Java Reference
In-Depth Information
SessionFactory
objects are expensive objects—needlessly duplicating them will cause
problems quickly, and creating them is a relatively time-consuming process. Ideally, you
should have a single
SessionFactory
for each database your application will access.
SessionFactory
objects are threadsafe, so it is not necessary to obtain one for each thread.
However, you will create numerous
Session
objects—at least one for each thread using Hiber-
nate. Sessions in Hibernate are
not
threadsafe, so sharing
Session
objects between threads
could cause data loss or deadlock. In fact, you will often want to create multiple
Session
instances even during the lifetime of a specific thread (see the “Threads” section for concur-
rency issues).
n
Caution
The analogy between a Hibernate session and a JDBC connection only goes so far. One impor-
tant difference is that if a Hibernate
Session
object throws an exception of any sort, you must discard it and
obtain a new one. This prevents data in the session's cache from becoming inconsistent with the database.
We've already covered the core methods in Chapter 4, so we won't discuss all the methods
available to you through the
Session
interface. For an exhaustive look at what's available, you
should read the API documentation on the Hibernate web site or in the Hibernate 3 down-
load. Table 8-1 gives an overview of the various categories of methods available to you.
Table 8-1.
Hibernate Method Summary
Method Description
Create, Read, Update, and Delete
save()
Saves an object to the database. This should not be called for an object
that has already been saved to the database.
saveOrUpdate()
Saves an object to the database, or updates the database if the object
already exists. This method is slightly less efficient than the
save()
method
since it may need to perform a
SELECT
statement to check whether the
object already exists, but it will not fail if the object has already been saved.
merge()
Merges the fields of a nonpersistent object into the appropriate persistent
object (determined by ID). If no such object exists in the database, then
one is created and saved.
persist()
Reassociates an object with the session so that changes made to the object
will be persisted.
get()
Retrieves a specific object from the database by the object's identifier.
getEntityName()
Retrieves the entity name (this will usually be the same as the class name
of the POJO).
getIdentifier()
Determines the identifier—the object(s) representing the primary key—for
a specific object associated with the session.
load()
Loads an object from the database by the object's identifier (you should use
the
get()
methods if you are not certain that the object is in the database).
refresh()
Refreshes the state of an associated object from the database.
update()
Updates the database with changes to an object.
delete()
Deletes an object from the database.
createFilter()
Creates a filter (query) to narrow operations on the database.
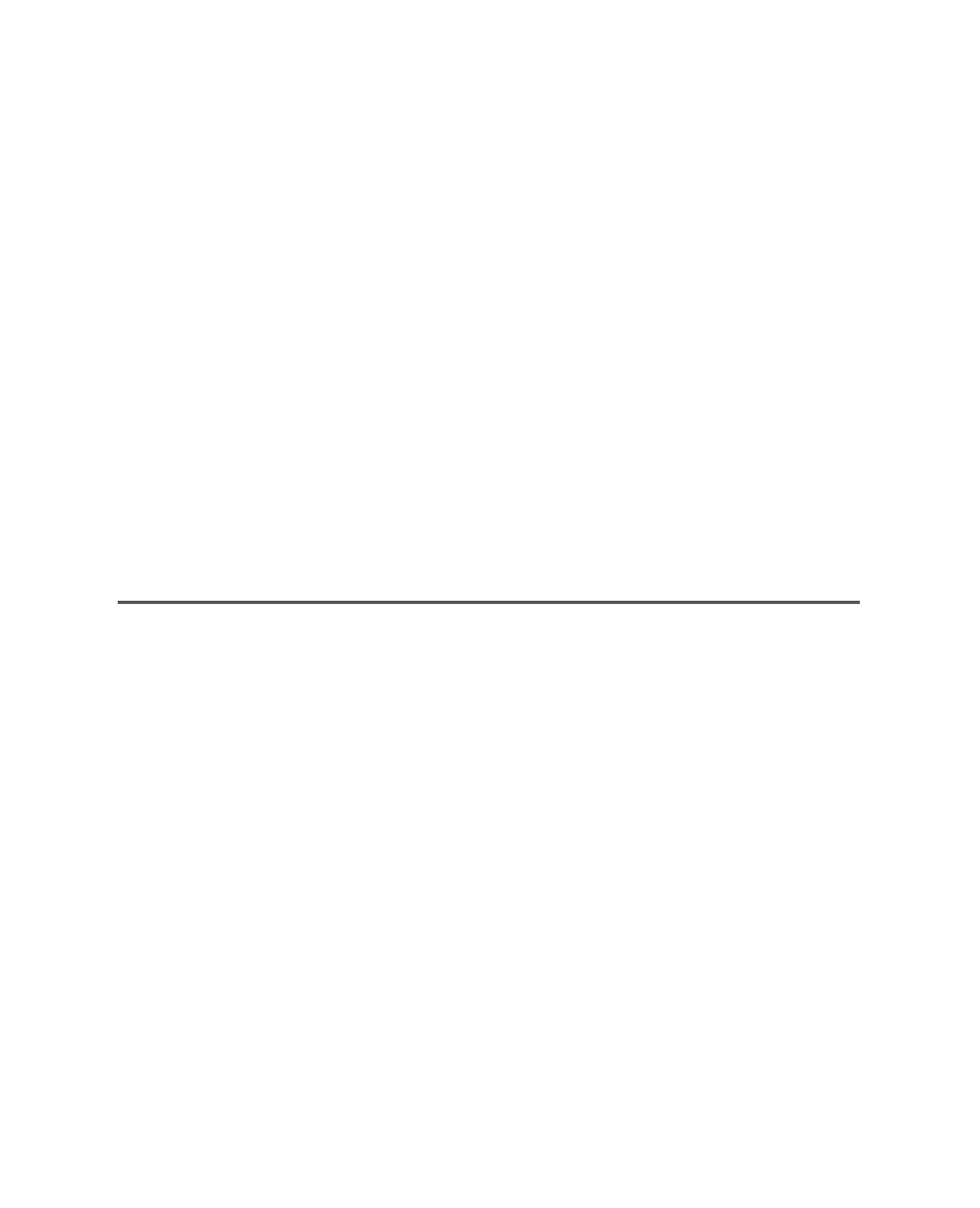




Search WWH ::

Custom Search