Java Reference
In-Depth Information
83 // node depth; prints right to left so that it looks
84 // correct when the output is rotated.
85
public void
printSideways() {
86 printSideways(overallRoot, 0);
87 }
88
89 // post: prints in reversed preorder the tree with given
90 // root, indenting each line to the given level
91
private void
printSideways(IntTreeNode root,
int
level) {
92
if
(root != null) {
93 printSideways(root.right, level + 1);
94
for
(
int
i = 0; i < level; i++) {
95 System.out.print(" ");
96 }
97 System.out.println(root.data);
98 printSideways(root.left, level + 1);
99 }
100 }
101 }
This section discusses several common binary tree operations. All of these operations
are built on top of a standard tree traversal and involve reasoning recursively about
the subtrees.
First let's write a method to find the sum of the values stored in a binary tree of
int
s.
The method header will look like this:
public int sum() {
...
}
As usual, you will need to introduce a private method that allows you to pass a
node as a parameter so that you can specify which subtree of the overall tree to work
with. The public method should call the private method, pass it the overall root, and
return the result:
public int sum() {
return sum(overallRoot);
}


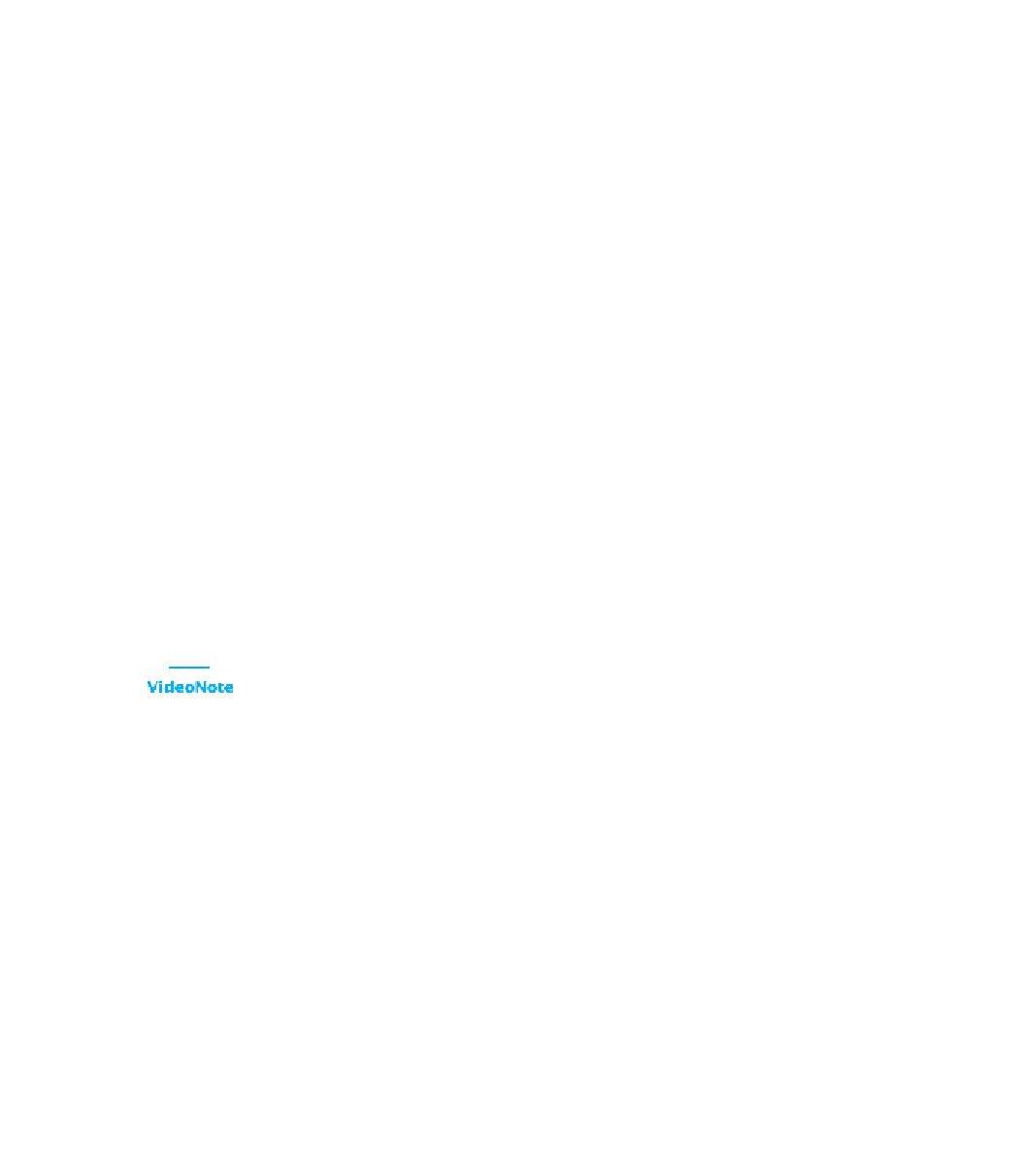
Search WWH ::

Custom Search