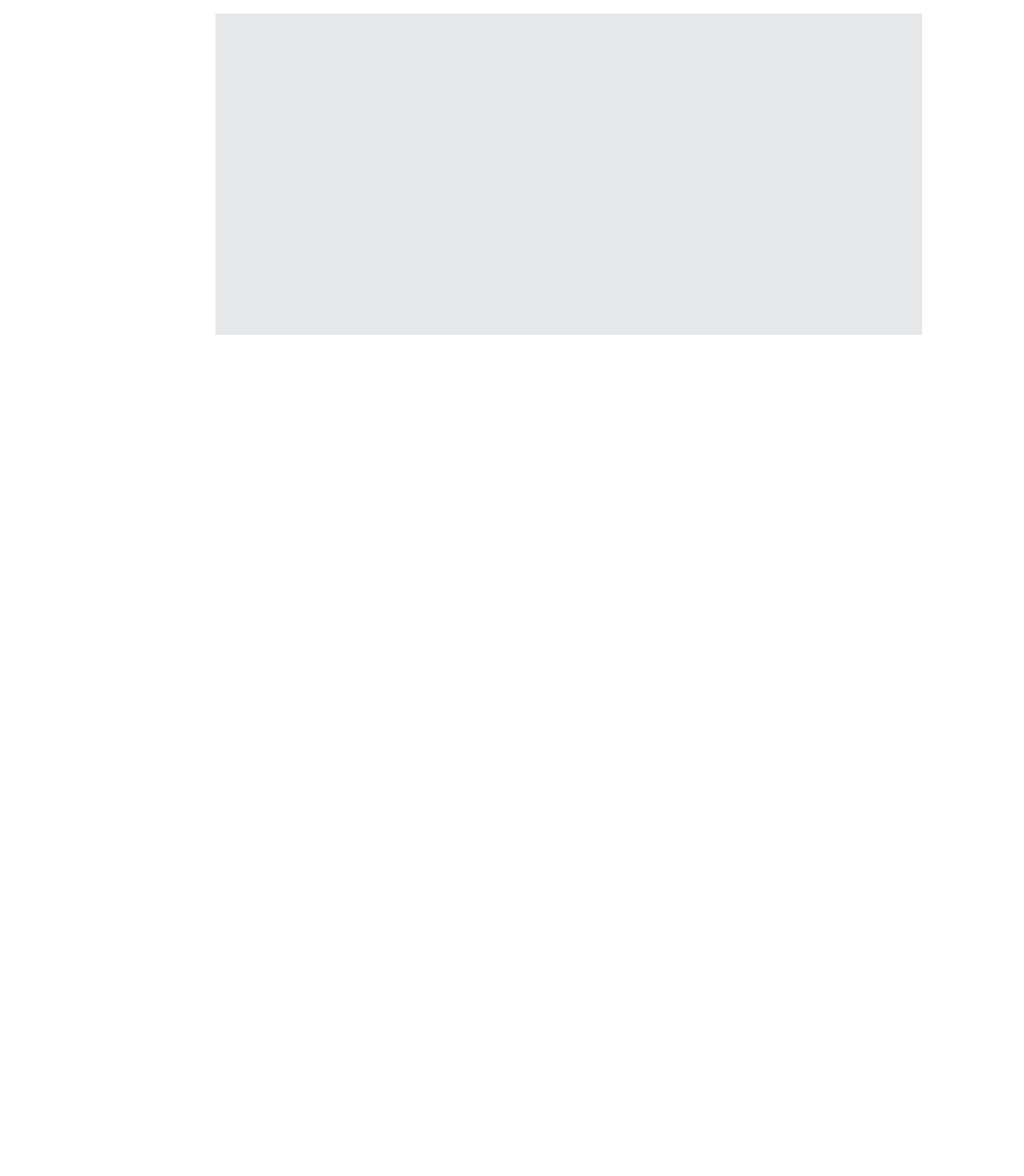
Search WWH ::

Custom Search