Java Reference
In-Depth Information
method to give a value to
overallRoot
. And instead of passing a parameter of type
IntTreeNode
, we want the method to return a value of type
IntTreeNode
(a reference
to the tree it has built). Thus, the pair of methods will look something like this:
public IntTree(int max) {
overallRoot = buildTree();
}
private IntTreeNode buildTree() {
...
}
This is a good first approximation, but it isn't right. As you learn to write code for
binary trees, you will discover that one of the most difficult tasks is figuring out the appro-
priate parameters to pass. As you practice more, you will see various patterns emerge.
One obvious parameter to include here is the value
max
that is passed to the public
method. How can the private method do its job properly if it doesn't know when to
stop? You can modify your pair to include that parameter:
public IntTree(int max) {
overallRoot = buildTree(max);
}
private IntTreeNode buildTree(int max) {
...
}
This still isn't enough information for the private method to do its job. Think of it
this way: The private method has to construct each of the different subtrees that are
involved. One call on the method will construct the overall tree. Another will con-
struct the left subtree of the overall root. Another will construct the right subtree of
the overall root. How is the method supposed to know which of these tasks to solve?
We must include an extra parameter telling the method the node number to use for
the root of the tree that it is constructing. When the method is passed the value
1
,it
will construct the overall root. When it is passed the value
2
, it will construct the left
subtree of the overall root, and so on. Including this second parameter, we end up
with the following code:
public IntTree(int max) {
overallRoot = buildTree(1, max);
}
// returns a sequential tree with n as its root unless n is
// greater than max, in which case it returns an empty tree
private IntTreeNode buildTree(int n, int max) {
...
}






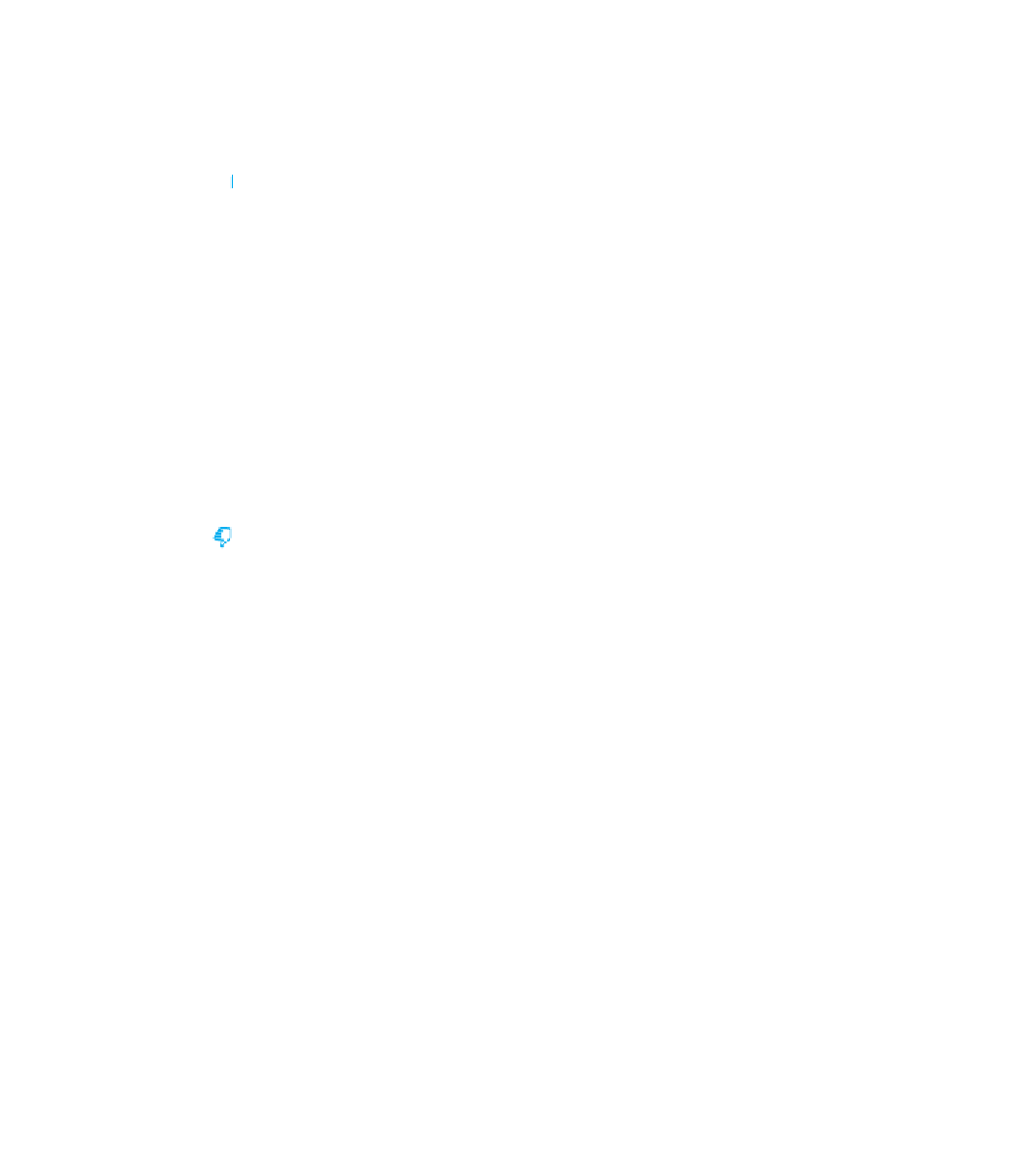

Search WWH ::

Custom Search