Java Reference
In-Depth Information
10.
for (String s : list) {
System.out.println(s.toUpperCase());
}
11.
The code throws a
ConcurrentModificationException
because it is illegal to
modify the elements of an
ArrayList
while running a for-each over it.
12.
The code doesn't compile because primitives cannot be specified as type parame-
ters for generic types. The solution is to use the “wrapper” type
Integer
instead
of
int
. Change the line declaring the
ArrayList
to the following lines:
ArrayList<Integer> numbers = new ArrayList<Integer>();
13.
The main purpose of a wrapper class is to act as a bridge between primitive values
and objects. An
Integer
is an object that holds an
int
value. Wrappers are useful
in that they allow primitive values to be stored into collections.
14.
To arrange an
ArrayList
into sorted order, call the
Collections.sort
method
on it. For example, if your
ArrayList
is stored in a variable named
list
, call the
following method:
Collections.sort(list);
In order for this to work, the type of the objects stored in the list must be
Comparable
.
15.
A natural ordering is an order for objects of a class where “lesser” objects come
before “greater” ones, as determined by a procedure called the class's comparison
function. To give your own class a natural ordering, declare it to implement the
Comparable
interface and define a comparison function for it by writing an appro-
priate
compareTo
method.
16.
(a)
n1.compareTo(n2) > 0
(b)
n3.compareTo(n1) == 0
(c)
n2.compareTo(n1) < 0
(d)
s1.compareTo(s2) < 0
(e)
s3.compareTo(s1) > 0
(f)
s2.compareTo(s2) == 0
17.
Scanner console = new Scanner(System.in);
System.out.print("Type a name: ");
String name1 = console.nextLine();
System.out.print("Type a name: ");
String name2 = console.nextLine();
if (name1.compareTo(name2) < 0) {
System.out.println(name1 + " goes before " + name2);
} else if (name1.compareTo(name2) > 0) {
System.out.println(name1 + " goes after " + name2);
} else { // equal
System.out.println(name1 + " is the same as " + name2);
}
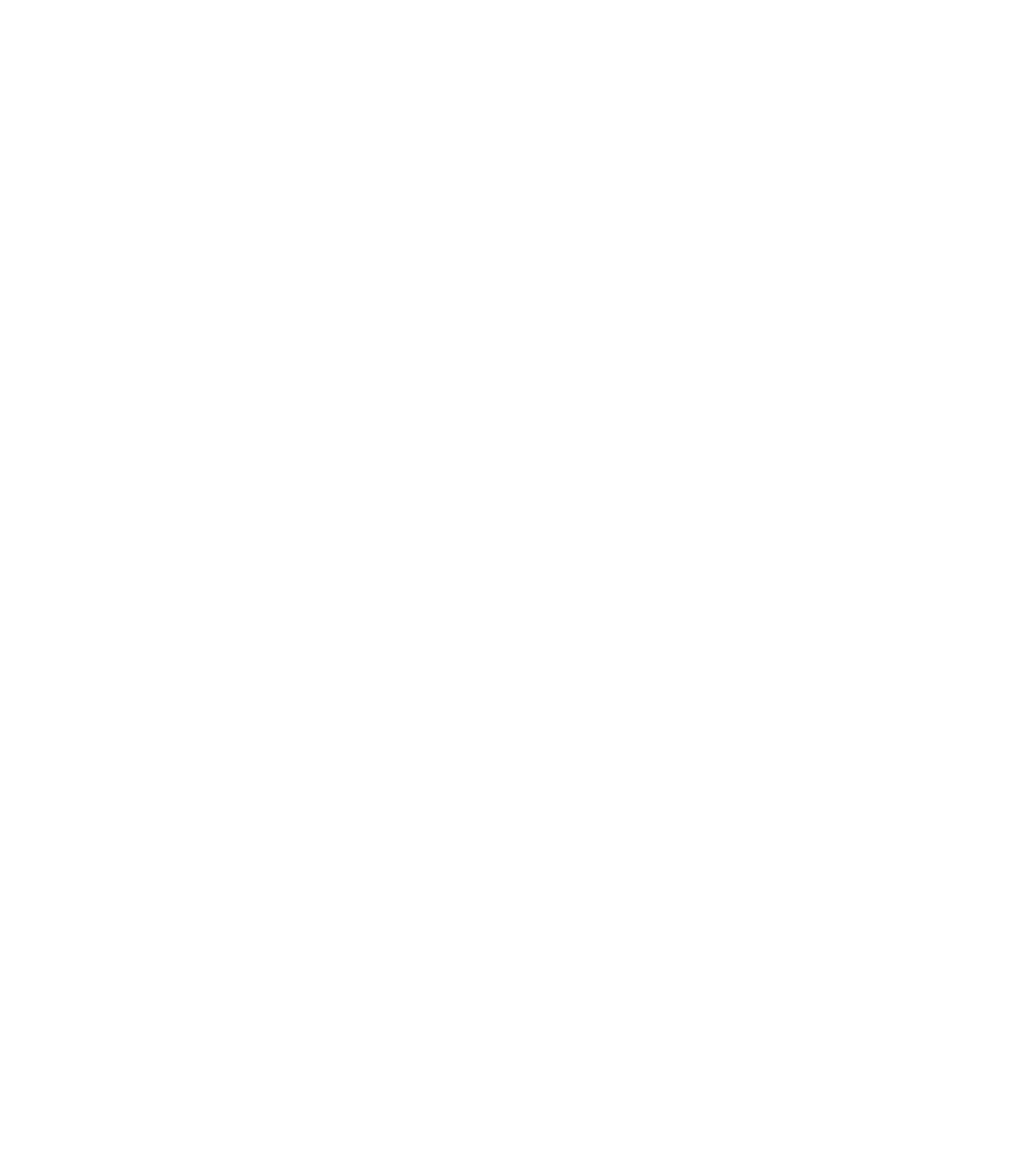
Search WWH ::

Custom Search