Java Reference
In-Depth Information
expression after the equals sign. These three assignments have the same effect as pro-
viding three declarations followed by three assignment statements:
double height;
double weight;
double bmi;
height = 70;
weight = 195;
bmi = weight / (height * height) * 703;
Another variation is to declare several variables that are all of the same type in a
single statement. The syntax is
<type> <name>, <name>, <name>, ..., <name>;
as in
double height, weight;
This example declares two different variables, both of type
double
. Notice that
the type appears just once, at the beginning of the declaration.
The final variation is a mixture of the previous two forms. You can declare multiple
variables all of the same type, and you can initialize them at the same time. For example,
you could say
double height = 70, weight = 195;
This statement declares the two
double
variables
height
and
weight
and gives
them initial values (
70
and
195
, respectively). Java even allows you to mix initializ-
ing and not initializing, as in
double height = 70, weight = 195, bmi;
This statement declares three
double
variables called
height
,
weight
, and
bmi
and provides initial values to two of them (
height
and
weight
). The variable
bmi
is
uninitialized.
Common Programming Error
Declaring the Same Variable Twice
One of the things to keep in mind as you learn is that you can declare any given
variable just once. You can assign it as many times as you like once you've
declared it, but the declaration should appear just once. Think of variable decla-
ration as being like checking into a hotel and assignment as being like going in
Continued on next page
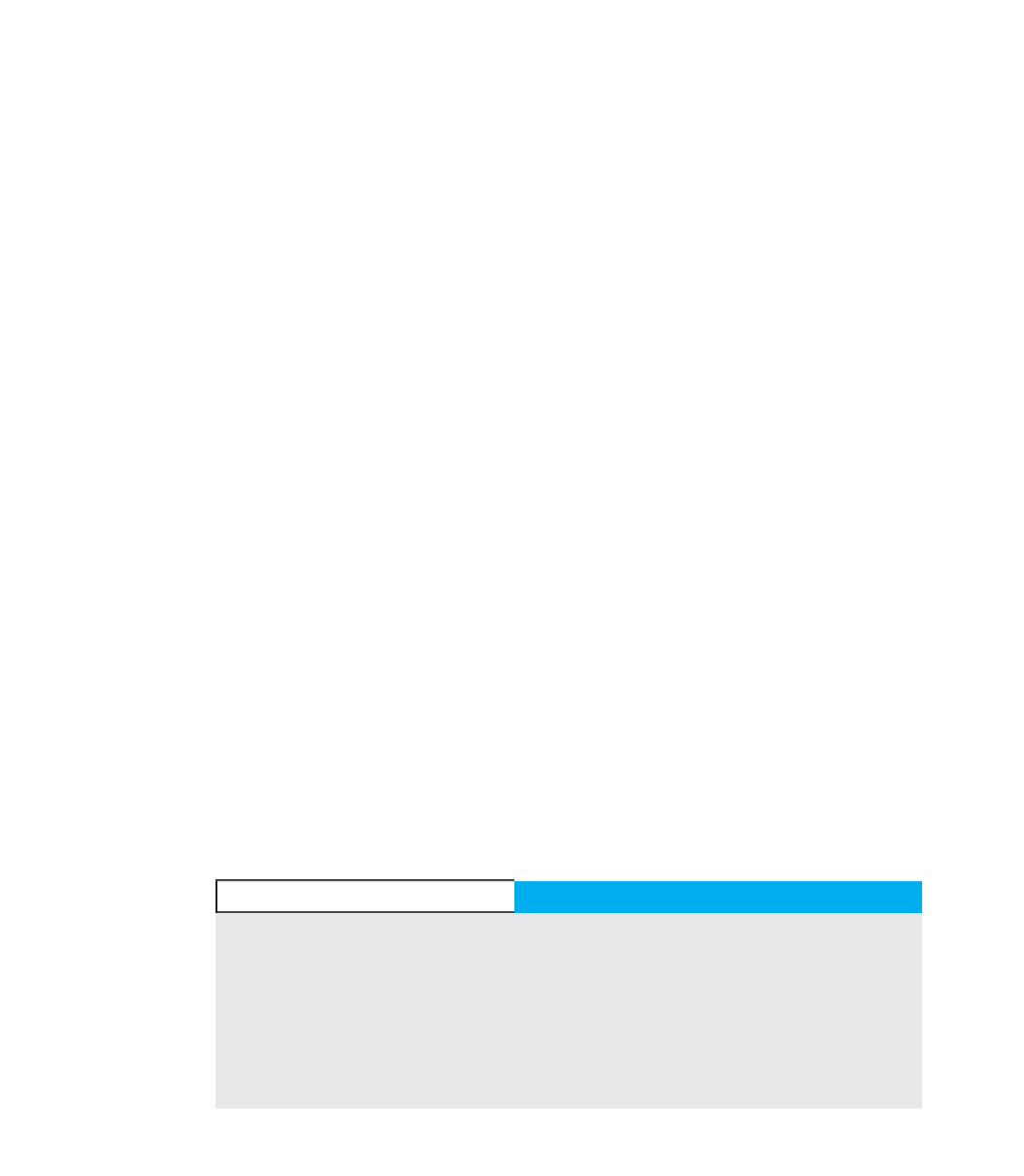
Search WWH ::

Custom Search