Java Reference
In-Depth Information
break
statement causes the current loop to exit or complete immediately. The
continue
statement causes the current loop to be reiterated from its beginning,
preserving the current values. Consider the following examples:
int x;
int inputMsgSize = 0;
for (x = 0; x < ARRAY_SIZE ; x++) {
inputMsgSize = errorMsgs[x].msgSize;
if (inputMsgSize == 0) {
// Exit the loop immediately. x will point to this element.
break;
}
}
// Evaluate inputMsgSize after the loop.
if (inputMsgSize == 0) {
...
}
The
break
statement exits the current
for
loop at once. Variables modified by
the loop will maintain their current values and may be available after the break
statement is executed, depending on how the variables were defined. In the exam-
ple,
inputMsgSize
will be set to 0 if the break was performed.
In contrast, consider this example:
for (x = 0; x < ARRAY_SIZE ; x++) {
int inputMsgSize = errorMsgs[x].msgSize;
if (inputMsgSize == 0) {
// Continue with the next item in the loop.
continue;
}
// Translate the error message using the getTranslation method.
// Note that the String parameter passed to the setErrorMsg() method is the
// result of the getTranslation() method.
errorMsgs[x].setErrorMsg ((errorMsgs[x].getTranslation()));
}
The
continue
statement causes the loop to continue with the next iteration. In
the example, error messages with sizes equal to 0 will not be translated. But the
loop will continue until all the error messages are examined (and translated, if they
contain text).
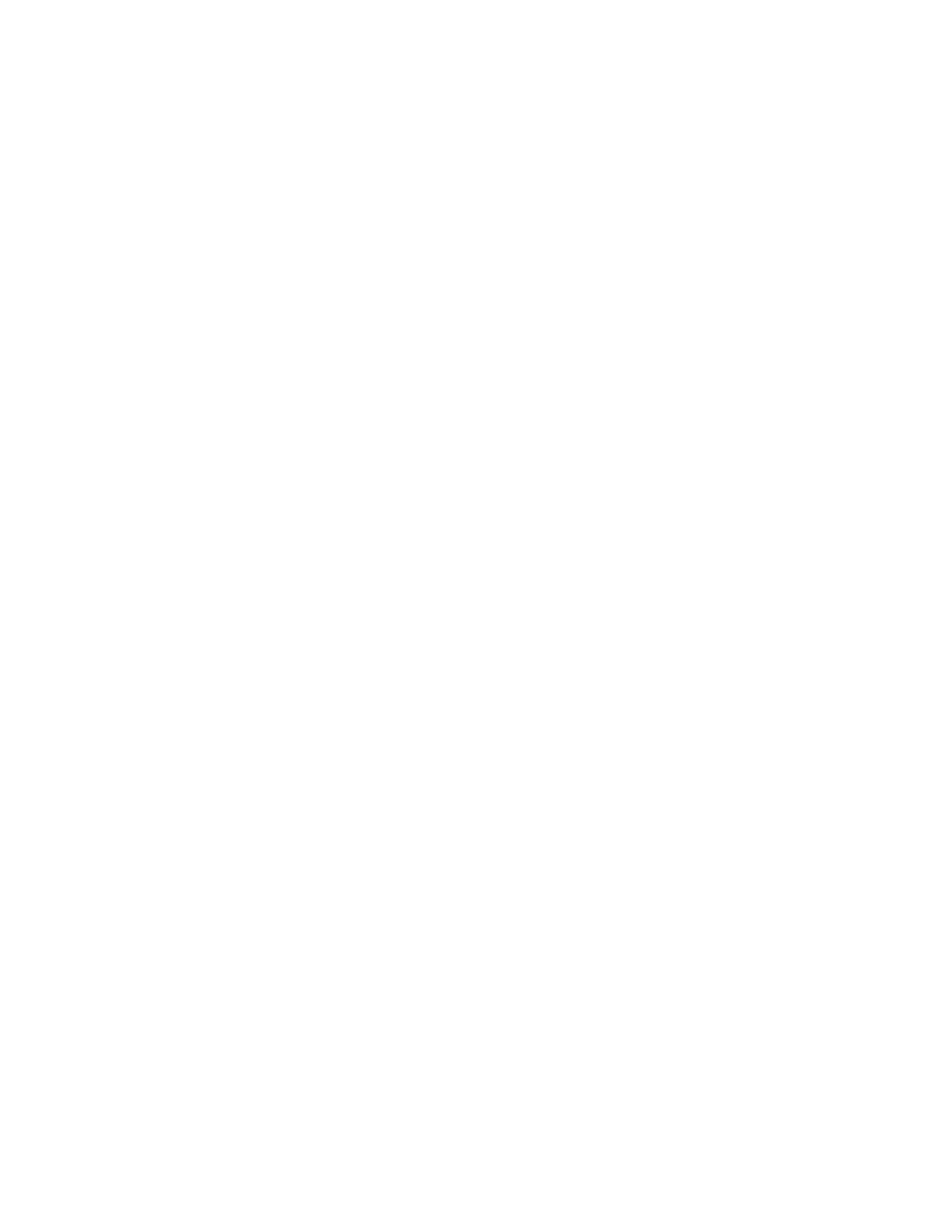