Java Reference
In-Depth Information
An object that needs to clean up resources as it is deleted can declare a
finalize()
method (in reality it needs to override the
finalize()
method in the base
java.lang.Object
class). The garbage collector will call this method before it deletes
the object.
By default, there is no guarantee that the
finalize
method will be called at all!
The Java runtime process could exit without first destroying this object. The only
guarantee is that if the object is about to be garbage collected, then this method
will be called first. There is no guarantee that this object will be garbage collected
before the Java runtime process exits. There is also no guarantee on which thread
will call
finalize()
.
In the database connection example, the runtime process may terminate before
the object's
finalize()
method is called and the database connection is properly
closed. The database engine will likely detect this event as an application failure and
will roll back any incomplete transactions.
Keep this behavior in mind, and code
finalize
methods defensively. That is,
you should only place process-level housekeeping or clean up code (such as mem-
ory management functions) in
finalize
methods. Do not assume that any code in
a
finalize
method will always or ever be performed.
You can also force the garbage collector to run by explicitly calling the
System.gc()
method.
And as a last issue to be aware of,
finalize()
methods do not automatically call
finalize()
methods in a class's superclass. This is unlike the behavior of construc-
tors, where the superclass's constructor is automatically called. Nested
finalize()
calls must be explicitly coded, as shown here:
protected void finalize() throws Throwable {
super.finalize();
}
E
XERCISES
: J
AVA
'
S
E
XCEPTIONS AND
T
HREADS
In this exercise, you are going to create some threads that simply display some mes-
sages, sleep, and eventually complete execution.
1. Using a text editor, create a file named MsgThread.java. Add these lines:
public class MsgThread extends Thread {
private volatile Boolean timeToStop = false;
public MsgThread () {
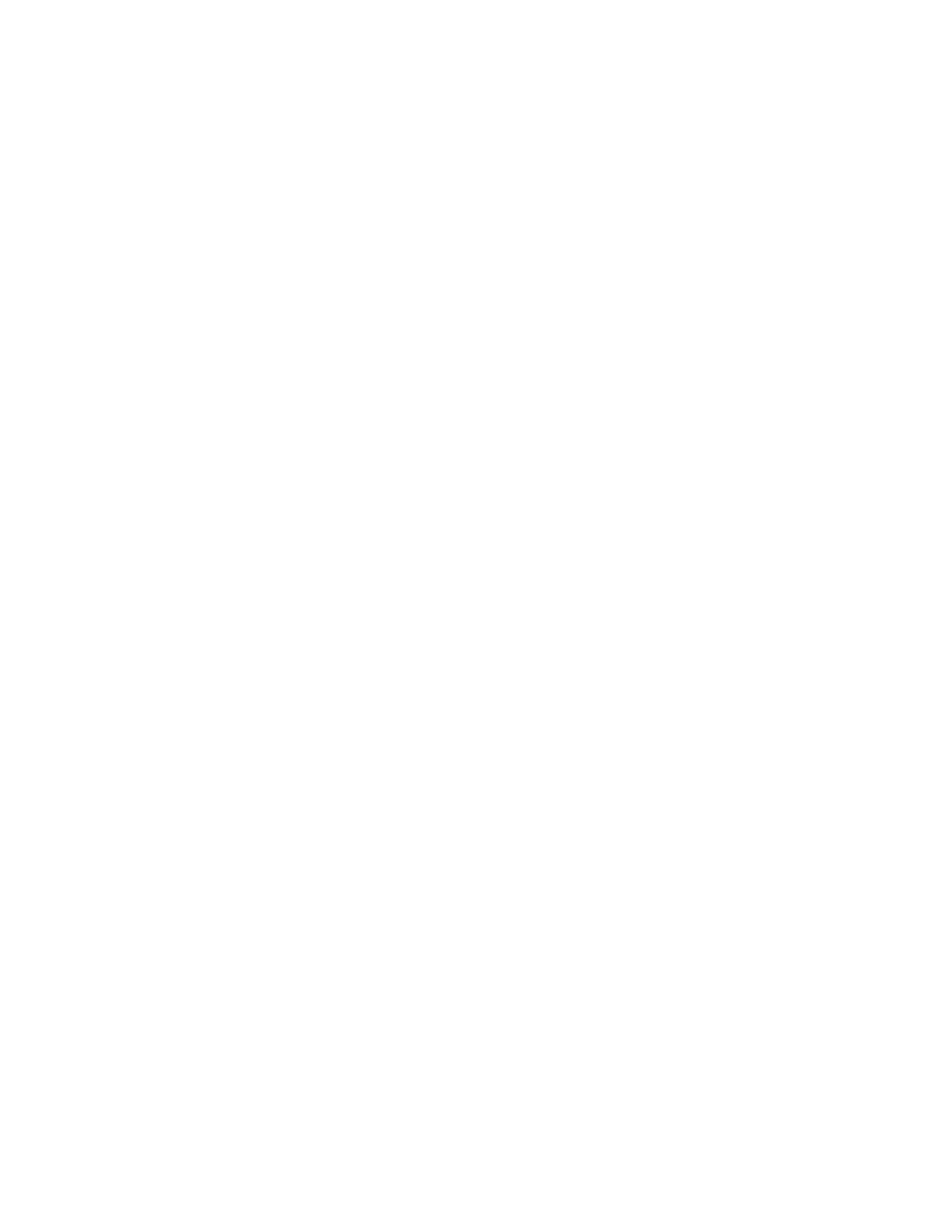