Java Reference
In-Depth Information
public interface PaperDao {
public List<Paper> getAll();
public void createPaper(final Paper paper);
public Paper getPaper(final Integer paperId);
public Paper createArticle(final Integer paperId,final Article article);
}
Ideally, you should define an interface to specify the methods that your DAO will con-
tain. Our sample application requires a single DAO with a few simple methods (shown in
Listing C-7).
With the interface clearly specified, your DAO class should then extend the
HibernateDaoSupport
class and implement the interface that you have defined. Extending
HibernateDaoSupport
provides a number of get/set pairs for necessary Spring attributes
(specifically the
sessionFactory
attribute) and various helper methods for implementing
your DAO. A typical DAO method implementation using these methods is shown in
Listing C-8.
Listing C-8.
Implementing
getAll()
Using the Methods from the
HibernateDaoSupport
Class
@SuppressWarnings("unchecked")
public List<Paper> getAll() {
Session session = getSession();
List<Paper> papers = (List<Paper>)session.createQuery("from Paper").list();
releaseSession(session);
return papers;
}
The
getSession()
and
releaseSession()
methods are derived from the DAO class. They
are roughly equivalent to the session factory's
openSession()
method and the session's
close()
method, respectively.
The
HibernateDaoSupport
class also provides access to an appropriately configured helper
object:
HibernateTemplate
. Using this object, the preceding code can be rewritten as shown in
Listing C-9.
Listing C-9
Implementing the
getAll()
Method Using the
HibernateTemplate
Class
@SuppressWarnings("unchecked")
public List<Paper> getAll() {
return (List<Paper>)getHibernateTemplate().find("from Paper");
}
This notably reduces the amount of boilerplate session management code that is
required to process this simply query.
HibernateTemplate
provides a set of methods
(shown in Table C-1) that allow you to carry out most of the basic Hibernate operations
in a similar single line of code.
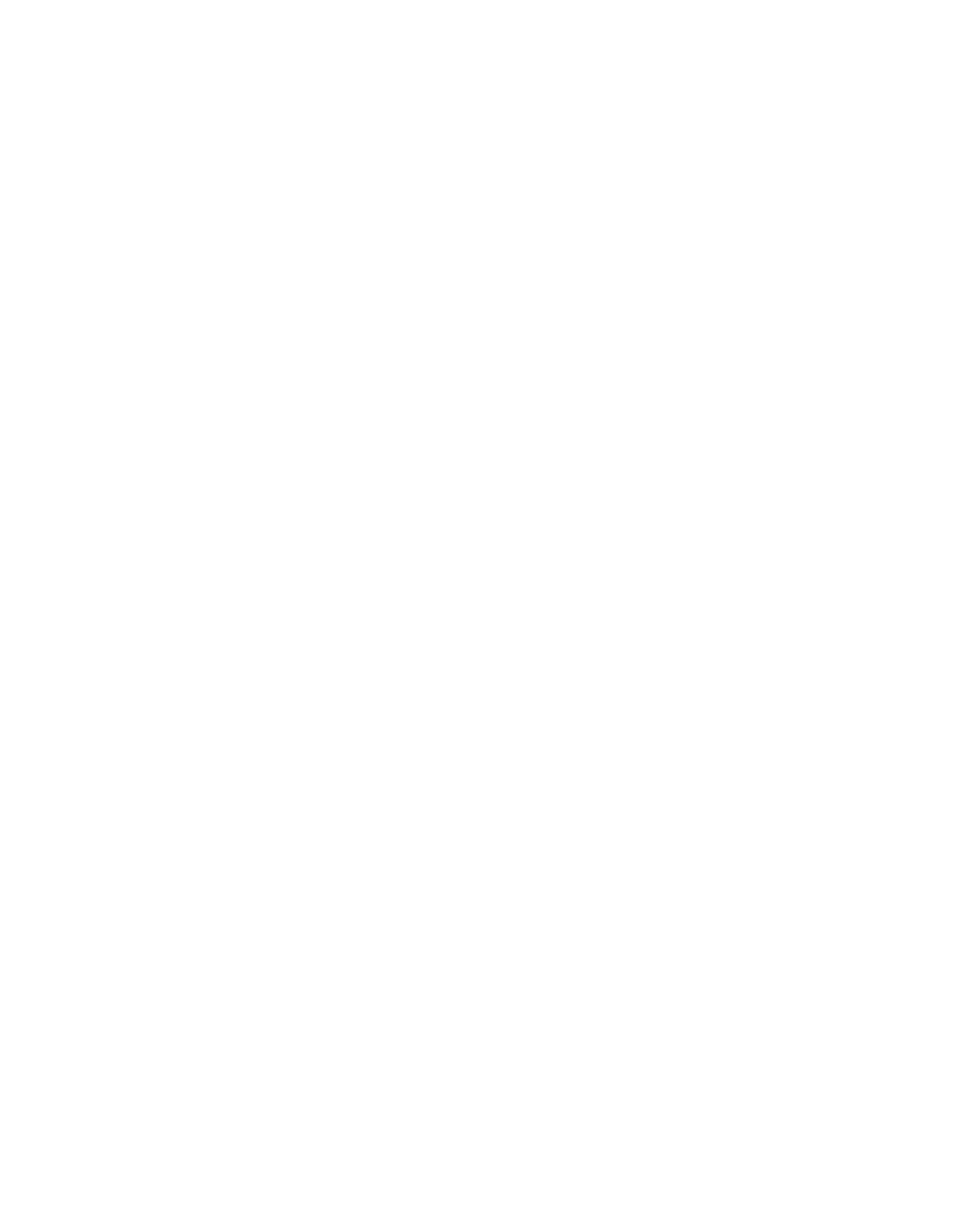
Search WWH ::

Custom Search