Java Reference
In-Depth Information
will perform the code block if
myErrorMsg
contains either
“Any Text”
or
“Some
Text.”
The following is a syntactically equivalent COBOL statement:
IF (MY-ERROR-MSG = "Any Text") OR
(MY-ERROR-MSG = "Some Text")
... // The IF code block
END-IF.
Java will “short-circuit,” or stop evaluating
condition
, as soon as an unam-
biguous result is available. Therefore, it is possible that some of the expressions in
condition
will not be executed. In the example, if
myErrorMsg
contains
“Any Text”
,
the
equals()
method will be performed only once.
The order of evaluation of expressions can be controlled using parentheses ().
Just as in COBOL, you should liberally use them to clarify your intentions, even if
the compiler does figure it out correctly.
if (myErrorMsg.msgText.equals ("Any Text") &&
myErrorMsg.msgSize == 8 ||
myErrorMsg.msgText.equals ("Some Text") {
// This code block will be performed only when the text equals "Any Text"
// since the length of "Some Text" is 9 (that is, it contains 9
// characters).
...
}
But the intentions of the developer are unclear, or perhaps there is a bug. The
proper code is more likely to be as follows:
if ((myErrorMsg.msgText.equals ("Any Text") &&
myErrorMsg.msgSize == 8) ||
myErrorMsg.msgText.equals ("Some Text")) {
// This code block will be performed for both "Any Text" and "Some Text."
...
}
This is a good time to talk some more about local variable scope. Local variables
are variables that have been defined somewhere in a Java code block:
if (inputMsg.equals ("Any Text")) {
...
// This next statement defines a local variable inputMsgSize and sets it to
// msgSize.
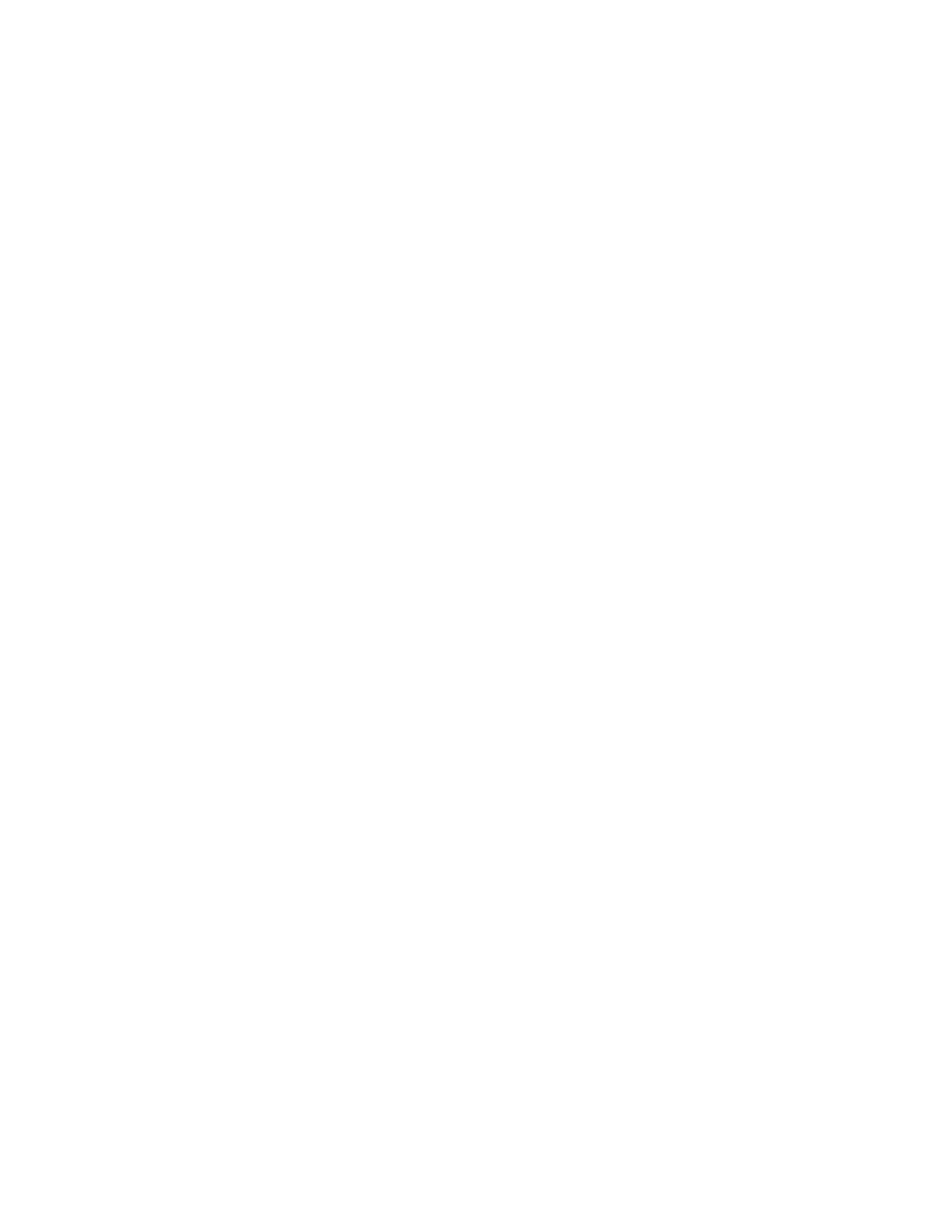