Java Reference
In-Depth Information
7.
What would happen if we removed the
root != null
test from the
printPreorder
method?
8.
Write a method called
printPostorder
that could be added to the
IntTree
class and that prints a postorder tra-
versal of the tree.
9.
Write a method called
printMirror
that could be added to the
IntTree
class and that prints a backward inorder
traversal of the tree. That is, for a given node, it examines the right subtree, then the node itself, then the left subtree.
Section 17.3: Common Tree Operations
10.
Why do many recursive tree methods use a public/private pair? What is the difference between the header of the
public method and that of the private method?
11.
Write a method called
size
that could be added to the
IntTree
class and that returns the total number of nodes in
the tree.
12.
Write methods called
min
and
max
that could be added to the
IntTree
class and that return the smallest and largest
values in the tree respectively. For example, if a variable called
tree
stores the values shown in Self-Check Problem
5, the call of
tree.min()
should return
-2
and the call of
tree.max()
should return
94
. If the tree is empty, the
methods should throw an
IllegalStateException
.
13.
Write a method called
countBranches
that could be added to the
IntTree
class and that returns the total number
of branch nodes in the tree. A branch node is any node that is not a leaf node. (Hint: Look at the code for
countLeaves
written in Section 17.3.)
Section 17.4: Binary Search Trees
14.
What is the difference between a regular binary tree and a binary search tree?
15.
Which of the following trees are valid binary search trees?
a.
−
5
−
1
−
7
b.
8
5
11
2
7
10
18
4
20
18
c.
42
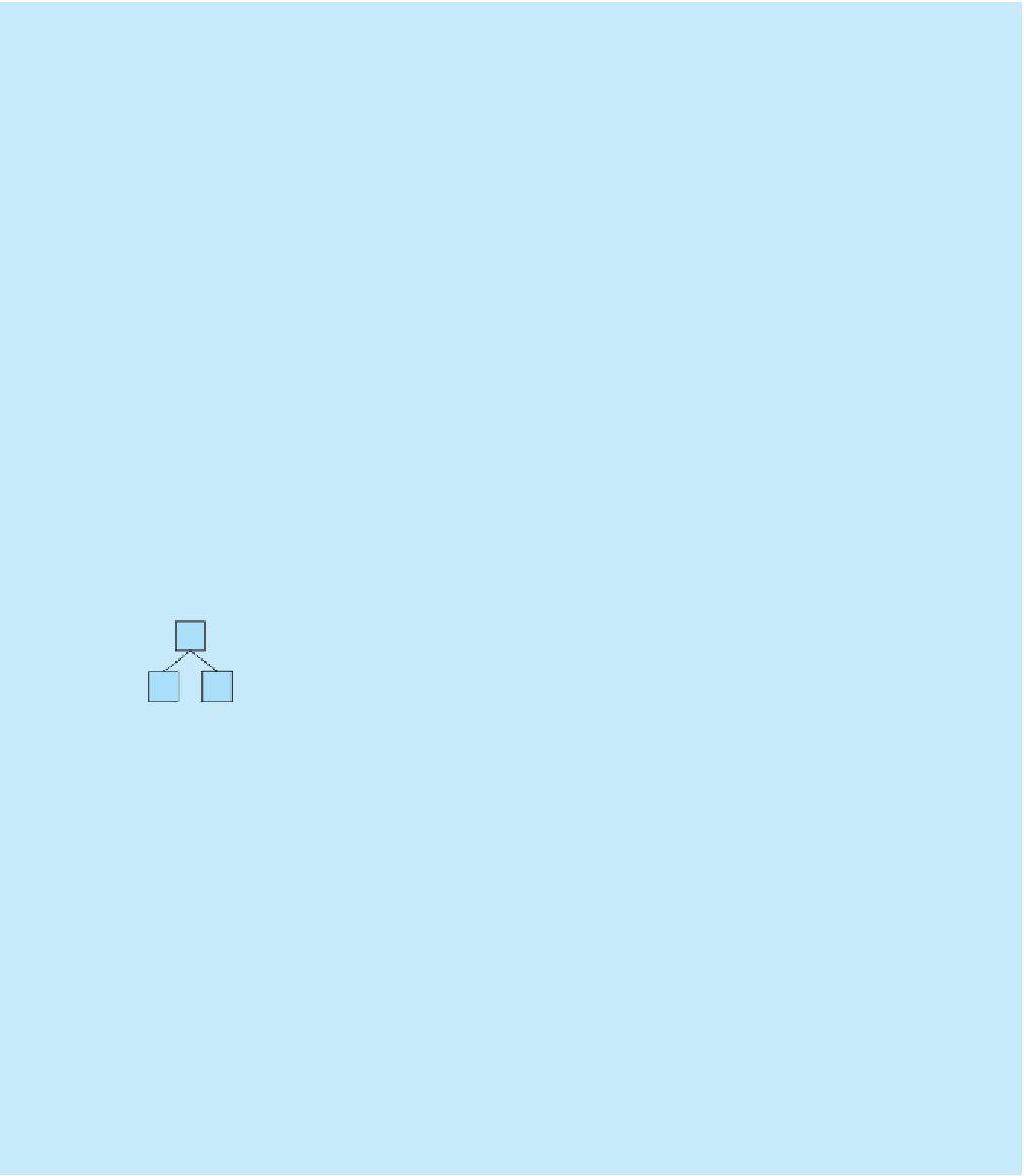
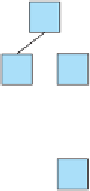
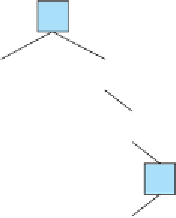
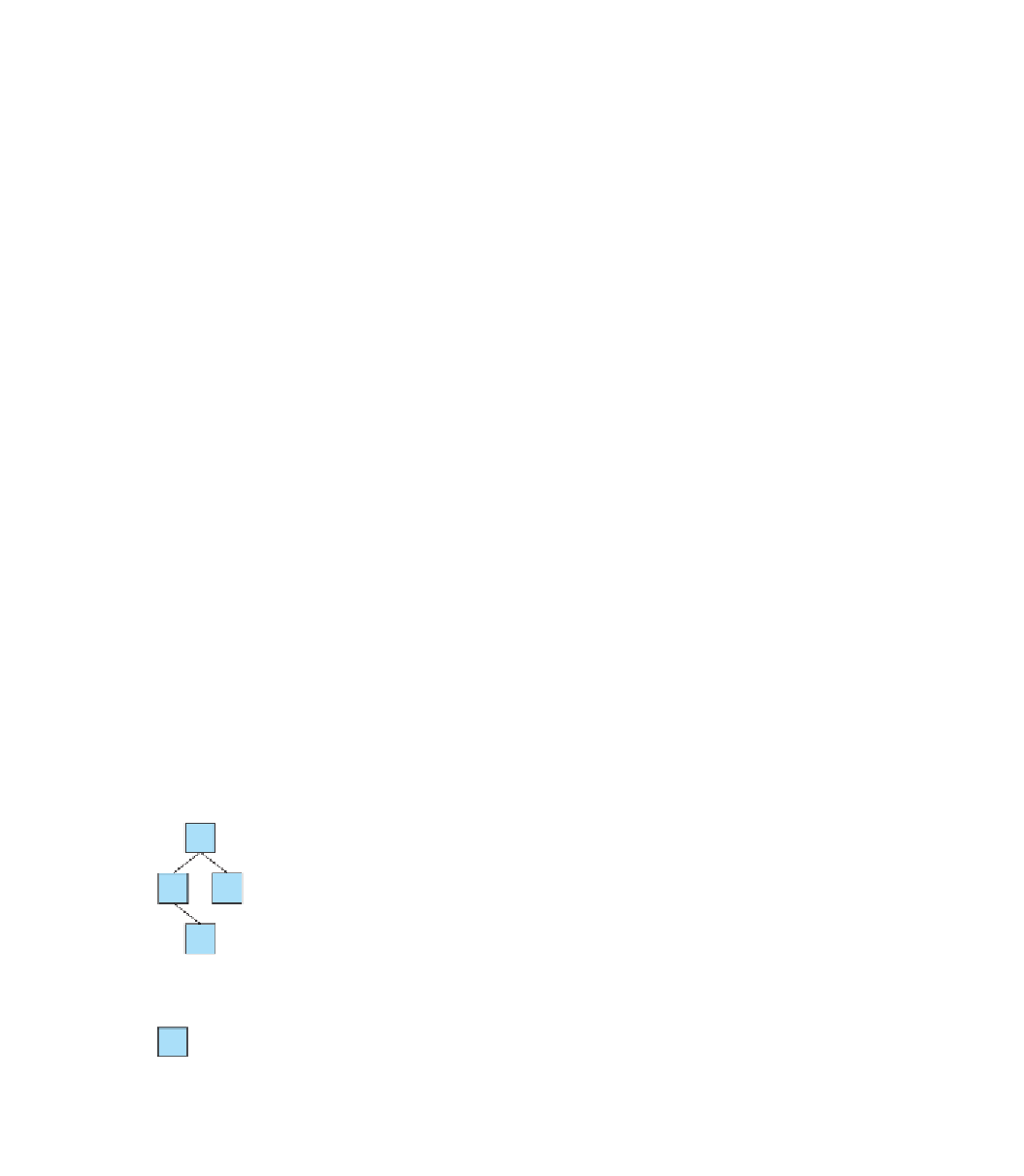
Search WWH ::

Custom Search