Java Reference
In-Depth Information
public void push(int n) {
if (top == MaxStack - 1) {
System.out.printf("\nStack Overflow\n");
System.exit(1);
}
++top;
ST[top] = n;
} //end push
To illustrate, after the numbers 36, 15, 52, and 23 have been pushed onto
S
, our picture in memory looks like
Figure
4-2
.
S
3
top
36
15
52
23
ST
0
1
2
3
4
5
Figure 4-2.
Stack view after pushing 36, 15, 52, and 23
Finally, to pop an item off the stack, we return the value in location
top
and decrease
top
by
1
. The basic idea is
as follows:
set hold to ST[top]
subtract 1 from top
return hold
Again, we must guard against trying to take something off an empty stack. What should we do if the stack is empty
and
pop
is called? We could simply report an error and halt the program. However, it might be better to return some
“rogue” value, indicating that the stack is empty. We take the latter approach in our function
pop
. Here is the instance
method
pop
in the
Stack
class:
public int pop() {
if (this.empty())return RogueValue; //a symbolic constant
int hold = ST[top];
--top;
return hold;
}
Note that even though we have written
pop
to do something reasonable if it is called and the stack is empty, it is
better if the programmer establishes that the stack is
not
empty (using the
empty
function)
before
calling
pop
.
Given the class
Stack
, we can now write Program P4.1, which reads some numbers, terminated by
0
, and prints
them in reverse order. Note that the word
public
is dropped from the class
Stack
in order to store the entire program
in one file,
StackTest.java
.
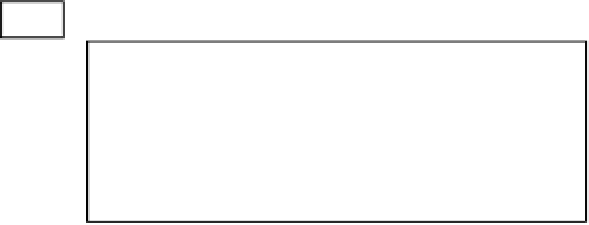





Search WWH ::

Custom Search