Java Reference
In-Depth Information
OPEN INPUT CUSTOMER -FILE.
MOVE 300 TO WW-CUSTOMER-NUMBER.
READ CUSTOMER -FILE.
. CLOSE CUSTOMER -FILE.
The preceding program reads the 300th record of the customer file.
RandomAccessFile
instances are constructed by passing two parameters. The
first parameter is either a string representing a file pathname or a
File
instance. The
second parameter is also a
String
parameter and is used to indicate the manner in
which the file is to be opened. If the second parameter is
r
, it means that the file is
to be opened in read-only mode and the write methods of the class will fail with
IOExceptions
. If the second parameter is
rw
, the file is opened in read-write mode.
In addition, if the file does not exist, it will be created. (There are also
rwd
and
rws
parameters, which are asynchronous versions of these modes.) Once you have
opened the file with the constructor, you can process it either sequentially or ran-
domly. To process it sequentially, you simply use the various read and write meth-
ods available from the implemented interfaces to write bytes, strings, or any of the
Java primitive variable types.
To process a file randomly, you need to understand the concept of a file
pointer. A file pointer is simply the current byte position in the file from the be-
ginning of the file. When you open the file, you are at its start and the current po-
sition is 0. Every reading or writing operation you perform resets this position. If
you read 5 bytes, the position is 4. If you then write 2 bytes, the position is 6. The
first byte of the file is byte 0. If you lose track of where you are positioned in the file,
you can call the
getFilePointer()
method to tell you the location.
You use the
seek()
method to perform random access. The
seek()
method
takes a long number as its argument. It repositions the file pointer without per-
forming any reading or writing. You can leap from the beginning of the file to the
last byte of the file simply by repositioning the file pointer with the
seek()
method.
To simulate relative record input/output, you add the concept of a record. As
long as the records are fixed length, you can easily translate from a record pointer
to a file pointer by subtracting one from the record number (if you consider the
first record number one, not zero) and multiplying by the record length.
Putting it all together, this is how you would process the customer file with Java:
// Set the record length and number field.
int recLen = 175;
int recNum = 300;
// Open the file.
RandomAccessFile file = new RandomAccessFile("test.fil", "r");
// Seek to the 300th record.
file.seek((recNum - 1) + recLen);
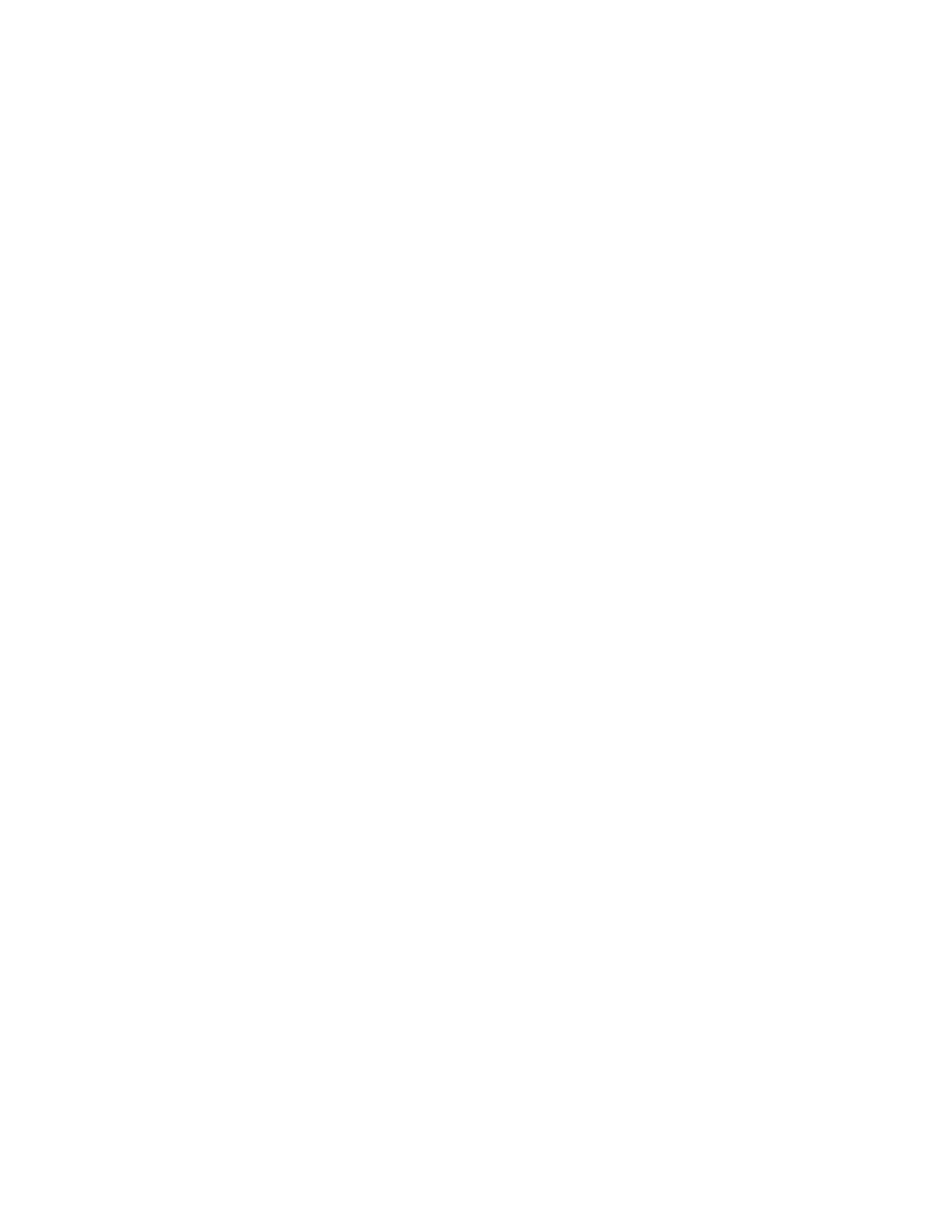