Java Reference
In-Depth Information
private static final Logger log = Logger.getAnonymousLogger();
private static final ThreadLocal session = new ThreadLocal();
private static final SessionFactory sessionFactory =
new Configuration().configure().buildSessionFactory();
}
Using the Session
The most common use cases for our POJOs will be to create them and delete them. In both
cases, we want the change to be reflected in the database.
For example, we want to be able to create a user, specifying the username and password,
and have this information stored in the database when we are done.
The logic to create a user (and reflect this in the database) is incredibly simple, as shown
in Listing 3-16.
Listing 3-16.
Creating a
User
Object and Reflecting This in the Database
try {
begin();
User user = new User(username,password);
getSession().save(user);
commit();
return user;
} catch( HibernateException e ) {
rollback();
throw new AdException("Could not create user " + username,e);
}
We begin a transaction, create the new
User
object, ask the session to save the object, and
then commit the transaction. If a problem is encountered (if, for example, a
User
entity with
that username has already been created in the database), then a Hibernate exception will be
thrown, and the entire transaction will be rolled back.
To retrieve the
User
object from the database, we will make our first excursion into HQL.
HQL is somewhat similar to SQL, but you should bear in mind that it refers to the names used
in the mapping files, rather than the table names and columns of the underlying database.
The appropriate HQL query to retrieve the users having a given name field is as follows:
from User where name= :username
where
User
is the class name and
:username
is the HQL named parameter that our code will
populate when we carry out the query. This is remarkably similar to the SQL for a prepared
statement to achieve the same end:
select * from user where name = ?
The complete code to retrieve a user for a specific username is shown in Listing 3-17.
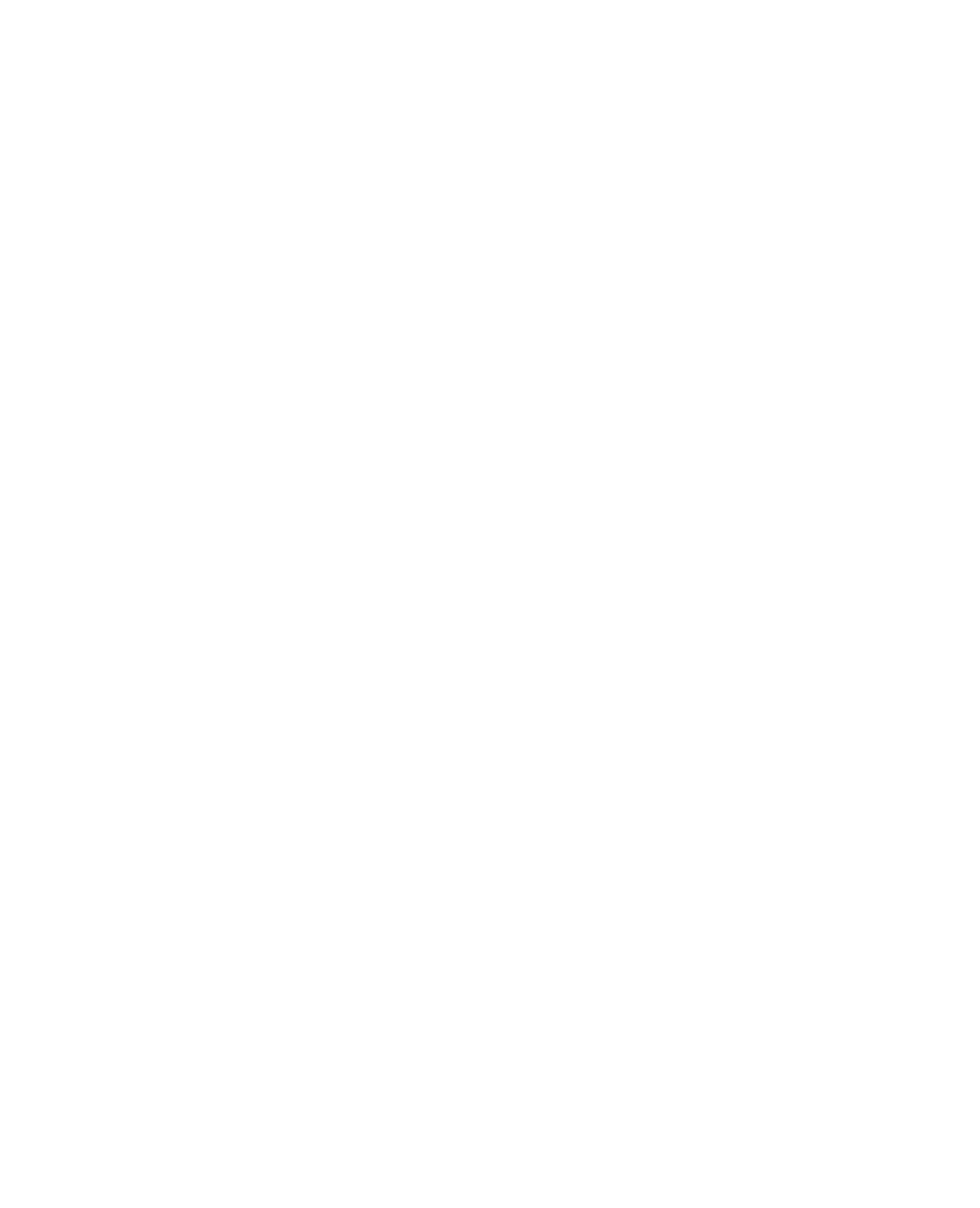
Search WWH ::

Custom Search