Java Reference
In-Depth Information
Inside a traditional process, there is only one thread of execution, that is, only
one statement can be performed at a time. When a statement is complete, the next
statement executes. The developer can control the sequence of statements, but
there is no way for the developer to have two statements in a program execute at the
same time.
In contrast, Java defines a multithreaded execution model. This model allows
any number of instruction streams or lines of execution inside a single process to
execute simultaneously. That is, your program can have many threads of execution
accomplishing work at the same time. A thread is any asynchronous subprocess in-
side your main program process.
Java threads allow you to construct applications that do not wait for a function
to complete before executing another function. Perhaps your program contains an
Account
object, which reads transactions from a database based on an end user's re-
quest. At the same time that this function is executing, you may need to perform an
interrupt()
method on a user interface class, which checks to see if the user wants
to cancel the request. Without simultaneous threads, you would have to wait for the
Account
object to complete its work before you could check the user interface class.
As a result, the user would not be able to cancel the request until the request was
complete!
I
NHERITING FROM
T
HREAD
Java provides two mechanisms to create new threads. The simplest mechanism is to
inherit from the
Thread
class. This class contains methods that need to be overridden
in order to make your
Thread
class offer functionality. You need to override the
run()
method and place your execution logic in here. The statements in the
run()
method will execute simultaneously with other statements in your program. The fol-
lowing statements define a class with a
run()
method that will print out a message:
public class MsgThread extends Thread {
public MsgThread () {
super ();
}
public void run () {
for (;;) {
// Create an infinite loop.
System.out.println ("Inside a thread");
}
}
}
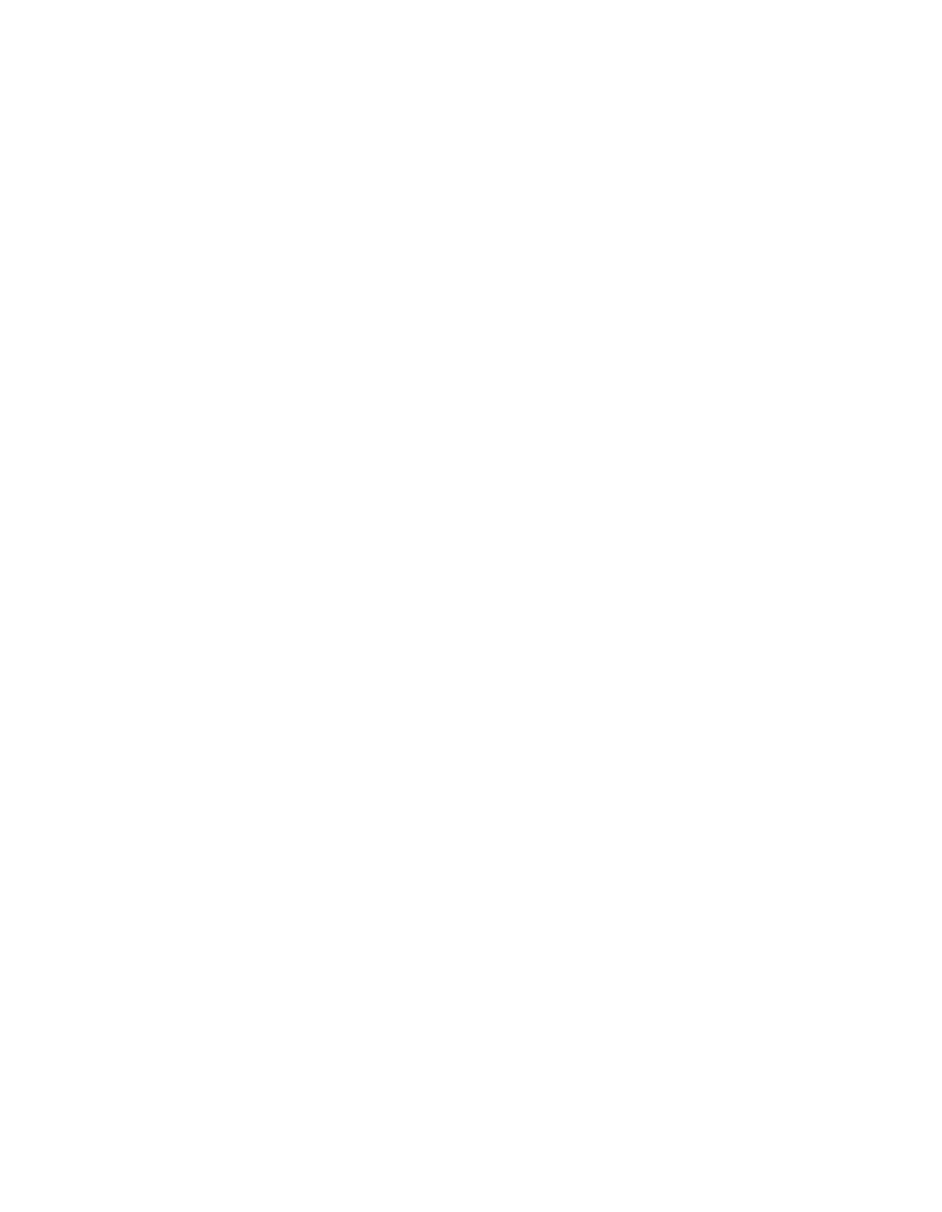