Java Reference
In-Depth Information
often avoid packages because they can make it harder to compile the entire project
successfully. More sophisticated Java editors such as Eclipse or NetBeans handle
packages better and usually provide a one-click button for compiling and executing
all of the packages of a project.
Protected and Default Access
In Chapter 8's discussion of encapsulation we learned about two kinds of access:
private
(visible only to one class) and
public
(visible to all classes). In general,
you should follow the convention shown in this topic of declaring all fields
private
and most methods
public
(other than internal helper methods). But Java has two
other levels of access:
•
protected
access:
Visible to this class and all of its subclasses, as well as to all classes in the same
package as this class.
•
default (package) access:
Visible to all classes in the same package as this class.
Some programmers prefer to declare their fields as
protected
when writing
classes that are part of an inheritance hierarchy. This declaration provides a relaxed
level of encapsulation, because subclasses can directly access protected fields rather
than having to go through accessor or mutator methods. For example, if a subclass
DividendStock
chose to extend the following
Stock
class, it would be able to
directly get or set its
symbol
or
shares
field values:
public class Stock {
protected String symbol;
protected int shares;
...
}
The downside is that any class in the same package can also access the fields,
which is generally discouraged. A compromise recommended by Joshua Bloch,
author of
Effective Java
, is to give subclasses access to private data through protected
methods instead of protected fields. This gives the class author the freedom to change
the implementation of the class later if necessary.
Default access is given to a field when it has no access modifier in front of its dec-
laration, such as in the initial versions of our
Point
class in Chapter 8 (prior to prop-
erly encapsulating it). A field or method with default access can be directly accessed
by any class in the same package. We generally discourage the use of default access
in most situations, since it can needlessly violate the encapsulation of the object.


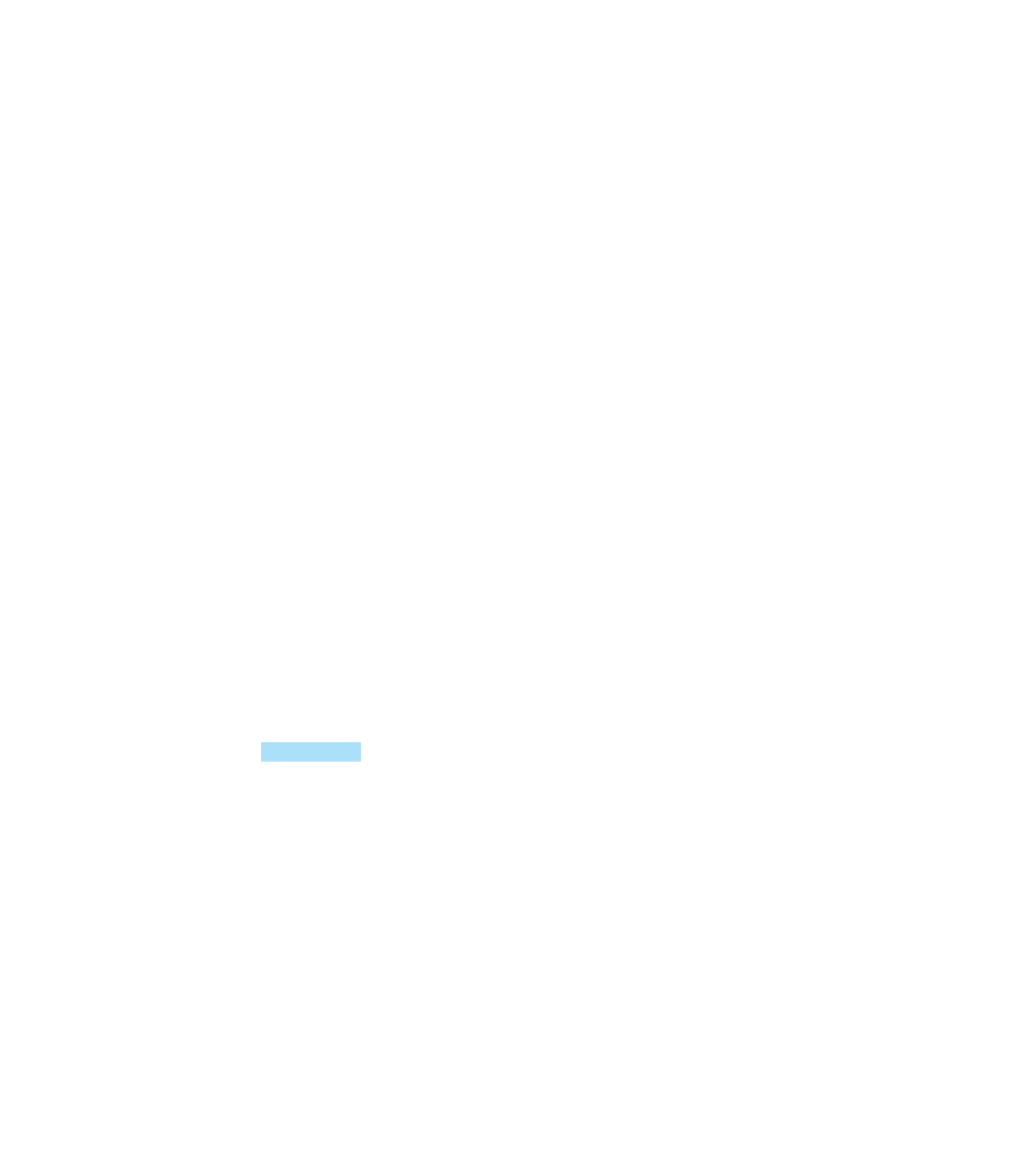