Graphics Reference
In-Depth Information
Standard Abstract Data Types:
integer
,
real
,
string
, etc.,
array
,
list
,
tree
,
graph
, etc.
integer array
,
real list
, etc.
These are defined by their
operations
(not some lower level data structure).
At lower levels, to describe
implementations
, there is also a
record
type that
combines known data types to produce other types. For example,
employee
=
record
string
name;
integer
age;
.
.
end
;
employee
e;
Fields of a record are referenced using the standard dot notation, as in “e.name”.
Alternatively, we shall use the capitalized field name as an operator to access the
field. For example, Name(e) will be used to denote e.name.
All pointer variables will end in “Ptr” and we shall sometimes use the convention
that if <z>Ptr is a declared pointer variable, then <z> is the item to which the
pointer points.
Pointers are often implementation artifacts, but if really needed in an abstract
program, we use operations such as PointerTo, ValueOf, SetValueOf, and Pointer-
ToFirst, as in
integer
i, k;
integer pointer
iPtr;
integer list
L;
iPtr := PointerTo (i);
k := ValueOf (iPtr);
SetValueOf (iPtr,7);
iPtr := PointerToFirst (L);
If Ptr is a pointer to a record, as in
employee pointer
Ptr;
we use the notation PtrÆage to denote the “age” field of the record pointed to by
Ptr. For example,
Ptr
Æ
age := 45;
i := Ptr
Æ
age;
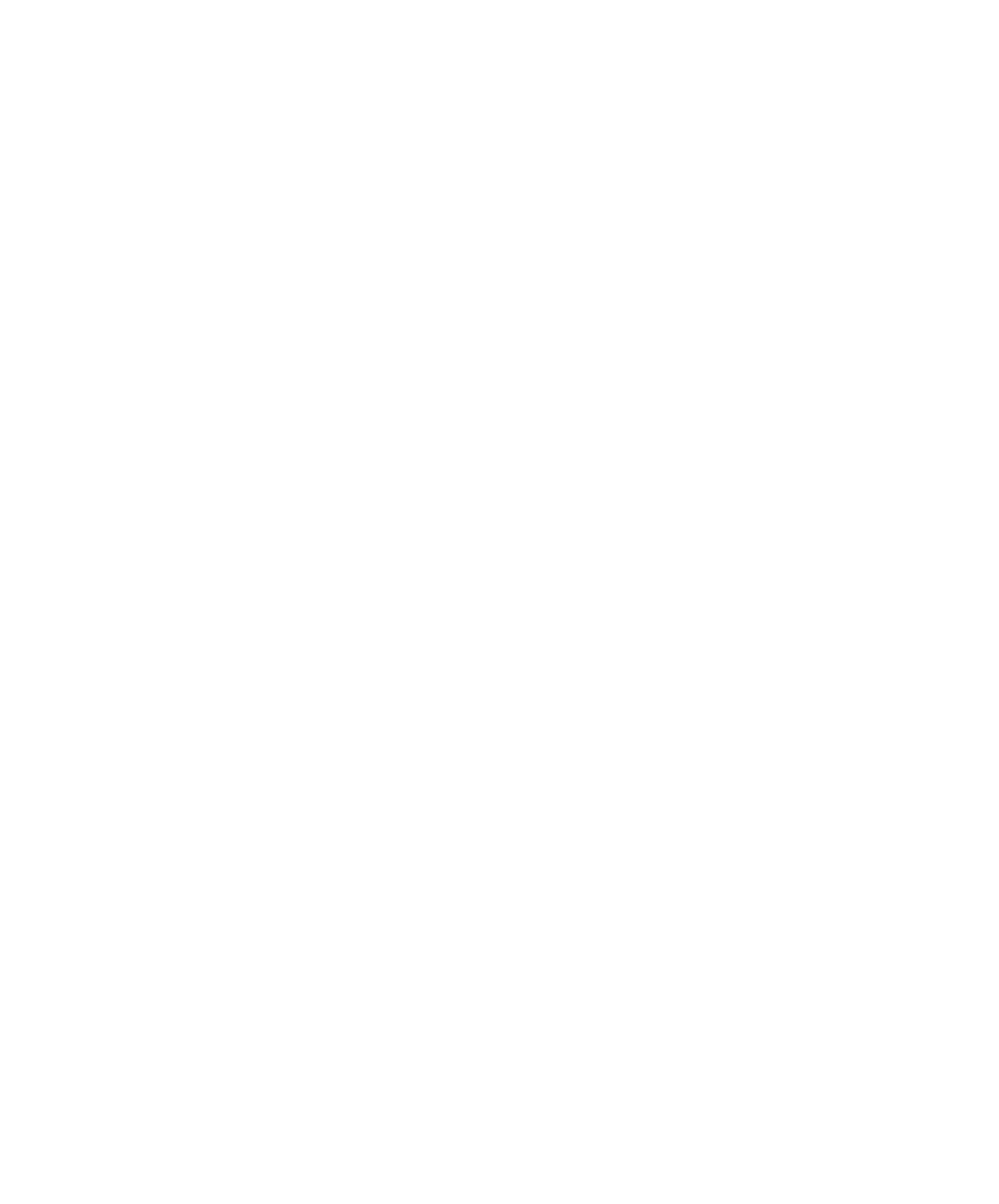