Java Reference
In-Depth Information
2.8.3 Comparing Object Variables
Consider the following that creates two identical, but separate, objects and stores their addresses in
a
and
b
:
Part a = new Part("Air Filter", 8.75);
Part b = new Part("Air Filter", 8.75);
Assume these statements create the situation shown in Figure
2-9
.
name:Air Filter
price: 8.75
name:Air Filter
price:8.75
2000
a
4000
b
Figure 2-9.
After creation of two identical objects
Since the objects are identical, it may come as a surprise that the following condition is
false
:
a == b
However, if you remember that
a
and
b
contain
addresses
and not objects, then we are comparing the address
in
a
(
2000
) with the address in
b
(
4000
). Since these are different, the comparison is
false
.
Two object variables would compare equal only when they contain the
same address
(in which case they point to
the same object). This could happen, for instance, when we assign one object variable to another.
We would, of course, need to know whether two
objects
are the same. That is, if
a
and
b
point to two objects, are
the
contents
of these objects the same? To do this, we must write our own method that compares the fields, one by one.
Using the class
Part
as an example, we write a method
equals
, which returns
true
if one object is identical to
another and
false
otherwise. The method can be used as follows to compare
Part
objects pointed to by
a
and
b
:
if (a.equals(b)) ...
The method simply checks whether the
name
fields and the
price
fields of the two objects are the same. Since
the
name
fields are
String
objects, we call the
equals
method of the
String
class to compare them.
1
public boolean equals(Part p) {
return name.equals(p.name) && (price == p.price);
}
In the method, the variables
name
and
price
(without being qualified) refer to the fields of the object via which
the method is invoked. Suppose we had used the following expression:
a.equals(b)
1
There is no conflict in using the same name
equals
to compare
Part
s and
String
s. If
equals
is invoked via a
Part
object, then
the
equals
method from the
Part
class is used. If
equals
is invoked via a
String
object, then the
equals
method from the
String
class is used.
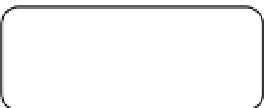





Search WWH ::

Custom Search