Java Reference
In-Depth Information
Criteria crit = session.createCriteria(Product.class);
Criterion price = Restrictions.gt("price",new Double(25.0));
crit.setMaxResults(1);
Product product = (Product) crit.uniqueResult();
Again, we stress that you need to make sure that your query only returns one or zero
results if you use the
uniqueResult()
method. Otherwise, Hibernate will throw a
NonUniqueResultException
exception, which may not be what you would expect—Hibernate
does not just pick the first result and return it.
Sorting the Query's Results
Sorting the query's results works much the same way with criteria as it would with HQL or
SQL. The Criteria API provides the
org.hibernate.criterion.Order
class to sort your result
set in either ascending or descending order, according to one of your object's properties.
Create an
Order
object with either of the two static factory methods on the
Order
class:
asc()
for ascending or
desc()
for descending. Both methods take the name of the property as
their only argument. After you create an
Order
, use the
addOrder()
method on the
Criteria
object to add it to the query.
This example demonstrates how you would use the
Order
class:
Criteria crit = session.createCriteria(Product.class);
crit.add(Restrictions.gt("price",new Double(25.0)));
crit.addOrder(Order.desc("price"));
List results = crit.list();
You may add more than one
Order
object to the
Criteria
object. Hibernate will pass
them through to the underlying SQL query. Your results will be sorted by the first order,
then any identical matches within the first sort will be sorted by the second order, and so
on. Beneath the covers, Hibernate passes this on to an SQL
ORDER BY
clause after substitut-
ing the proper database column name for the property.
Associations
To add a restriction on a class that is associated with your criteria's class, you will need to
create another
Criteria
object. Pass the property name of the associated class to the
createCriteria()
method, and you will have another
Criteria
object. You can get the
results from either
Criteria
object, although you should pick one style and be consistent
for readability's sake. We find that getting the results from the top-level
Criteria
object
(the one that takes a class as a parameter) makes it clear what type of object is expected in
the results.
The association works going from one-to-many as well as from many-to-one. First, we
will demonstrate how to use one-to-many associations to obtain suppliers who sell products
with a price over $25. Notice that we create a new
Criteria
object for the
products
property,
add restrictions to the products' criteria we just created, and then obtain the results from the
supplier
Criteria
object:
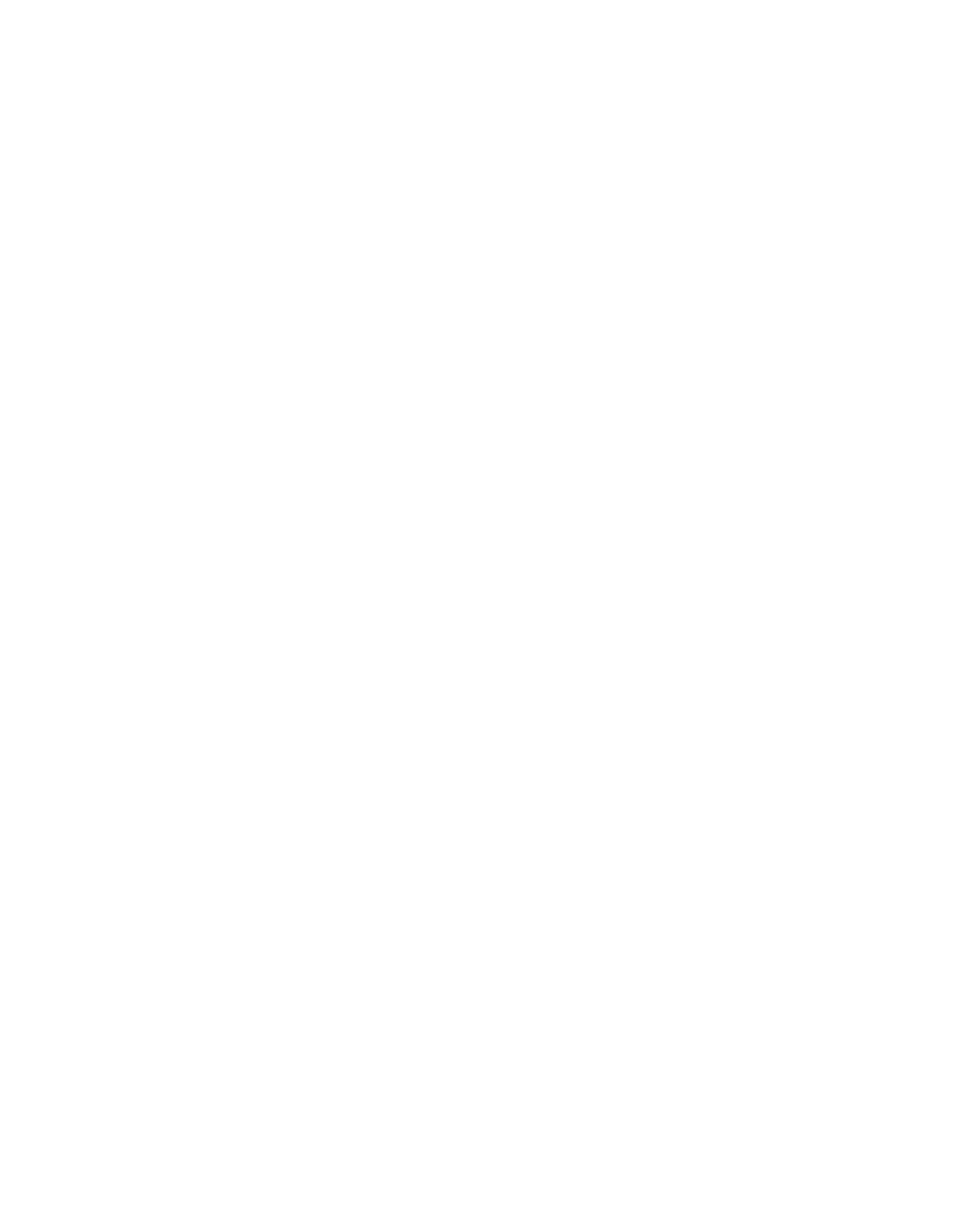
Search WWH ::

Custom Search