Java Reference
In-Depth Information
System.out.println();
}
private void printMirror(IntTreeNode root) {
if (root != null) {
printMirror(root.right);
System.out.print(" " + root.data);
printMirror(root.left);
}
}
10.
Many tree methods use a public/private pair because the algorithms are best imple-
mented recursively, but each recursive call must examine a progressively smaller
portion of the tree. Therefore the header of the private method generally accepts a
tree node as an additional parameter.
11.
public int size() {
return size(overallRoot);
}
private int size(IntTreeNode root) {
if (root == null) {
return 0;
} else {
return 1 + size(root.left) + size(root.right);
}
}
12.
public int min() {
if (overallRoot == null) {
throw new IllegalStateException("empty tree");
}
return min(overallRoot);
}
private int min(IntTreeNode root) {
if (root.left == null && root.right == null) {
return root.data;
} else {
int minValue = root.data;
if (root.left != null) {
minValue = Math.min(minValue, min(root.left));
}
if (root.right != null) {
minValue = Math.min(minValue, min(root.right));
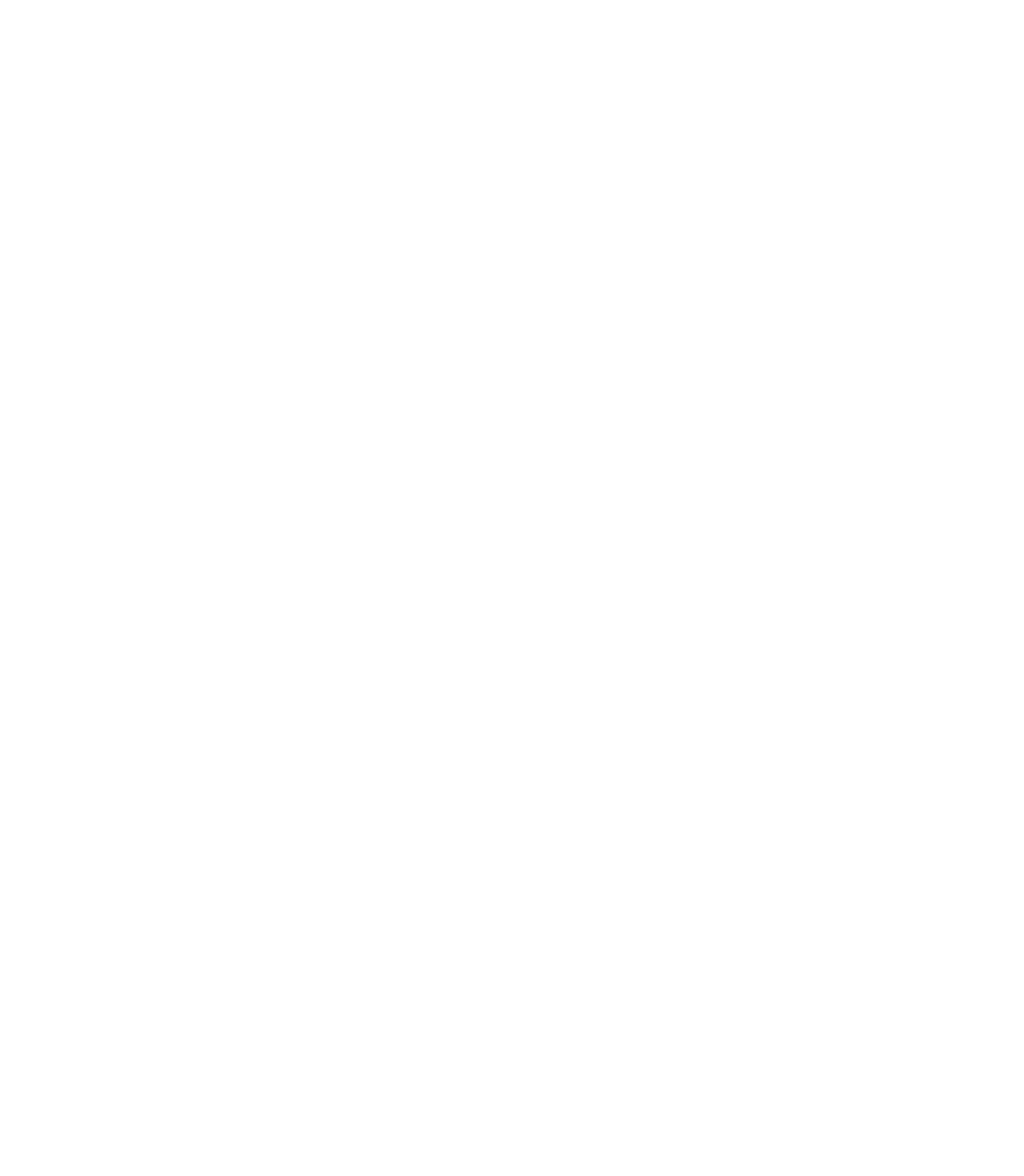
Search WWH ::

Custom Search