Java Reference
In-Depth Information
To demonstrate the proper way to create a Swing-based program, Listing 2-1 shows the
source for a selectable button.
Listing 2-1.
Swing Application Framework
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ButtonSample {
public static void main(String args[]) {
Runnable runner = new Runnable() {
public void run() {
JFrame frame = new JFrame("Button Sample");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JButton button = new JButton("Select Me");
// Define ActionListener
ActionListener actionListener = new ActionListener() {
public void actionPerformed(ActionEvent actionEvent) {
System.out.println("I was selected.");
}
};
// Attach listeners
button.addActionListener(actionListener);
frame.add(button, BorderLayout.SOUTH);
frame.setSize(300, 100);
frame.setVisible(true);
}
};
EventQueue.invokeLater(runner);
}
}
This code produces the button shown in Figure 2-3.
Figure 2-3.
Button sample
First, let's look at the
invokeLater()
method. It requires a
Runnable
object as its argument.
You just create a
Runnable
object and pass it along to the
invokeLater()
method. Some time
after the current event dispatching is done, this
Runnable
object will execute.
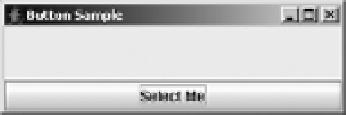