Game Development Reference
In-Depth Information
uniform
f l o a t 4
c o n s t a n t C o l o r ;
/ / We a r e
g o i n g
t o
do
a
t e x t u r e
lookup .
Here we
d e c l a r e
a
” v a r i a b l e ”
/ /
t o
r e f e r
t o
t h i s
t e x t u r e ,
and
a n n o t a t e
i t
w i t h
s u f f i c i e n t
i n f o r m a t i o n
/ /
so
t h a t
our
r e n d e r i n g
code
can
s e l e c t
t h e
a p p r o p r i a t e
t e x t u r e
i n t o
/ /
t h e
r e n d e r i n g
c o n t e x t
b e f o r e
drawing
p r i m i t i v e s .
sampler2D
d i f f u s e M a p ;
/ /
The
body
o f
our
p i x e l
s h a d e r .
I t
o n l y
has
one
o u t p u t ,
which
f a l l s
/ /
under
t h e
s e m a n t i c
”COLOR”
f l o a t 4
main ( I n p u t
i n p u t ) :
COLOR
{
/ /
F e t c h
t h e
t e x e l
f l o a t 4
t e x e l
=
tex2D
( d i f f u s e M a p ,
i n p u t . uv ) ;
/ /
Modulate
i t
by
t h e
c o n s t a n t
c o l o r
and
o u t p u t .
Note
t h a t
/ /
o p e r a t o r
∗
p e r f o r m s
component
−
w i s e
m u l t i p l i c a t i o n .
r e t u r n
t e x e l
∗
c o n s t a n t C o l o r ;
}
Listing 10.12
Pixel shader for decal rendering
Clearly, the higher-level code must supply the shader constants and the
primitive data properly. The simplest way to match up a shader constant
with the higher-level code is to specifically assign a register number to a
constant by using special HLSL variable declaration syntax, but there are
subtler techniques, such as locating constants by name. These practical
details are certainly important, but they are don't belong in this topic.
10.11.2 Basic Per-Pixel Blinn-Phong Lighting
Now let's look at a simple example that actually does some lighting cal-
culations. We start with basic per-pixel lighting, although we don't use a
bump map just yet. This example simply illustrates the Phong shading
technique of interpolating the normal across the face and evaluating the
full lighting equation per-pixel. We compare Phong shading with Gouraud
shading in
Section 10.11.3,
and we show an example of normal mapping in
All of our lighting examples use the standard Blinn-Phong lighting equa-
tion. In this example and most of the examples to follow, the lighting envi-
ronment consists of a single linearly-attenuated omni light plus a constant
ambient.
For the first example (Listings 10.13 and 10.14), we do all of the work
in the pixel shader. In this case, the vertex shader is fairly trivial; it just
needs to pass through the inputs to the pixel shader.
/ /
Mesh
i n p u t s .
s t r u c t
I n p u t
{
f l o a t 4
p o s
:
POSITION
;
/ /
p o s i t i o n
i n
model
space
f l o a t 3
n o r m a l
:
NORMAL
;
/ /
v e r t e x
normal
i n
model
space
f l o a t 2
uv
:
TEXCOORD0
;
/ /
t e x t u r e
c o o r d s
f o r
d i f f u s e ,
spec
maps
}
;
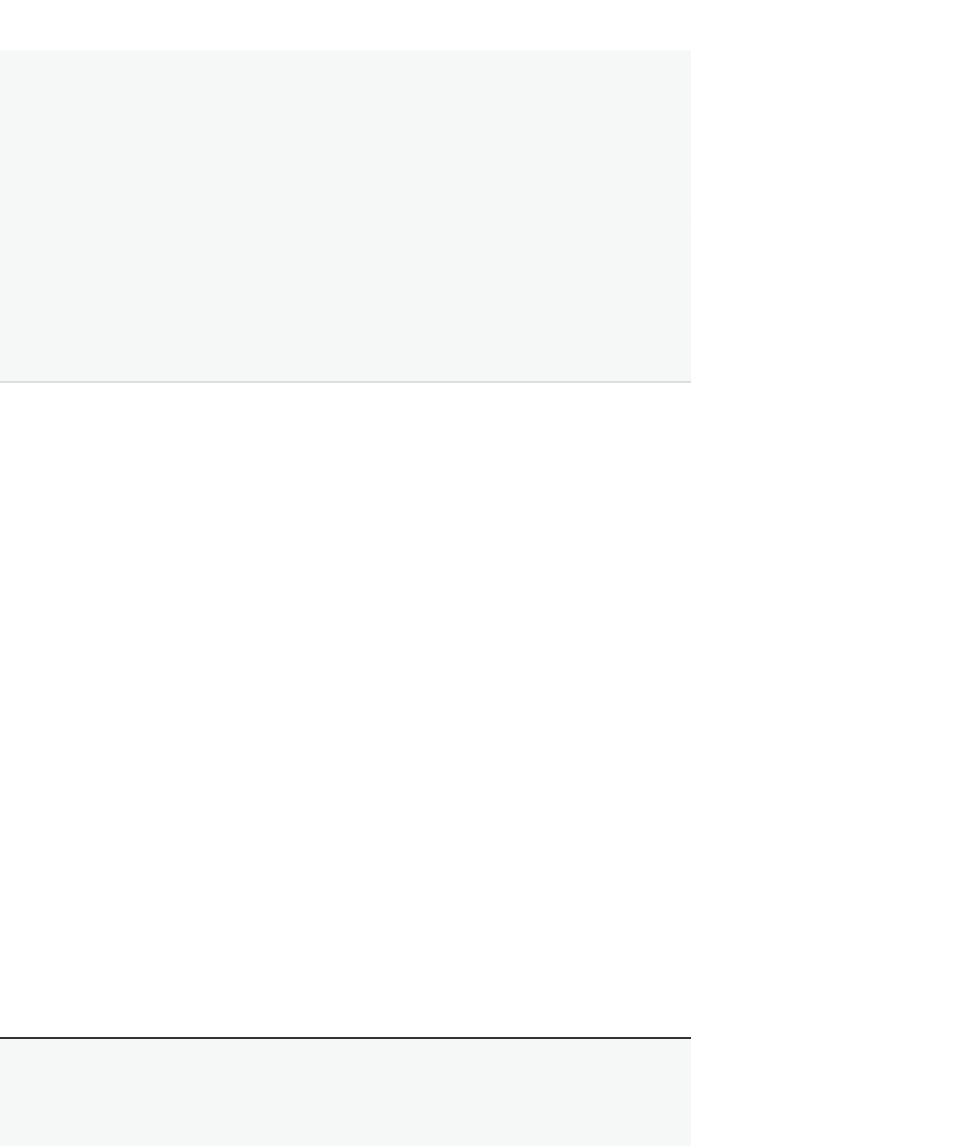






Search WWH ::

Custom Search