Java Reference
In-Depth Information
Loading Entities
Hibernate's
Session
interface provides several
load()
methods for loading entities from your
database. Each
load()
method requires the object's primary key as an identifier.
In addition to the
id
, Hibernate also needs to know which class or entity name to use to
find the object with that
id
. Last, you will need to cast the object returned by
load()
to the class
you desire. The basic
load()
methods are as follows:
public Object load(Class theClass, Serializable id) throws HibernateException
public Object load(String entityName, Serializable id) throws HibernateException
public void load(Object object, Serializable id) throws HibernateException
The last
load()
method takes an object as an argument. The object should be of the same
class as the object you would like loaded, and it should be empty. Hibernate will populate that
object with the object you requested. We find this syntax to be somewhat confusing when put
into applications, so we do not tend to use it ourselves.
The other
load()
methods take a lock mode as an argument. The lock mode specifies
whether Hibernate should look into the cache for the object, and which database lock level
Hibernate should use for the row (or rows) of data that represent this object. The Hibernate
developers claim that Hibernate will usually pick the correct lock mode for you, although we
have seen situations in which it is important to manually choose the correct lock. In addition,
your database may choose its own locking strategy—for instance, locking down an entire table
rather than multiple rows within a table. In order of least restrictive to most restrictive, the
various lock modes you can use are as follows:
•
NONE
: Uses no row-level locking, and uses a cached object if available; this is the
Hibernate default.
•
READ
: Prevents other
SELECT
queries from reading data that is in the middle of a trans-
action (and thus possibly invalid) until it is committed.
•
UPGRADE
: Uses the
SELECT FOR UPDATE
SQL syntax to lock the data until the transaction
is finished.
•
UPGRADE_NOWAIT
: Uses the
NOWAIT
keyword (for Oracle), which returns an error immedi-
ately if there is another thread using that row. Otherwise this is similar to
UPGRADE
.
All of these lock modes are static fields on the
org.hibernate.LockMode
class. We discuss
locking and deadlocks with respect to transactions in more detail in Chapter 8. The
load()
methods that use lock modes are as follows:
public Object load(Class theClass, Serializable id, LockMode lockMode)
throws HibernateException
public Object load(String entityName, Serializable id, LockMode lockMode)
throws HibernateException
You should not use a
load()
method unless you are sure that the object exists. If you are
not certain, then use one of the
get()
methods. The
load()
methods will throw an exception if
the unique
id
is not found in the database, whereas the
get()
methods will merely return a
null reference.
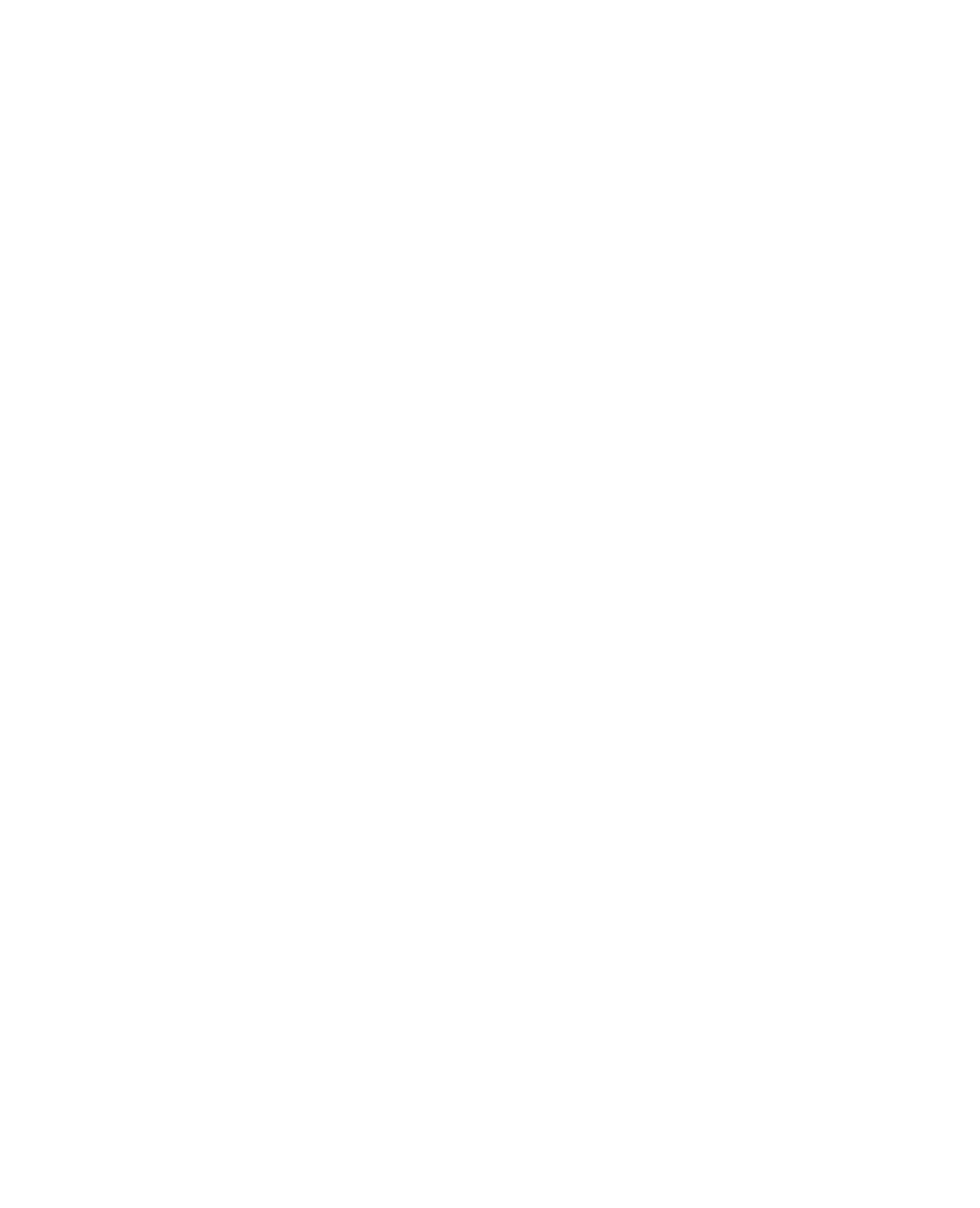
Search WWH ::

Custom Search