Java Reference
In-Depth Information
The following program, named CatchDBZ, intercepts the Java
ArtihmeticException error response in the case of a division by zero.
Note that because ArithmeticException is unchecked it does not have to
be advertised in the header of the main() method.
On the Web
The source file for the program CatchDBZ.java can be found in the
Chapter 19 folder at www.crcpress.com.
//**********************************************************
//**********************************************************
// Program: CatchDBZ
// Reference: Chapter 19
// Topics:
// 1. Catching Java's ArithmeticException error in a
// division by zero
// Requires:
// 1. Keyin class in the current directory
//**********************************************************
//**********************************************************
public class CatchDBZ
{
public static void main(String[] args)
{
int dividend = 100;
int divisor, result;
while(true)
{
divisor = Keyin.inInt("Enter divisor (100 to end): ");
if(divisor == 100)
break;
try
{
result = dividend / divisor; // May raise exception
System.out.println("result="+result);
}
catch(ArithmeticException msgText)
{
System.out.println("Error is:"+msgText);
}
}
}
}
The CatchDBZ.java program, listed previously, allows the user to enter
the divisor of an integer division operation. A special value of 100 is used
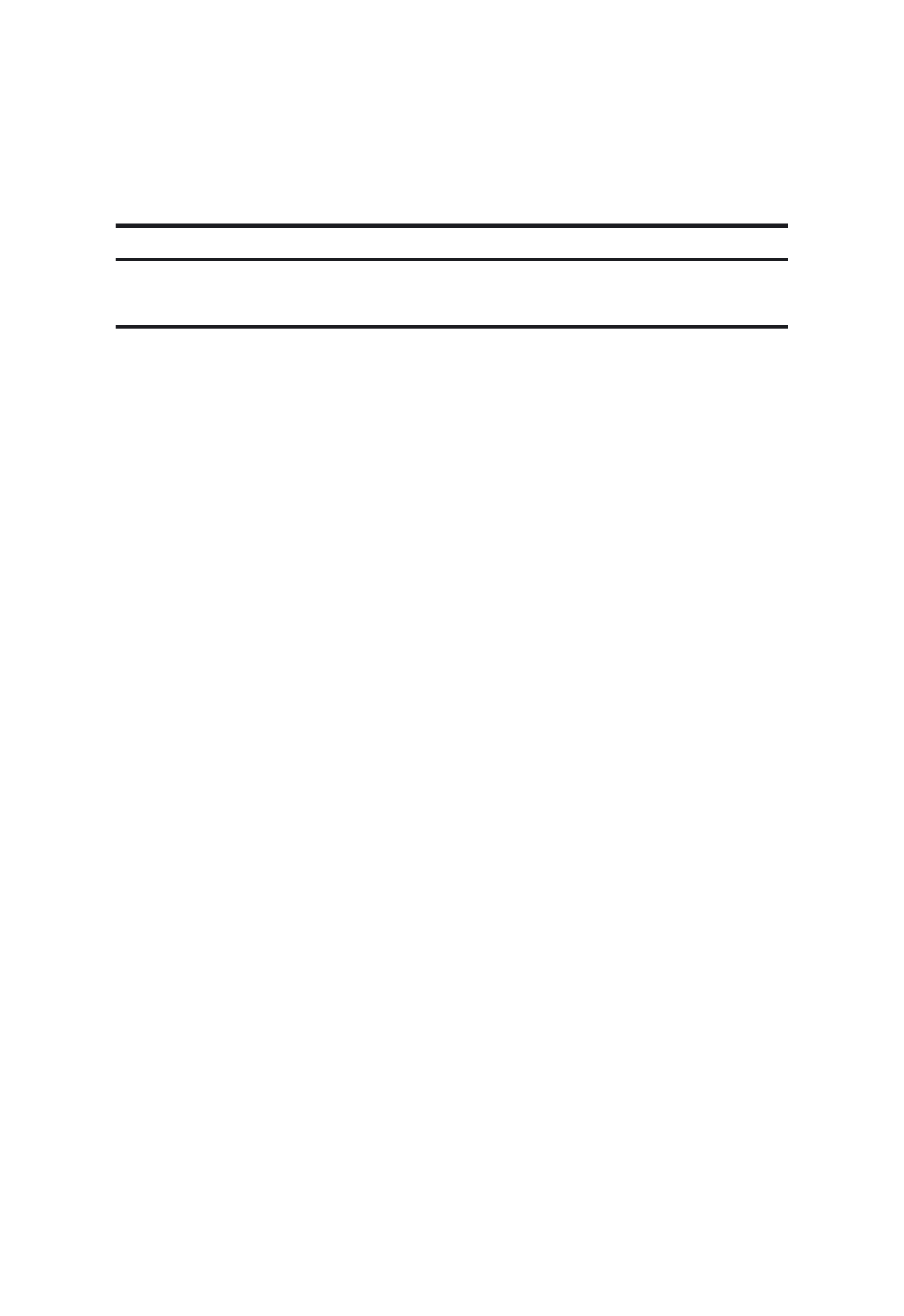






