Java Reference
In-Depth Information
force the correct order of evaluation:
if (ms %
(
60 * 1000
)
== 0)
minutes++;
There is, however, a much better way to fix the program.
Replace all magic numbers with
appropriately named constants
:
public class Clock {
private static final int MS_PER_HOUR = 60 * 60 * 1000;
private static final int MS_PER_MINUTE = 60 * 1000;
public static void main(String[] args) {
int minutes = 0;
for (int ms = 0; ms < MS_PER_HOUR; ms++)
if (ms % MS_PER_MINUTE == 0)
minutes++;
System.out.println(minutes);
}
}
The expression
ms % 60*1000
in the original program was laid out to fool you into thinking that
multiplication has higher precedence than remainder. The compiler, however, ignores this white
space, so
never use spacing to express grouping; use parentheses
. Spacing can be deceptive, but
parentheses never lie.
< Day Day Up >
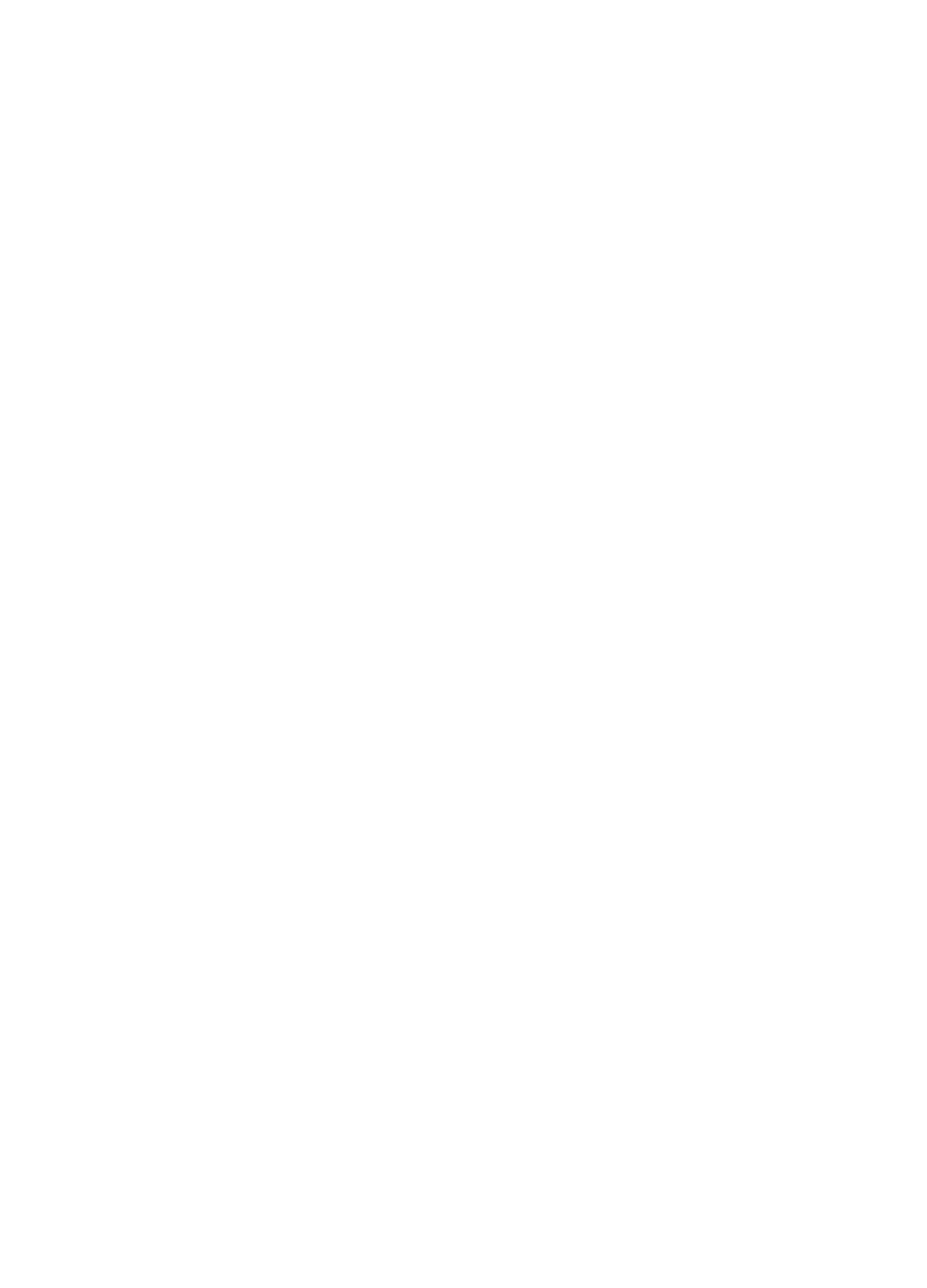
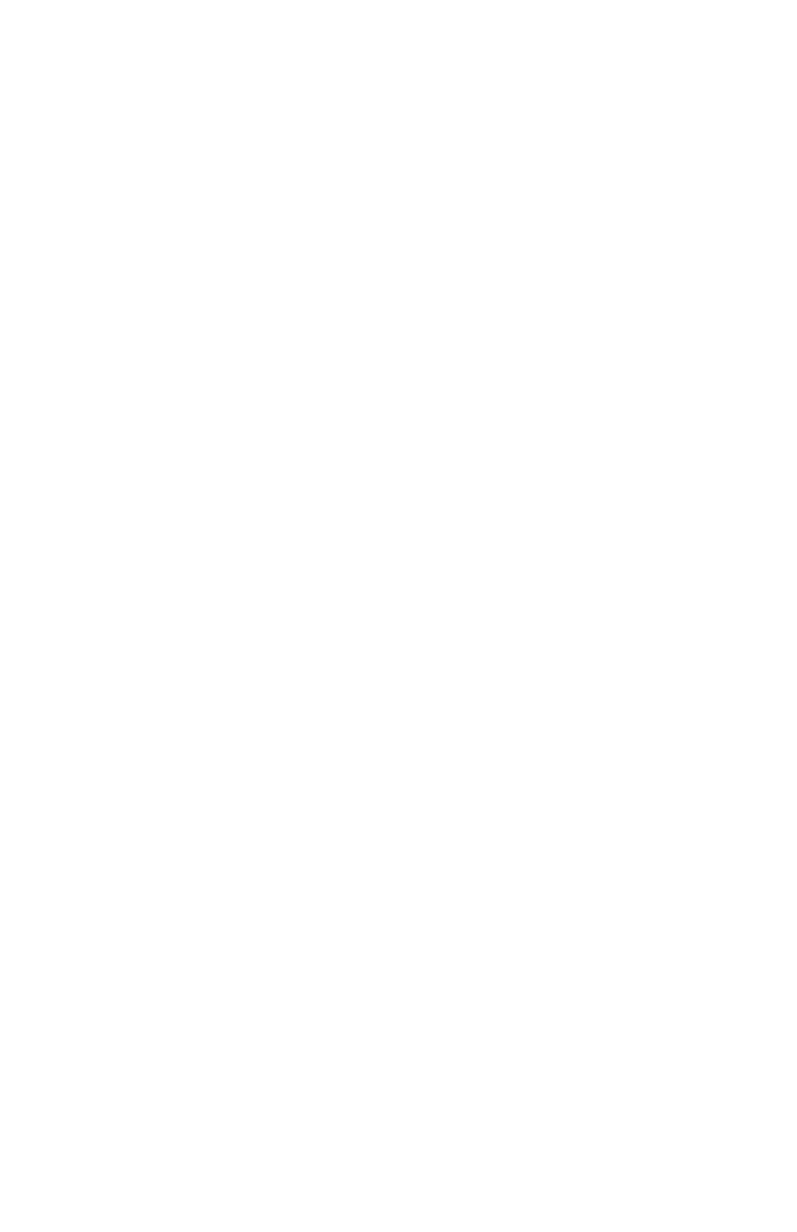









Search WWH ::

Custom Search