Java Reference
In-Depth Information
An Example Event Listener
Before we get stuck in a simple example, a word of caution: events are very much an exposed
part of the inner workings of the
Session
. While this is ideal for something requiring the level
of interference of a security tool, you will not need this for most purposes. Listing A-22 is more
in the nature of an illustrative “hack” than a real solution. In a real application, you would
probably solve this particular problem either within the body of the application, by using
interceptors, or by using triggers.
Listing A-22 shows how an event listener could be used to prevent the booking of cer-
tain seats from being persisted to the database in a concert hall ticket booking application
(we revisit this example application in a little more detail with a slightly more realistic sce-
nario in the later section, “Interceptors”).
Listing A-22.
Programmatically Installing an Event Listener
public class EventExample {
public static void main(String[] args) {
Configuration config = new Configuration();
// Apply this event listener (programmatically)
config.setListener("save-update", new BookingSaveOrUpdateEventListener());
SessionFactory factory = config.configure().buildSessionFactory();
Session session = factory.openSession();
Transaction tx = session.beginTransaction();
// Make our bookings... seat R1 is NOT to be saved.
session.saveOrUpdate(new Booking("charles","R1"));
session.saveOrUpdate(new Booking("camilla","R2"));
// The confirmation letters should not be sent
// out until AFTER the commit completes.
tx.commit();
}
}
Our example is only going to implement the
SaveOrUpdateEventListener
interface. You
will notice that in Listing A-22, the original calls to
save()
have been replaced with calls to
saveOrUpdate()
. There is a close correspondence between the methods on the
Session
interface and the event listeners with similar names. A call to
save()
will not invoke
saveOrUpdate()
, and vice versa. Try using the
save()
method in the
EventExample
, and you
will see that the
BookingSaveOrUpdateListener
is not invoked. In Listing A-23, we present
the logic of the listener registered in Listing A-22.
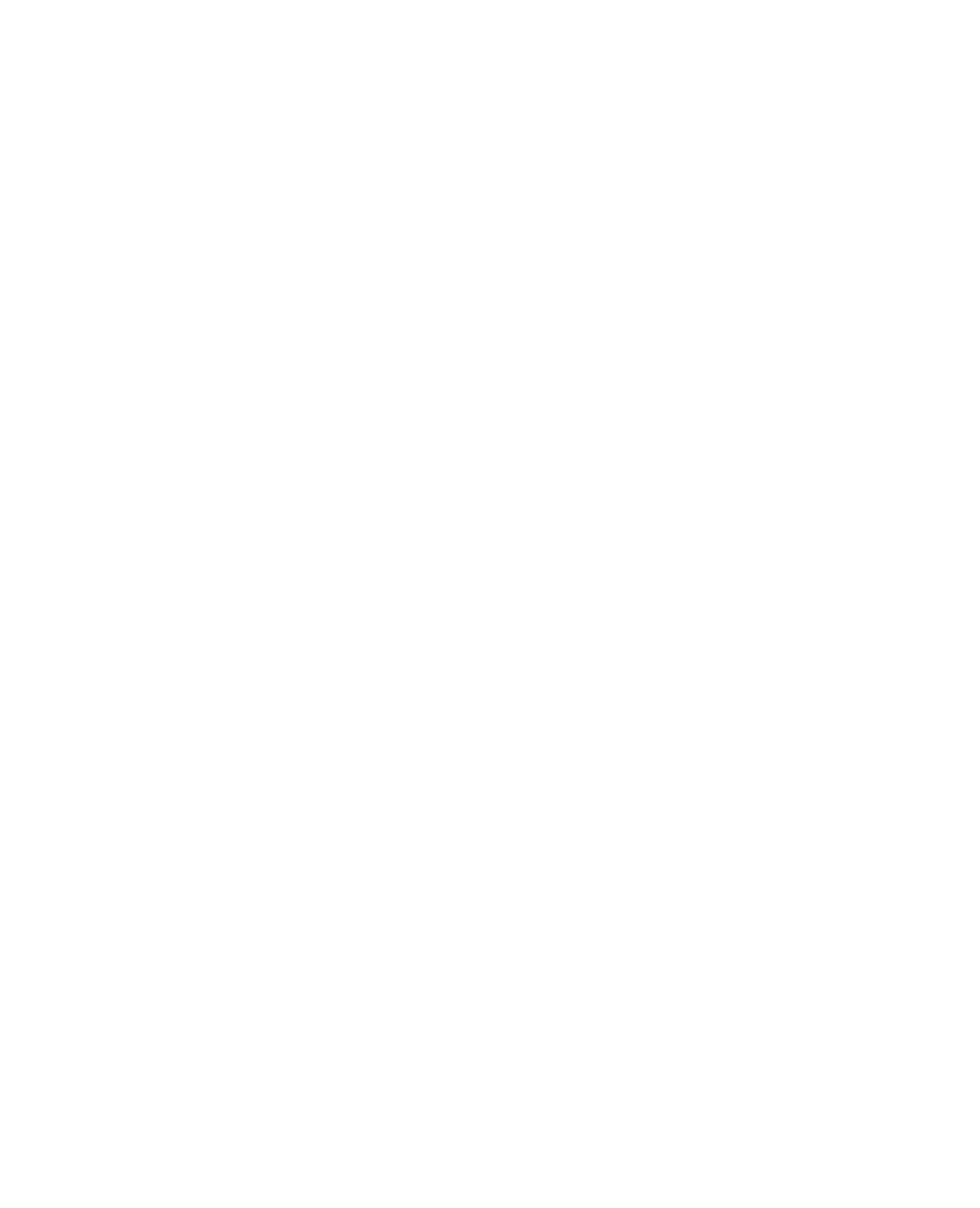
Search WWH ::

Custom Search