Java Reference
In-Depth Information
If you only have one result in your HQL result set, Hibernate has a shortcut method for
obtaining just that object.
Obtaining a Unique Result
HQL's
Query
interface provides a
uniqueResult()
method for obtaining just one object from
an HQL query. Although your query may only yield one object, you may also use the
uniqueResult()
method with other result sets if you limit the results to just the first result.
You could use the
setMaxResults()
method discussed in the previous section. The
uniqueResult()
method on the
Query
object returns a single object, or
null
if there are zero
results. If there is more than one result, the
uniqueResult()
method throws a
NonUniqueResultException
.
The following short example demonstrates having a result set that would have included
more than one result, except that it was limited with the
setMaxResults()
method:
String hql = "from Product where price>25.0";
Query query = session.createQuery(hql);
query.setMaxResults(1);
Product product = (Product) query.uniqueResult();
//test for null here if needed
Unless your query returns one or zero results, the
uniqueResult()
method will throw a
NonUniqueResultException
exception. Do not expect Hibernate just to pick off the first result
and return it—either set the maximum results of the HQL query to
1
, or obtain the first object
from the result list.
Sorting Results with the order by Clause
To sort your HQL query's results, you will need to use the
order by
clause. You can order the
results by any property on the objects in the result set: either ascending (
asc
) or descending
(
desc
). You can use ordering on more than one property in the query if you need to. A typical
HQL query for sorting results looks like this:
from Product p where p.price>25.0 order by p.price desc
If you wanted to sort by more than one property, you would just add the additional prop-
erties to the end of the
order by
clause, separated by commas. For instance, you could sort by
product price and the supplier's name, as follows:
from Product p order by p.supplier.name asc, p.price asc
HQL is more straightforward for ordering than the equivalent approach using the Criteria
Query API.
Associations
Associations allow you to use more than one class in an HQL query, just as SQL allows you to use
joins between tables in a relational database. Add an association to an HQL query with the
join
clause. Hibernate supports five different types of joins:
inner join
,
cross join
,
left outer
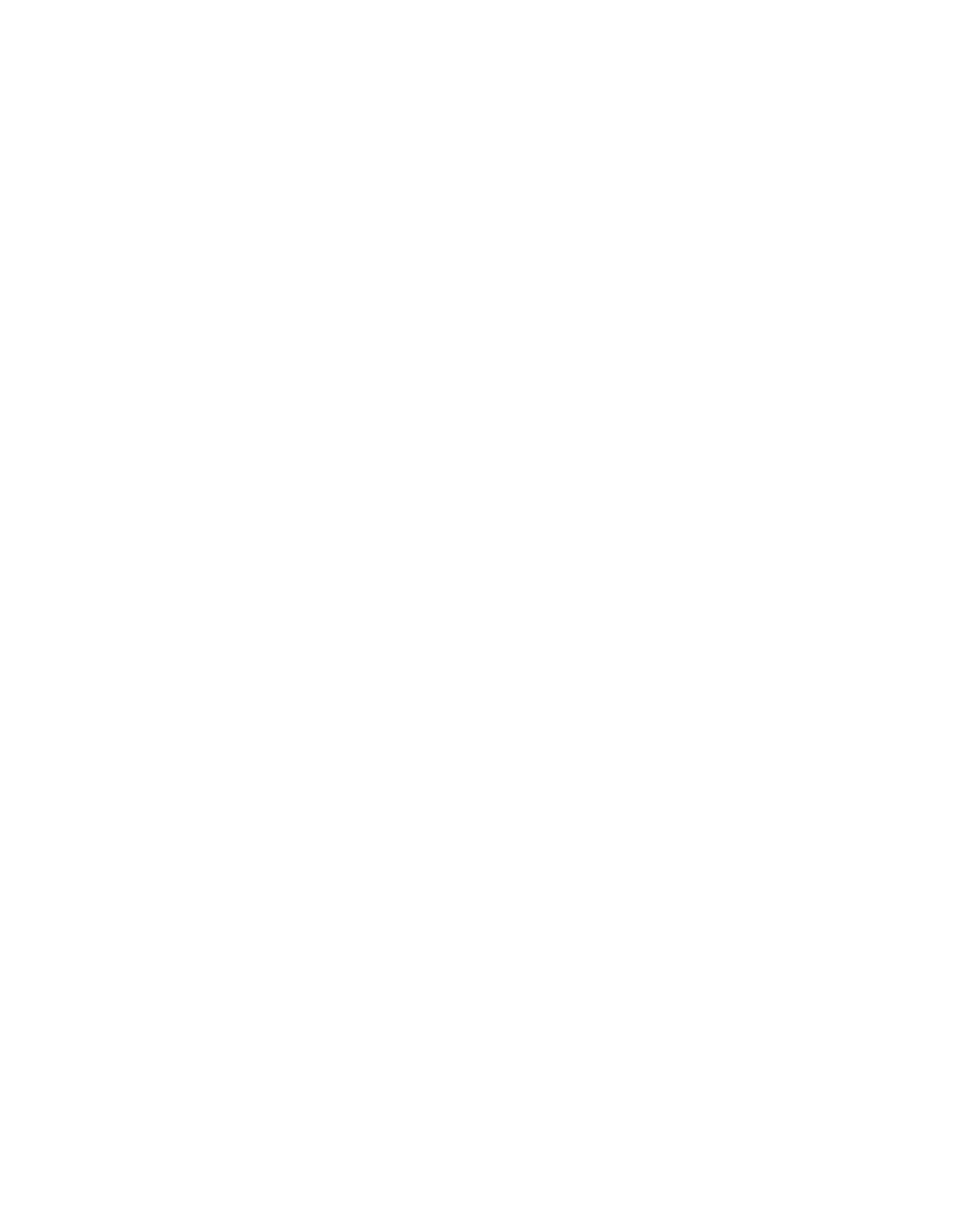
Search WWH ::

Custom Search