Java Reference
In-Depth Information
Let's now see how we can write public methods for the
IntTree
class that will
perform these traversals. Let's begin by writing a public method that will print the
preorder traversal of a tree. Your public method will look like this:
public void printPreorder() {
...
}
At this point we run into a problem that we discussed in Chapter 12. Often when
you write a recursive solution, you have to introduce a second method that we call a
helper method. When you do so, it is best to write the second method as a private
method. For these binary tree methods, it will almost always be the case that you
actually have to write a pair of methods to solve any of these problems.
The issue is that the client makes a request to print the entire tree. But to solve this
problem recursively, you need a method that works on every subtree, not just the
overall tree. In other words, you need a method that takes an
IntTreeNode
as a
parameter so that the recursive method will match the recursive structure of the tree.
That way each recursive call will be able to pass on smaller and smaller subtrees,
which allows you to get closer to reaching a base case. As a result, you will see a
common pattern in binary tree code that looks like this:
public void doSomething(<parameters>) {
doSomething(overallRoot, <parameters>);
}
private void doSomething(IntTreeNode root, <parameters>) {
...
}
Binary tree code won't always follow this pattern exactly because there might be
other actions to perform in the public method, the return type might not be
void,
or
there might be other parameters. But this general pattern will be there even with all of
these variations.
To solve the problem of printing in preorder, you need a private method that has an
IntTreeNode
parameter:
private void printPreorder(IntTreeNode root) {
...
}
You start the recursive process by passing it the overall root. You can also include
code to print text at the beginning and end of the line of output that you want to produce:
public void printPreorder() {
System.out.print("preorder:");
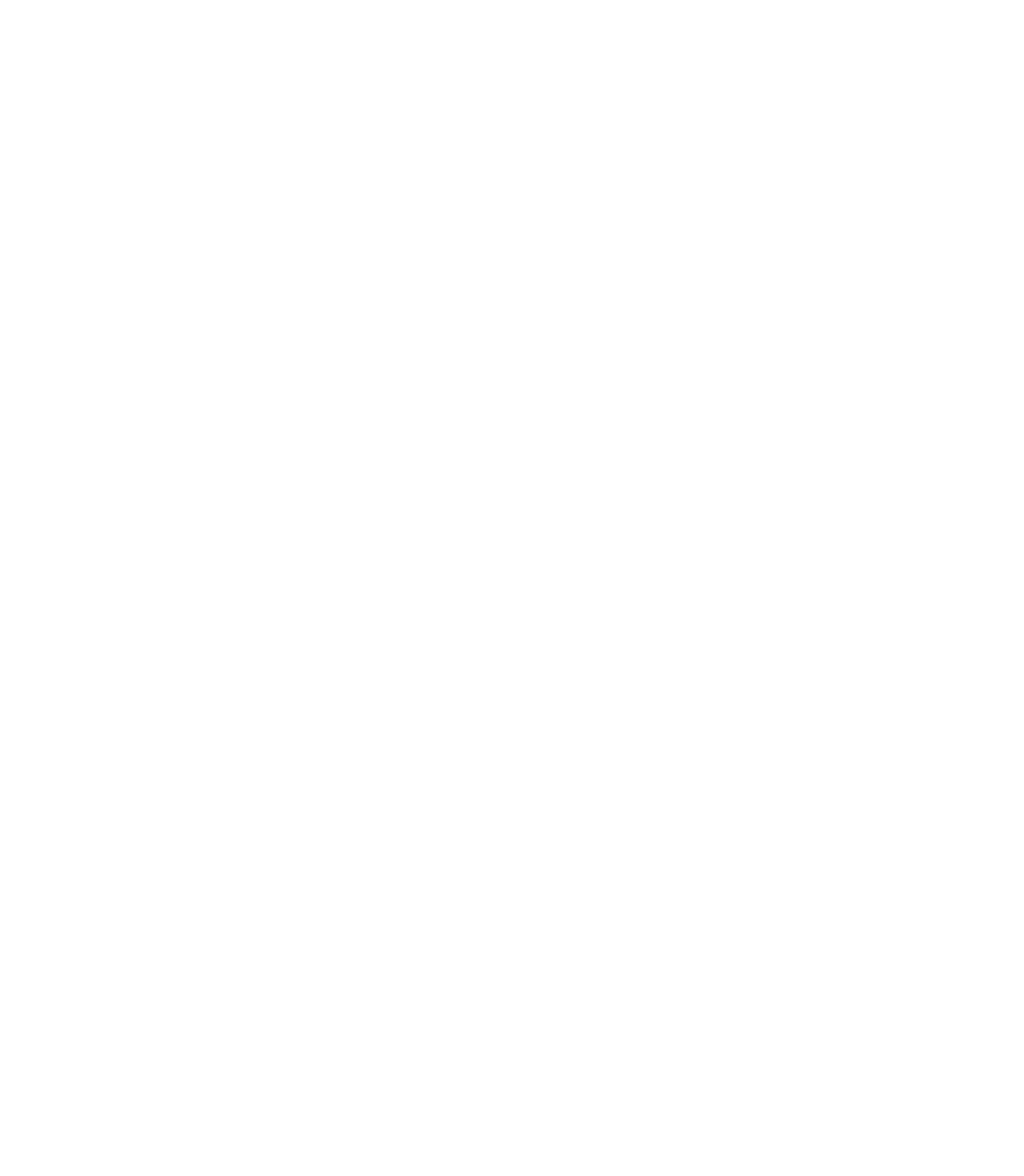
Search WWH ::

Custom Search