Java Reference
In-Depth Information
first.push(9);
IntegerStack second = first.clone();
With the default
clone
method, the data in memory will look something
like this:
Now consider what happens when future code invokes
first.pop()
, fol-
lowed by
first.push(17)
. The top element in the stack
first
will change
from
9
to
17
, which is expected. The programmer will probably be sur-
prised, however, to see that the top element of
second
will
also
change
to
17
because there is only one array that is shared by the two stacks.
The solution is to override
clone
to make a copy of the array:
public IntegerStack clone() {
try {
IntegerStack nObj = (IntegerStack) super.clone();
nObj.buffer = buffer.clone();
return nObj;
} catch (CloneNotSupportedException e) {
// Cannot happen -- we support
// clone, and so do arrays
throw new InternalError(e.toString());
}
}
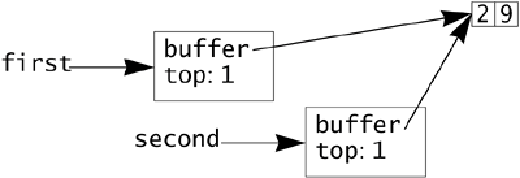