Java Reference
In-Depth Information
Mixed-type comparisons are always confusing because the system is forced to promote one operand
to match the type of the other. The conversion is invisible and may not yield the results that you
expect. There are several ways to avoid mixed-type comparisons. To pursue our fruit metaphor, you
can choose to compare apples to apples or oranges to oranges. You can cast the
int
to a
byte
, after
which you will be comparing one
byte
value to another:
if (b == (byte)0x90)
System.out.println("Joy!");
Alternatively, you can convert the
byte
to an
int
, suppressing sign extension with a mask, after
which you will be comparing one
int
value to another:
if ((b & 0xff) == 0x90)
System.out.println("Joy!");
Either of these solutions works, but the best way to avoid this kind of problem is to move the
constant value outside the loop and into a constant declaration.
Here is a first attempt:
public class BigDelight {
private static final byte TARGET = 0x90; // Broken!
public static void main(String[] args) {
for (byte b = Byte.MIN_VALUE; b < Byte.MAX_VALUE; b++)
if (b == TARGET)
System.out.print("Joy!");
}
}
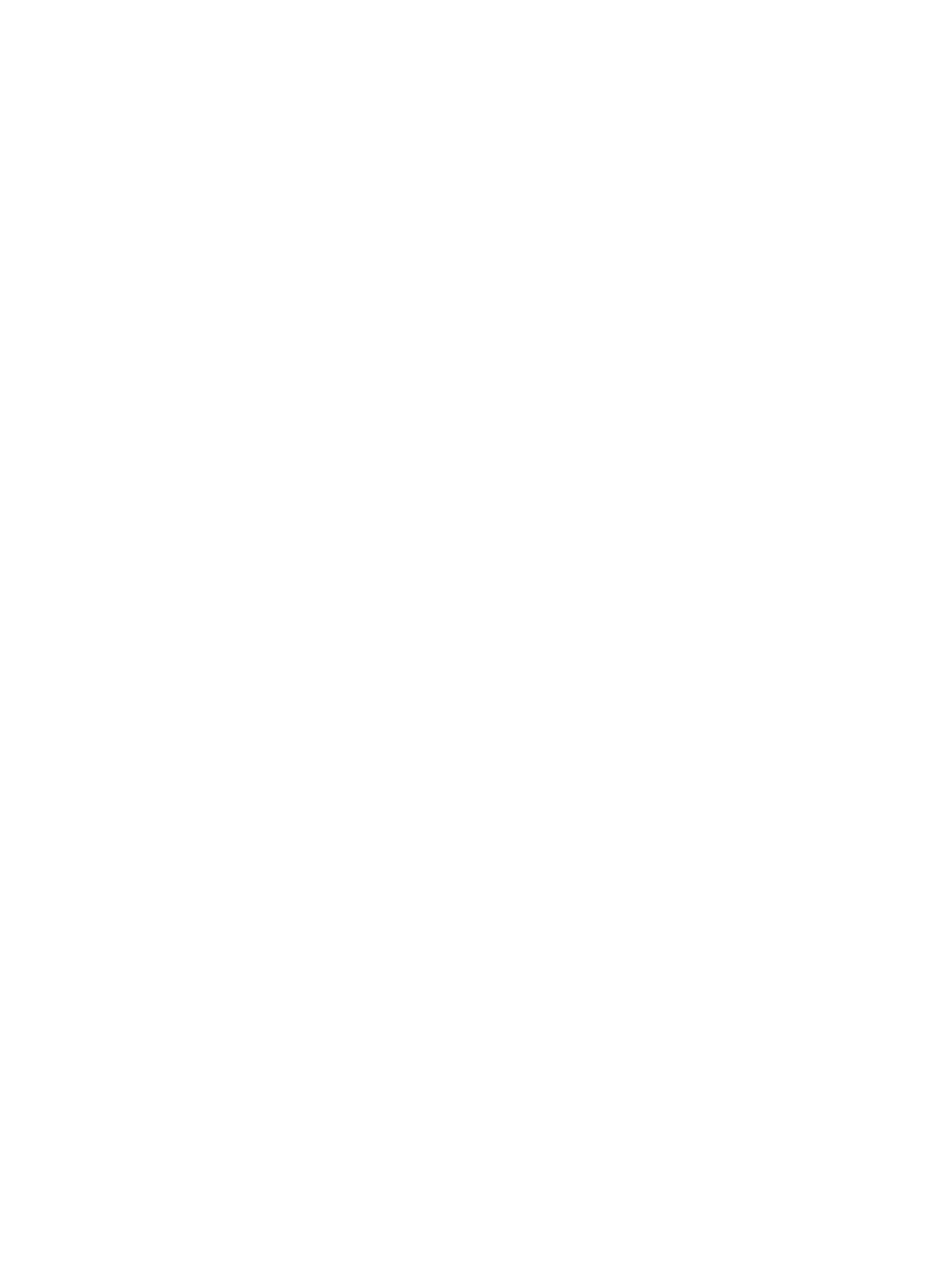
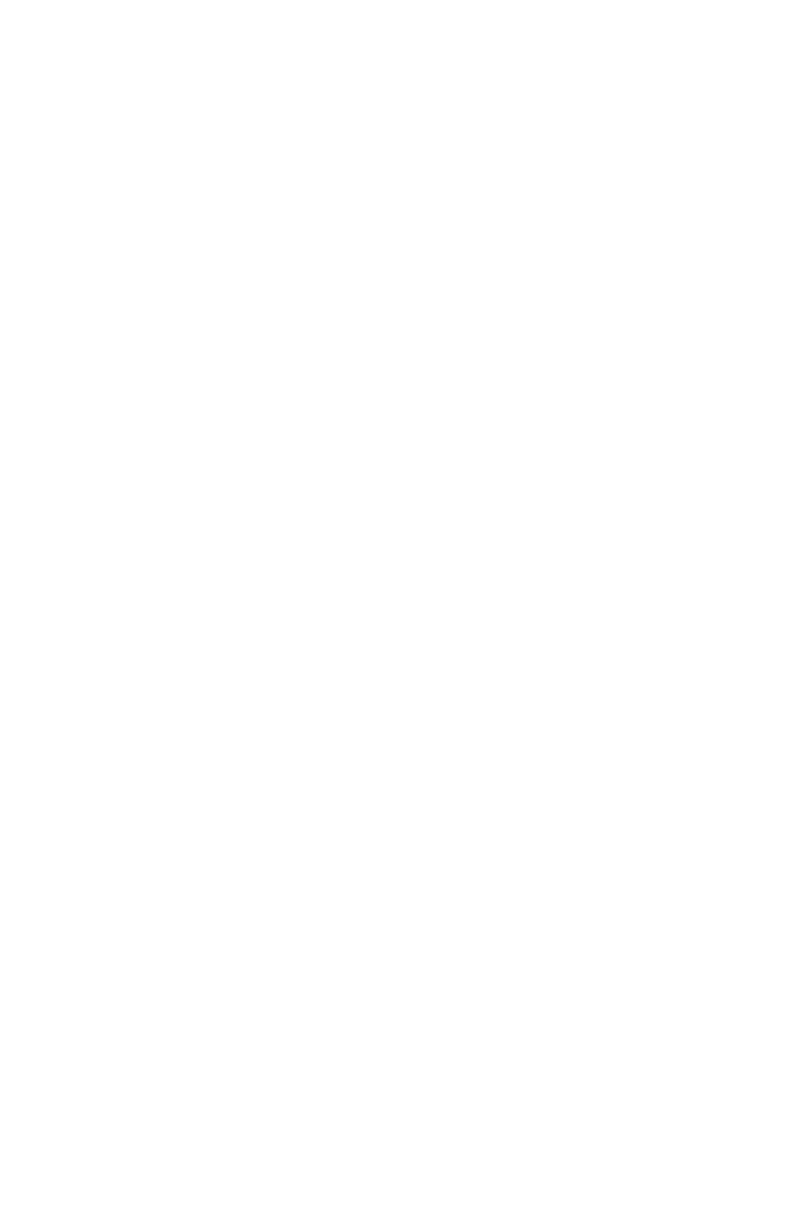



Search WWH ::

Custom Search