Java Reference
In-Depth Information
rather than sign extension. The resulting
int
value is
65535
, which is just what the program prints.
Although there is a simple rule describing the sign extension behavior of widening primitive
conversions between signed and unsigned integral types, it is best not to write programs that depend
on it. If you are doing a widening conversion to or from a
char
, which is the only unsigned integral
type, it is best to make your intentions explicit.
If you are converting from a
char
value
c
to a wider type and you don't want sign extension,
consider using a bit mask for clarity, even though it isn't required:
int i = c & 0xffff;
Alternatively, write a comment describing the behavior of the conversion:
int i = c; // Sign extension is not performed
If you are converting from a
char
value
c
to a wider integral type and you want sign extension, cast
the
char
to a
short
, which is the same width as a
char
but signed. Given the subtlety of this code,
you should also write a comment:
int i = (short) c; // Cast causes sign extension
If you are converting from a
byte
value
b
to a
char
and you don't want sign extension, you must use
a bit mask to suppress it. This is a common idiom, so no comment is necessary:
char c = (char) (b & 0xff);
If you are converting from a
byte
to a
char
and you want sign extension, write a comment:
char c = (char) b; // Sign extension is performed
The lesson is simple:
If you can't tell what a program does by looking at it, it probably doesn't
do what you want
. Strive for clarity. Although a simple rule describes the sign extension behavior
of widening conversions involving signed and unsigned integral types, most programmers don't
know it. If your program depends on it, make your intentions clear.
< Day Day Up >

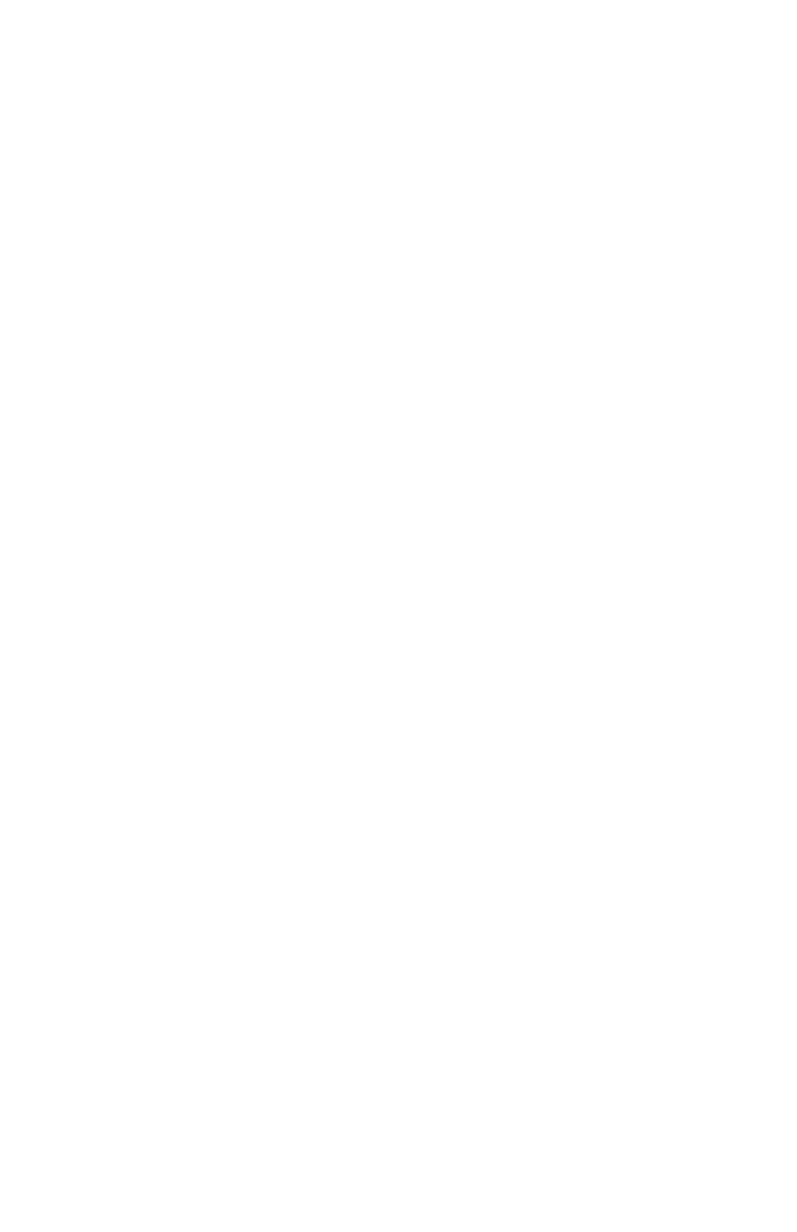









Search WWH ::

Custom Search